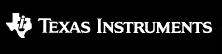 |
 |
Go to the documentation of this file. 33 #ifndef __HW_CPU_SCS_H__ 34 #define __HW_CPU_SCS_H__ 43 #define CPU_SCS_O_ICTR 0x00000004 46 #define CPU_SCS_O_ACTLR 0x00000008 49 #define CPU_SCS_O_STCSR 0x00000010 52 #define CPU_SCS_O_STRVR 0x00000014 55 #define CPU_SCS_O_STCVR 0x00000018 58 #define CPU_SCS_O_STCR 0x0000001C 61 #define CPU_SCS_O_NVIC_ISER0 0x00000100 64 #define CPU_SCS_O_NVIC_ISER1 0x00000104 67 #define CPU_SCS_O_NVIC_ICER0 0x00000180 70 #define CPU_SCS_O_NVIC_ICER1 0x00000184 73 #define CPU_SCS_O_NVIC_ISPR0 0x00000200 76 #define CPU_SCS_O_NVIC_ISPR1 0x00000204 79 #define CPU_SCS_O_NVIC_ICPR0 0x00000280 82 #define CPU_SCS_O_NVIC_ICPR1 0x00000284 85 #define CPU_SCS_O_NVIC_IABR0 0x00000300 88 #define CPU_SCS_O_NVIC_IABR1 0x00000304 91 #define CPU_SCS_O_NVIC_IPR0 0x00000400 94 #define CPU_SCS_O_NVIC_IPR1 0x00000404 97 #define CPU_SCS_O_NVIC_IPR2 0x00000408 100 #define CPU_SCS_O_NVIC_IPR3 0x0000040C 103 #define CPU_SCS_O_NVIC_IPR4 0x00000410 106 #define CPU_SCS_O_NVIC_IPR5 0x00000414 109 #define CPU_SCS_O_NVIC_IPR6 0x00000418 112 #define CPU_SCS_O_NVIC_IPR7 0x0000041C 115 #define CPU_SCS_O_NVIC_IPR8 0x00000420 118 #define CPU_SCS_O_NVIC_IPR9 0x00000424 121 #define CPU_SCS_O_CPUID 0x00000D00 124 #define CPU_SCS_O_ICSR 0x00000D04 127 #define CPU_SCS_O_VTOR 0x00000D08 130 #define CPU_SCS_O_AIRCR 0x00000D0C 133 #define CPU_SCS_O_SCR 0x00000D10 136 #define CPU_SCS_O_CCR 0x00000D14 139 #define CPU_SCS_O_SHPR1 0x00000D18 142 #define CPU_SCS_O_SHPR2 0x00000D1C 145 #define CPU_SCS_O_SHPR3 0x00000D20 148 #define CPU_SCS_O_SHCSR 0x00000D24 151 #define CPU_SCS_O_CFSR 0x00000D28 154 #define CPU_SCS_O_HFSR 0x00000D2C 157 #define CPU_SCS_O_DFSR 0x00000D30 160 #define CPU_SCS_O_MMFAR 0x00000D34 163 #define CPU_SCS_O_BFAR 0x00000D38 166 #define CPU_SCS_O_AFSR 0x00000D3C 169 #define CPU_SCS_O_ID_PFR0 0x00000D40 172 #define CPU_SCS_O_ID_PFR1 0x00000D44 175 #define CPU_SCS_O_ID_DFR0 0x00000D48 178 #define CPU_SCS_O_ID_AFR0 0x00000D4C 181 #define CPU_SCS_O_ID_MMFR0 0x00000D50 184 #define CPU_SCS_O_ID_MMFR1 0x00000D54 187 #define CPU_SCS_O_ID_MMFR2 0x00000D58 190 #define CPU_SCS_O_ID_MMFR3 0x00000D5C 193 #define CPU_SCS_O_ID_ISAR0 0x00000D60 196 #define CPU_SCS_O_ID_ISAR1 0x00000D64 199 #define CPU_SCS_O_ID_ISAR2 0x00000D68 202 #define CPU_SCS_O_ID_ISAR3 0x00000D6C 205 #define CPU_SCS_O_ID_ISAR4 0x00000D70 208 #define CPU_SCS_O_CPACR 0x00000D88 211 #define CPU_SCS_O_MPU_TYPE 0x00000D90 214 #define CPU_SCS_O_MPU_CTRL 0x00000D94 217 #define CPU_SCS_O_MPU_RNR 0x00000D98 220 #define CPU_SCS_O_MPU_RBAR 0x00000D9C 223 #define CPU_SCS_O_MPU_RASR 0x00000DA0 226 #define CPU_SCS_O_MPU_RBAR_A1 0x00000DA4 229 #define CPU_SCS_O_MPU_RASR_A1 0x00000DA8 232 #define CPU_SCS_O_MPU_RBAR_A2 0x00000DAC 235 #define CPU_SCS_O_MPU_RASR_A2 0x00000DB0 238 #define CPU_SCS_O_MPU_RBAR_A3 0x00000DB4 241 #define CPU_SCS_O_MPU_RASR_A3 0x00000DB8 244 #define CPU_SCS_O_DHCSR 0x00000DF0 247 #define CPU_SCS_O_DCRSR 0x00000DF4 250 #define CPU_SCS_O_DCRDR 0x00000DF8 253 #define CPU_SCS_O_DEMCR 0x00000DFC 256 #define CPU_SCS_O_STIR 0x00000F00 259 #define CPU_SCS_O_FPCCR 0x00000F34 262 #define CPU_SCS_O_FPCAR 0x00000F38 265 #define CPU_SCS_O_FPDSCR 0x00000F3C 268 #define CPU_SCS_O_MVFR0 0x00000F40 271 #define CPU_SCS_O_MVFR1 0x00000F44 290 #define CPU_SCS_ICTR_INTLINESNUM_W 3 291 #define CPU_SCS_ICTR_INTLINESNUM_M 0x00000007 292 #define CPU_SCS_ICTR_INTLINESNUM_S 0 303 #define CPU_SCS_ACTLR_DISOOFP 0x00000200 304 #define CPU_SCS_ACTLR_DISOOFP_BITN 9 305 #define CPU_SCS_ACTLR_DISOOFP_M 0x00000200 306 #define CPU_SCS_ACTLR_DISOOFP_S 9 311 #define CPU_SCS_ACTLR_DISFPCA 0x00000100 312 #define CPU_SCS_ACTLR_DISFPCA_BITN 8 313 #define CPU_SCS_ACTLR_DISFPCA_M 0x00000100 314 #define CPU_SCS_ACTLR_DISFPCA_S 8 319 #define CPU_SCS_ACTLR_DISFOLD 0x00000004 320 #define CPU_SCS_ACTLR_DISFOLD_BITN 2 321 #define CPU_SCS_ACTLR_DISFOLD_M 0x00000004 322 #define CPU_SCS_ACTLR_DISFOLD_S 2 330 #define CPU_SCS_ACTLR_DISDEFWBUF 0x00000002 331 #define CPU_SCS_ACTLR_DISDEFWBUF_BITN 1 332 #define CPU_SCS_ACTLR_DISDEFWBUF_M 0x00000002 333 #define CPU_SCS_ACTLR_DISDEFWBUF_S 1 340 #define CPU_SCS_ACTLR_DISMCYCINT 0x00000001 341 #define CPU_SCS_ACTLR_DISMCYCINT_BITN 0 342 #define CPU_SCS_ACTLR_DISMCYCINT_M 0x00000001 343 #define CPU_SCS_ACTLR_DISMCYCINT_S 0 357 #define CPU_SCS_STCSR_COUNTFLAG 0x00010000 358 #define CPU_SCS_STCSR_COUNTFLAG_BITN 16 359 #define CPU_SCS_STCSR_COUNTFLAG_M 0x00010000 360 #define CPU_SCS_STCSR_COUNTFLAG_S 16 371 #define CPU_SCS_STCSR_CLKSOURCE 0x00000004 372 #define CPU_SCS_STCSR_CLKSOURCE_BITN 2 373 #define CPU_SCS_STCSR_CLKSOURCE_M 0x00000004 374 #define CPU_SCS_STCSR_CLKSOURCE_S 2 381 #define CPU_SCS_STCSR_TICKINT 0x00000002 382 #define CPU_SCS_STCSR_TICKINT_BITN 1 383 #define CPU_SCS_STCSR_TICKINT_M 0x00000002 384 #define CPU_SCS_STCSR_TICKINT_S 1 395 #define CPU_SCS_STCSR_ENABLE 0x00000001 396 #define CPU_SCS_STCSR_ENABLE_BITN 0 397 #define CPU_SCS_STCSR_ENABLE_M 0x00000001 398 #define CPU_SCS_STCSR_ENABLE_S 0 409 #define CPU_SCS_STRVR_RELOAD_W 24 410 #define CPU_SCS_STRVR_RELOAD_M 0x00FFFFFF 411 #define CPU_SCS_STRVR_RELOAD_S 0 424 #define CPU_SCS_STCVR_CURRENT_W 24 425 #define CPU_SCS_STCVR_CURRENT_M 0x00FFFFFF 426 #define CPU_SCS_STCVR_CURRENT_S 0 436 #define CPU_SCS_STCR_NOREF 0x80000000 437 #define CPU_SCS_STCR_NOREF_BITN 31 438 #define CPU_SCS_STCR_NOREF_M 0x80000000 439 #define CPU_SCS_STCR_NOREF_S 31 445 #define CPU_SCS_STCR_SKEW 0x40000000 446 #define CPU_SCS_STCR_SKEW_BITN 30 447 #define CPU_SCS_STCR_SKEW_M 0x40000000 448 #define CPU_SCS_STCR_SKEW_S 30 455 #define CPU_SCS_STCR_TENMS_W 24 456 #define CPU_SCS_STCR_TENMS_M 0x00FFFFFF 457 #define CPU_SCS_STCR_TENMS_S 0 469 #define CPU_SCS_NVIC_ISER0_SETENA31 0x80000000 470 #define CPU_SCS_NVIC_ISER0_SETENA31_BITN 31 471 #define CPU_SCS_NVIC_ISER0_SETENA31_M 0x80000000 472 #define CPU_SCS_NVIC_ISER0_SETENA31_S 31 479 #define CPU_SCS_NVIC_ISER0_SETENA30 0x40000000 480 #define CPU_SCS_NVIC_ISER0_SETENA30_BITN 30 481 #define CPU_SCS_NVIC_ISER0_SETENA30_M 0x40000000 482 #define CPU_SCS_NVIC_ISER0_SETENA30_S 30 489 #define CPU_SCS_NVIC_ISER0_SETENA29 0x20000000 490 #define CPU_SCS_NVIC_ISER0_SETENA29_BITN 29 491 #define CPU_SCS_NVIC_ISER0_SETENA29_M 0x20000000 492 #define CPU_SCS_NVIC_ISER0_SETENA29_S 29 499 #define CPU_SCS_NVIC_ISER0_SETENA28 0x10000000 500 #define CPU_SCS_NVIC_ISER0_SETENA28_BITN 28 501 #define CPU_SCS_NVIC_ISER0_SETENA28_M 0x10000000 502 #define CPU_SCS_NVIC_ISER0_SETENA28_S 28 509 #define CPU_SCS_NVIC_ISER0_SETENA27 0x08000000 510 #define CPU_SCS_NVIC_ISER0_SETENA27_BITN 27 511 #define CPU_SCS_NVIC_ISER0_SETENA27_M 0x08000000 512 #define CPU_SCS_NVIC_ISER0_SETENA27_S 27 519 #define CPU_SCS_NVIC_ISER0_SETENA26 0x04000000 520 #define CPU_SCS_NVIC_ISER0_SETENA26_BITN 26 521 #define CPU_SCS_NVIC_ISER0_SETENA26_M 0x04000000 522 #define CPU_SCS_NVIC_ISER0_SETENA26_S 26 529 #define CPU_SCS_NVIC_ISER0_SETENA25 0x02000000 530 #define CPU_SCS_NVIC_ISER0_SETENA25_BITN 25 531 #define CPU_SCS_NVIC_ISER0_SETENA25_M 0x02000000 532 #define CPU_SCS_NVIC_ISER0_SETENA25_S 25 539 #define CPU_SCS_NVIC_ISER0_SETENA24 0x01000000 540 #define CPU_SCS_NVIC_ISER0_SETENA24_BITN 24 541 #define CPU_SCS_NVIC_ISER0_SETENA24_M 0x01000000 542 #define CPU_SCS_NVIC_ISER0_SETENA24_S 24 549 #define CPU_SCS_NVIC_ISER0_SETENA23 0x00800000 550 #define CPU_SCS_NVIC_ISER0_SETENA23_BITN 23 551 #define CPU_SCS_NVIC_ISER0_SETENA23_M 0x00800000 552 #define CPU_SCS_NVIC_ISER0_SETENA23_S 23 559 #define CPU_SCS_NVIC_ISER0_SETENA22 0x00400000 560 #define CPU_SCS_NVIC_ISER0_SETENA22_BITN 22 561 #define CPU_SCS_NVIC_ISER0_SETENA22_M 0x00400000 562 #define CPU_SCS_NVIC_ISER0_SETENA22_S 22 569 #define CPU_SCS_NVIC_ISER0_SETENA21 0x00200000 570 #define CPU_SCS_NVIC_ISER0_SETENA21_BITN 21 571 #define CPU_SCS_NVIC_ISER0_SETENA21_M 0x00200000 572 #define CPU_SCS_NVIC_ISER0_SETENA21_S 21 579 #define CPU_SCS_NVIC_ISER0_SETENA20 0x00100000 580 #define CPU_SCS_NVIC_ISER0_SETENA20_BITN 20 581 #define CPU_SCS_NVIC_ISER0_SETENA20_M 0x00100000 582 #define CPU_SCS_NVIC_ISER0_SETENA20_S 20 589 #define CPU_SCS_NVIC_ISER0_SETENA19 0x00080000 590 #define CPU_SCS_NVIC_ISER0_SETENA19_BITN 19 591 #define CPU_SCS_NVIC_ISER0_SETENA19_M 0x00080000 592 #define CPU_SCS_NVIC_ISER0_SETENA19_S 19 599 #define CPU_SCS_NVIC_ISER0_SETENA18 0x00040000 600 #define CPU_SCS_NVIC_ISER0_SETENA18_BITN 18 601 #define CPU_SCS_NVIC_ISER0_SETENA18_M 0x00040000 602 #define CPU_SCS_NVIC_ISER0_SETENA18_S 18 609 #define CPU_SCS_NVIC_ISER0_SETENA17 0x00020000 610 #define CPU_SCS_NVIC_ISER0_SETENA17_BITN 17 611 #define CPU_SCS_NVIC_ISER0_SETENA17_M 0x00020000 612 #define CPU_SCS_NVIC_ISER0_SETENA17_S 17 619 #define CPU_SCS_NVIC_ISER0_SETENA16 0x00010000 620 #define CPU_SCS_NVIC_ISER0_SETENA16_BITN 16 621 #define CPU_SCS_NVIC_ISER0_SETENA16_M 0x00010000 622 #define CPU_SCS_NVIC_ISER0_SETENA16_S 16 629 #define CPU_SCS_NVIC_ISER0_SETENA15 0x00008000 630 #define CPU_SCS_NVIC_ISER0_SETENA15_BITN 15 631 #define CPU_SCS_NVIC_ISER0_SETENA15_M 0x00008000 632 #define CPU_SCS_NVIC_ISER0_SETENA15_S 15 639 #define CPU_SCS_NVIC_ISER0_SETENA14 0x00004000 640 #define CPU_SCS_NVIC_ISER0_SETENA14_BITN 14 641 #define CPU_SCS_NVIC_ISER0_SETENA14_M 0x00004000 642 #define CPU_SCS_NVIC_ISER0_SETENA14_S 14 649 #define CPU_SCS_NVIC_ISER0_SETENA13 0x00002000 650 #define CPU_SCS_NVIC_ISER0_SETENA13_BITN 13 651 #define CPU_SCS_NVIC_ISER0_SETENA13_M 0x00002000 652 #define CPU_SCS_NVIC_ISER0_SETENA13_S 13 659 #define CPU_SCS_NVIC_ISER0_SETENA12 0x00001000 660 #define CPU_SCS_NVIC_ISER0_SETENA12_BITN 12 661 #define CPU_SCS_NVIC_ISER0_SETENA12_M 0x00001000 662 #define CPU_SCS_NVIC_ISER0_SETENA12_S 12 669 #define CPU_SCS_NVIC_ISER0_SETENA11 0x00000800 670 #define CPU_SCS_NVIC_ISER0_SETENA11_BITN 11 671 #define CPU_SCS_NVIC_ISER0_SETENA11_M 0x00000800 672 #define CPU_SCS_NVIC_ISER0_SETENA11_S 11 679 #define CPU_SCS_NVIC_ISER0_SETENA10 0x00000400 680 #define CPU_SCS_NVIC_ISER0_SETENA10_BITN 10 681 #define CPU_SCS_NVIC_ISER0_SETENA10_M 0x00000400 682 #define CPU_SCS_NVIC_ISER0_SETENA10_S 10 689 #define CPU_SCS_NVIC_ISER0_SETENA9 0x00000200 690 #define CPU_SCS_NVIC_ISER0_SETENA9_BITN 9 691 #define CPU_SCS_NVIC_ISER0_SETENA9_M 0x00000200 692 #define CPU_SCS_NVIC_ISER0_SETENA9_S 9 699 #define CPU_SCS_NVIC_ISER0_SETENA8 0x00000100 700 #define CPU_SCS_NVIC_ISER0_SETENA8_BITN 8 701 #define CPU_SCS_NVIC_ISER0_SETENA8_M 0x00000100 702 #define CPU_SCS_NVIC_ISER0_SETENA8_S 8 709 #define CPU_SCS_NVIC_ISER0_SETENA7 0x00000080 710 #define CPU_SCS_NVIC_ISER0_SETENA7_BITN 7 711 #define CPU_SCS_NVIC_ISER0_SETENA7_M 0x00000080 712 #define CPU_SCS_NVIC_ISER0_SETENA7_S 7 719 #define CPU_SCS_NVIC_ISER0_SETENA6 0x00000040 720 #define CPU_SCS_NVIC_ISER0_SETENA6_BITN 6 721 #define CPU_SCS_NVIC_ISER0_SETENA6_M 0x00000040 722 #define CPU_SCS_NVIC_ISER0_SETENA6_S 6 729 #define CPU_SCS_NVIC_ISER0_SETENA5 0x00000020 730 #define CPU_SCS_NVIC_ISER0_SETENA5_BITN 5 731 #define CPU_SCS_NVIC_ISER0_SETENA5_M 0x00000020 732 #define CPU_SCS_NVIC_ISER0_SETENA5_S 5 739 #define CPU_SCS_NVIC_ISER0_SETENA4 0x00000010 740 #define CPU_SCS_NVIC_ISER0_SETENA4_BITN 4 741 #define CPU_SCS_NVIC_ISER0_SETENA4_M 0x00000010 742 #define CPU_SCS_NVIC_ISER0_SETENA4_S 4 749 #define CPU_SCS_NVIC_ISER0_SETENA3 0x00000008 750 #define CPU_SCS_NVIC_ISER0_SETENA3_BITN 3 751 #define CPU_SCS_NVIC_ISER0_SETENA3_M 0x00000008 752 #define CPU_SCS_NVIC_ISER0_SETENA3_S 3 759 #define CPU_SCS_NVIC_ISER0_SETENA2 0x00000004 760 #define CPU_SCS_NVIC_ISER0_SETENA2_BITN 2 761 #define CPU_SCS_NVIC_ISER0_SETENA2_M 0x00000004 762 #define CPU_SCS_NVIC_ISER0_SETENA2_S 2 769 #define CPU_SCS_NVIC_ISER0_SETENA1 0x00000002 770 #define CPU_SCS_NVIC_ISER0_SETENA1_BITN 1 771 #define CPU_SCS_NVIC_ISER0_SETENA1_M 0x00000002 772 #define CPU_SCS_NVIC_ISER0_SETENA1_S 1 779 #define CPU_SCS_NVIC_ISER0_SETENA0 0x00000001 780 #define CPU_SCS_NVIC_ISER0_SETENA0_BITN 0 781 #define CPU_SCS_NVIC_ISER0_SETENA0_M 0x00000001 782 #define CPU_SCS_NVIC_ISER0_SETENA0_S 0 794 #define CPU_SCS_NVIC_ISER1_SETENA37 0x00000020 795 #define CPU_SCS_NVIC_ISER1_SETENA37_BITN 5 796 #define CPU_SCS_NVIC_ISER1_SETENA37_M 0x00000020 797 #define CPU_SCS_NVIC_ISER1_SETENA37_S 5 804 #define CPU_SCS_NVIC_ISER1_SETENA36 0x00000010 805 #define CPU_SCS_NVIC_ISER1_SETENA36_BITN 4 806 #define CPU_SCS_NVIC_ISER1_SETENA36_M 0x00000010 807 #define CPU_SCS_NVIC_ISER1_SETENA36_S 4 814 #define CPU_SCS_NVIC_ISER1_SETENA35 0x00000008 815 #define CPU_SCS_NVIC_ISER1_SETENA35_BITN 3 816 #define CPU_SCS_NVIC_ISER1_SETENA35_M 0x00000008 817 #define CPU_SCS_NVIC_ISER1_SETENA35_S 3 824 #define CPU_SCS_NVIC_ISER1_SETENA34 0x00000004 825 #define CPU_SCS_NVIC_ISER1_SETENA34_BITN 2 826 #define CPU_SCS_NVIC_ISER1_SETENA34_M 0x00000004 827 #define CPU_SCS_NVIC_ISER1_SETENA34_S 2 834 #define CPU_SCS_NVIC_ISER1_SETENA33 0x00000002 835 #define CPU_SCS_NVIC_ISER1_SETENA33_BITN 1 836 #define CPU_SCS_NVIC_ISER1_SETENA33_M 0x00000002 837 #define CPU_SCS_NVIC_ISER1_SETENA33_S 1 844 #define CPU_SCS_NVIC_ISER1_SETENA32 0x00000001 845 #define CPU_SCS_NVIC_ISER1_SETENA32_BITN 0 846 #define CPU_SCS_NVIC_ISER1_SETENA32_M 0x00000001 847 #define CPU_SCS_NVIC_ISER1_SETENA32_S 0 859 #define CPU_SCS_NVIC_ICER0_CLRENA31 0x80000000 860 #define CPU_SCS_NVIC_ICER0_CLRENA31_BITN 31 861 #define CPU_SCS_NVIC_ICER0_CLRENA31_M 0x80000000 862 #define CPU_SCS_NVIC_ICER0_CLRENA31_S 31 869 #define CPU_SCS_NVIC_ICER0_CLRENA30 0x40000000 870 #define CPU_SCS_NVIC_ICER0_CLRENA30_BITN 30 871 #define CPU_SCS_NVIC_ICER0_CLRENA30_M 0x40000000 872 #define CPU_SCS_NVIC_ICER0_CLRENA30_S 30 879 #define CPU_SCS_NVIC_ICER0_CLRENA29 0x20000000 880 #define CPU_SCS_NVIC_ICER0_CLRENA29_BITN 29 881 #define CPU_SCS_NVIC_ICER0_CLRENA29_M 0x20000000 882 #define CPU_SCS_NVIC_ICER0_CLRENA29_S 29 889 #define CPU_SCS_NVIC_ICER0_CLRENA28 0x10000000 890 #define CPU_SCS_NVIC_ICER0_CLRENA28_BITN 28 891 #define CPU_SCS_NVIC_ICER0_CLRENA28_M 0x10000000 892 #define CPU_SCS_NVIC_ICER0_CLRENA28_S 28 899 #define CPU_SCS_NVIC_ICER0_CLRENA27 0x08000000 900 #define CPU_SCS_NVIC_ICER0_CLRENA27_BITN 27 901 #define CPU_SCS_NVIC_ICER0_CLRENA27_M 0x08000000 902 #define CPU_SCS_NVIC_ICER0_CLRENA27_S 27 909 #define CPU_SCS_NVIC_ICER0_CLRENA26 0x04000000 910 #define CPU_SCS_NVIC_ICER0_CLRENA26_BITN 26 911 #define CPU_SCS_NVIC_ICER0_CLRENA26_M 0x04000000 912 #define CPU_SCS_NVIC_ICER0_CLRENA26_S 26 919 #define CPU_SCS_NVIC_ICER0_CLRENA25 0x02000000 920 #define CPU_SCS_NVIC_ICER0_CLRENA25_BITN 25 921 #define CPU_SCS_NVIC_ICER0_CLRENA25_M 0x02000000 922 #define CPU_SCS_NVIC_ICER0_CLRENA25_S 25 929 #define CPU_SCS_NVIC_ICER0_CLRENA24 0x01000000 930 #define CPU_SCS_NVIC_ICER0_CLRENA24_BITN 24 931 #define CPU_SCS_NVIC_ICER0_CLRENA24_M 0x01000000 932 #define CPU_SCS_NVIC_ICER0_CLRENA24_S 24 939 #define CPU_SCS_NVIC_ICER0_CLRENA23 0x00800000 940 #define CPU_SCS_NVIC_ICER0_CLRENA23_BITN 23 941 #define CPU_SCS_NVIC_ICER0_CLRENA23_M 0x00800000 942 #define CPU_SCS_NVIC_ICER0_CLRENA23_S 23 949 #define CPU_SCS_NVIC_ICER0_CLRENA22 0x00400000 950 #define CPU_SCS_NVIC_ICER0_CLRENA22_BITN 22 951 #define CPU_SCS_NVIC_ICER0_CLRENA22_M 0x00400000 952 #define CPU_SCS_NVIC_ICER0_CLRENA22_S 22 959 #define CPU_SCS_NVIC_ICER0_CLRENA21 0x00200000 960 #define CPU_SCS_NVIC_ICER0_CLRENA21_BITN 21 961 #define CPU_SCS_NVIC_ICER0_CLRENA21_M 0x00200000 962 #define CPU_SCS_NVIC_ICER0_CLRENA21_S 21 969 #define CPU_SCS_NVIC_ICER0_CLRENA20 0x00100000 970 #define CPU_SCS_NVIC_ICER0_CLRENA20_BITN 20 971 #define CPU_SCS_NVIC_ICER0_CLRENA20_M 0x00100000 972 #define CPU_SCS_NVIC_ICER0_CLRENA20_S 20 979 #define CPU_SCS_NVIC_ICER0_CLRENA19 0x00080000 980 #define CPU_SCS_NVIC_ICER0_CLRENA19_BITN 19 981 #define CPU_SCS_NVIC_ICER0_CLRENA19_M 0x00080000 982 #define CPU_SCS_NVIC_ICER0_CLRENA19_S 19 989 #define CPU_SCS_NVIC_ICER0_CLRENA18 0x00040000 990 #define CPU_SCS_NVIC_ICER0_CLRENA18_BITN 18 991 #define CPU_SCS_NVIC_ICER0_CLRENA18_M 0x00040000 992 #define CPU_SCS_NVIC_ICER0_CLRENA18_S 18 999 #define CPU_SCS_NVIC_ICER0_CLRENA17 0x00020000 1000 #define CPU_SCS_NVIC_ICER0_CLRENA17_BITN 17 1001 #define CPU_SCS_NVIC_ICER0_CLRENA17_M 0x00020000 1002 #define CPU_SCS_NVIC_ICER0_CLRENA17_S 17 1009 #define CPU_SCS_NVIC_ICER0_CLRENA16 0x00010000 1010 #define CPU_SCS_NVIC_ICER0_CLRENA16_BITN 16 1011 #define CPU_SCS_NVIC_ICER0_CLRENA16_M 0x00010000 1012 #define CPU_SCS_NVIC_ICER0_CLRENA16_S 16 1019 #define CPU_SCS_NVIC_ICER0_CLRENA15 0x00008000 1020 #define CPU_SCS_NVIC_ICER0_CLRENA15_BITN 15 1021 #define CPU_SCS_NVIC_ICER0_CLRENA15_M 0x00008000 1022 #define CPU_SCS_NVIC_ICER0_CLRENA15_S 15 1029 #define CPU_SCS_NVIC_ICER0_CLRENA14 0x00004000 1030 #define CPU_SCS_NVIC_ICER0_CLRENA14_BITN 14 1031 #define CPU_SCS_NVIC_ICER0_CLRENA14_M 0x00004000 1032 #define CPU_SCS_NVIC_ICER0_CLRENA14_S 14 1039 #define CPU_SCS_NVIC_ICER0_CLRENA13 0x00002000 1040 #define CPU_SCS_NVIC_ICER0_CLRENA13_BITN 13 1041 #define CPU_SCS_NVIC_ICER0_CLRENA13_M 0x00002000 1042 #define CPU_SCS_NVIC_ICER0_CLRENA13_S 13 1049 #define CPU_SCS_NVIC_ICER0_CLRENA12 0x00001000 1050 #define CPU_SCS_NVIC_ICER0_CLRENA12_BITN 12 1051 #define CPU_SCS_NVIC_ICER0_CLRENA12_M 0x00001000 1052 #define CPU_SCS_NVIC_ICER0_CLRENA12_S 12 1059 #define CPU_SCS_NVIC_ICER0_CLRENA11 0x00000800 1060 #define CPU_SCS_NVIC_ICER0_CLRENA11_BITN 11 1061 #define CPU_SCS_NVIC_ICER0_CLRENA11_M 0x00000800 1062 #define CPU_SCS_NVIC_ICER0_CLRENA11_S 11 1069 #define CPU_SCS_NVIC_ICER0_CLRENA10 0x00000400 1070 #define CPU_SCS_NVIC_ICER0_CLRENA10_BITN 10 1071 #define CPU_SCS_NVIC_ICER0_CLRENA10_M 0x00000400 1072 #define CPU_SCS_NVIC_ICER0_CLRENA10_S 10 1079 #define CPU_SCS_NVIC_ICER0_CLRENA9 0x00000200 1080 #define CPU_SCS_NVIC_ICER0_CLRENA9_BITN 9 1081 #define CPU_SCS_NVIC_ICER0_CLRENA9_M 0x00000200 1082 #define CPU_SCS_NVIC_ICER0_CLRENA9_S 9 1089 #define CPU_SCS_NVIC_ICER0_CLRENA8 0x00000100 1090 #define CPU_SCS_NVIC_ICER0_CLRENA8_BITN 8 1091 #define CPU_SCS_NVIC_ICER0_CLRENA8_M 0x00000100 1092 #define CPU_SCS_NVIC_ICER0_CLRENA8_S 8 1099 #define CPU_SCS_NVIC_ICER0_CLRENA7 0x00000080 1100 #define CPU_SCS_NVIC_ICER0_CLRENA7_BITN 7 1101 #define CPU_SCS_NVIC_ICER0_CLRENA7_M 0x00000080 1102 #define CPU_SCS_NVIC_ICER0_CLRENA7_S 7 1109 #define CPU_SCS_NVIC_ICER0_CLRENA6 0x00000040 1110 #define CPU_SCS_NVIC_ICER0_CLRENA6_BITN 6 1111 #define CPU_SCS_NVIC_ICER0_CLRENA6_M 0x00000040 1112 #define CPU_SCS_NVIC_ICER0_CLRENA6_S 6 1119 #define CPU_SCS_NVIC_ICER0_CLRENA5 0x00000020 1120 #define CPU_SCS_NVIC_ICER0_CLRENA5_BITN 5 1121 #define CPU_SCS_NVIC_ICER0_CLRENA5_M 0x00000020 1122 #define CPU_SCS_NVIC_ICER0_CLRENA5_S 5 1129 #define CPU_SCS_NVIC_ICER0_CLRENA4 0x00000010 1130 #define CPU_SCS_NVIC_ICER0_CLRENA4_BITN 4 1131 #define CPU_SCS_NVIC_ICER0_CLRENA4_M 0x00000010 1132 #define CPU_SCS_NVIC_ICER0_CLRENA4_S 4 1139 #define CPU_SCS_NVIC_ICER0_CLRENA3 0x00000008 1140 #define CPU_SCS_NVIC_ICER0_CLRENA3_BITN 3 1141 #define CPU_SCS_NVIC_ICER0_CLRENA3_M 0x00000008 1142 #define CPU_SCS_NVIC_ICER0_CLRENA3_S 3 1149 #define CPU_SCS_NVIC_ICER0_CLRENA2 0x00000004 1150 #define CPU_SCS_NVIC_ICER0_CLRENA2_BITN 2 1151 #define CPU_SCS_NVIC_ICER0_CLRENA2_M 0x00000004 1152 #define CPU_SCS_NVIC_ICER0_CLRENA2_S 2 1159 #define CPU_SCS_NVIC_ICER0_CLRENA1 0x00000002 1160 #define CPU_SCS_NVIC_ICER0_CLRENA1_BITN 1 1161 #define CPU_SCS_NVIC_ICER0_CLRENA1_M 0x00000002 1162 #define CPU_SCS_NVIC_ICER0_CLRENA1_S 1 1169 #define CPU_SCS_NVIC_ICER0_CLRENA0 0x00000001 1170 #define CPU_SCS_NVIC_ICER0_CLRENA0_BITN 0 1171 #define CPU_SCS_NVIC_ICER0_CLRENA0_M 0x00000001 1172 #define CPU_SCS_NVIC_ICER0_CLRENA0_S 0 1184 #define CPU_SCS_NVIC_ICER1_CLRENA37 0x00000020 1185 #define CPU_SCS_NVIC_ICER1_CLRENA37_BITN 5 1186 #define CPU_SCS_NVIC_ICER1_CLRENA37_M 0x00000020 1187 #define CPU_SCS_NVIC_ICER1_CLRENA37_S 5 1194 #define CPU_SCS_NVIC_ICER1_CLRENA36 0x00000010 1195 #define CPU_SCS_NVIC_ICER1_CLRENA36_BITN 4 1196 #define CPU_SCS_NVIC_ICER1_CLRENA36_M 0x00000010 1197 #define CPU_SCS_NVIC_ICER1_CLRENA36_S 4 1204 #define CPU_SCS_NVIC_ICER1_CLRENA35 0x00000008 1205 #define CPU_SCS_NVIC_ICER1_CLRENA35_BITN 3 1206 #define CPU_SCS_NVIC_ICER1_CLRENA35_M 0x00000008 1207 #define CPU_SCS_NVIC_ICER1_CLRENA35_S 3 1214 #define CPU_SCS_NVIC_ICER1_CLRENA34 0x00000004 1215 #define CPU_SCS_NVIC_ICER1_CLRENA34_BITN 2 1216 #define CPU_SCS_NVIC_ICER1_CLRENA34_M 0x00000004 1217 #define CPU_SCS_NVIC_ICER1_CLRENA34_S 2 1224 #define CPU_SCS_NVIC_ICER1_CLRENA33 0x00000002 1225 #define CPU_SCS_NVIC_ICER1_CLRENA33_BITN 1 1226 #define CPU_SCS_NVIC_ICER1_CLRENA33_M 0x00000002 1227 #define CPU_SCS_NVIC_ICER1_CLRENA33_S 1 1234 #define CPU_SCS_NVIC_ICER1_CLRENA32 0x00000001 1235 #define CPU_SCS_NVIC_ICER1_CLRENA32_BITN 0 1236 #define CPU_SCS_NVIC_ICER1_CLRENA32_M 0x00000001 1237 #define CPU_SCS_NVIC_ICER1_CLRENA32_S 0 1249 #define CPU_SCS_NVIC_ISPR0_SETPEND31 0x80000000 1250 #define CPU_SCS_NVIC_ISPR0_SETPEND31_BITN 31 1251 #define CPU_SCS_NVIC_ISPR0_SETPEND31_M 0x80000000 1252 #define CPU_SCS_NVIC_ISPR0_SETPEND31_S 31 1259 #define CPU_SCS_NVIC_ISPR0_SETPEND30 0x40000000 1260 #define CPU_SCS_NVIC_ISPR0_SETPEND30_BITN 30 1261 #define CPU_SCS_NVIC_ISPR0_SETPEND30_M 0x40000000 1262 #define CPU_SCS_NVIC_ISPR0_SETPEND30_S 30 1269 #define CPU_SCS_NVIC_ISPR0_SETPEND29 0x20000000 1270 #define CPU_SCS_NVIC_ISPR0_SETPEND29_BITN 29 1271 #define CPU_SCS_NVIC_ISPR0_SETPEND29_M 0x20000000 1272 #define CPU_SCS_NVIC_ISPR0_SETPEND29_S 29 1279 #define CPU_SCS_NVIC_ISPR0_SETPEND28 0x10000000 1280 #define CPU_SCS_NVIC_ISPR0_SETPEND28_BITN 28 1281 #define CPU_SCS_NVIC_ISPR0_SETPEND28_M 0x10000000 1282 #define CPU_SCS_NVIC_ISPR0_SETPEND28_S 28 1289 #define CPU_SCS_NVIC_ISPR0_SETPEND27 0x08000000 1290 #define CPU_SCS_NVIC_ISPR0_SETPEND27_BITN 27 1291 #define CPU_SCS_NVIC_ISPR0_SETPEND27_M 0x08000000 1292 #define CPU_SCS_NVIC_ISPR0_SETPEND27_S 27 1299 #define CPU_SCS_NVIC_ISPR0_SETPEND26 0x04000000 1300 #define CPU_SCS_NVIC_ISPR0_SETPEND26_BITN 26 1301 #define CPU_SCS_NVIC_ISPR0_SETPEND26_M 0x04000000 1302 #define CPU_SCS_NVIC_ISPR0_SETPEND26_S 26 1309 #define CPU_SCS_NVIC_ISPR0_SETPEND25 0x02000000 1310 #define CPU_SCS_NVIC_ISPR0_SETPEND25_BITN 25 1311 #define CPU_SCS_NVIC_ISPR0_SETPEND25_M 0x02000000 1312 #define CPU_SCS_NVIC_ISPR0_SETPEND25_S 25 1319 #define CPU_SCS_NVIC_ISPR0_SETPEND24 0x01000000 1320 #define CPU_SCS_NVIC_ISPR0_SETPEND24_BITN 24 1321 #define CPU_SCS_NVIC_ISPR0_SETPEND24_M 0x01000000 1322 #define CPU_SCS_NVIC_ISPR0_SETPEND24_S 24 1329 #define CPU_SCS_NVIC_ISPR0_SETPEND23 0x00800000 1330 #define CPU_SCS_NVIC_ISPR0_SETPEND23_BITN 23 1331 #define CPU_SCS_NVIC_ISPR0_SETPEND23_M 0x00800000 1332 #define CPU_SCS_NVIC_ISPR0_SETPEND23_S 23 1339 #define CPU_SCS_NVIC_ISPR0_SETPEND22 0x00400000 1340 #define CPU_SCS_NVIC_ISPR0_SETPEND22_BITN 22 1341 #define CPU_SCS_NVIC_ISPR0_SETPEND22_M 0x00400000 1342 #define CPU_SCS_NVIC_ISPR0_SETPEND22_S 22 1349 #define CPU_SCS_NVIC_ISPR0_SETPEND21 0x00200000 1350 #define CPU_SCS_NVIC_ISPR0_SETPEND21_BITN 21 1351 #define CPU_SCS_NVIC_ISPR0_SETPEND21_M 0x00200000 1352 #define CPU_SCS_NVIC_ISPR0_SETPEND21_S 21 1359 #define CPU_SCS_NVIC_ISPR0_SETPEND20 0x00100000 1360 #define CPU_SCS_NVIC_ISPR0_SETPEND20_BITN 20 1361 #define CPU_SCS_NVIC_ISPR0_SETPEND20_M 0x00100000 1362 #define CPU_SCS_NVIC_ISPR0_SETPEND20_S 20 1369 #define CPU_SCS_NVIC_ISPR0_SETPEND19 0x00080000 1370 #define CPU_SCS_NVIC_ISPR0_SETPEND19_BITN 19 1371 #define CPU_SCS_NVIC_ISPR0_SETPEND19_M 0x00080000 1372 #define CPU_SCS_NVIC_ISPR0_SETPEND19_S 19 1379 #define CPU_SCS_NVIC_ISPR0_SETPEND18 0x00040000 1380 #define CPU_SCS_NVIC_ISPR0_SETPEND18_BITN 18 1381 #define CPU_SCS_NVIC_ISPR0_SETPEND18_M 0x00040000 1382 #define CPU_SCS_NVIC_ISPR0_SETPEND18_S 18 1389 #define CPU_SCS_NVIC_ISPR0_SETPEND17 0x00020000 1390 #define CPU_SCS_NVIC_ISPR0_SETPEND17_BITN 17 1391 #define CPU_SCS_NVIC_ISPR0_SETPEND17_M 0x00020000 1392 #define CPU_SCS_NVIC_ISPR0_SETPEND17_S 17 1399 #define CPU_SCS_NVIC_ISPR0_SETPEND16 0x00010000 1400 #define CPU_SCS_NVIC_ISPR0_SETPEND16_BITN 16 1401 #define CPU_SCS_NVIC_ISPR0_SETPEND16_M 0x00010000 1402 #define CPU_SCS_NVIC_ISPR0_SETPEND16_S 16 1409 #define CPU_SCS_NVIC_ISPR0_SETPEND15 0x00008000 1410 #define CPU_SCS_NVIC_ISPR0_SETPEND15_BITN 15 1411 #define CPU_SCS_NVIC_ISPR0_SETPEND15_M 0x00008000 1412 #define CPU_SCS_NVIC_ISPR0_SETPEND15_S 15 1419 #define CPU_SCS_NVIC_ISPR0_SETPEND14 0x00004000 1420 #define CPU_SCS_NVIC_ISPR0_SETPEND14_BITN 14 1421 #define CPU_SCS_NVIC_ISPR0_SETPEND14_M 0x00004000 1422 #define CPU_SCS_NVIC_ISPR0_SETPEND14_S 14 1429 #define CPU_SCS_NVIC_ISPR0_SETPEND13 0x00002000 1430 #define CPU_SCS_NVIC_ISPR0_SETPEND13_BITN 13 1431 #define CPU_SCS_NVIC_ISPR0_SETPEND13_M 0x00002000 1432 #define CPU_SCS_NVIC_ISPR0_SETPEND13_S 13 1439 #define CPU_SCS_NVIC_ISPR0_SETPEND12 0x00001000 1440 #define CPU_SCS_NVIC_ISPR0_SETPEND12_BITN 12 1441 #define CPU_SCS_NVIC_ISPR0_SETPEND12_M 0x00001000 1442 #define CPU_SCS_NVIC_ISPR0_SETPEND12_S 12 1449 #define CPU_SCS_NVIC_ISPR0_SETPEND11 0x00000800 1450 #define CPU_SCS_NVIC_ISPR0_SETPEND11_BITN 11 1451 #define CPU_SCS_NVIC_ISPR0_SETPEND11_M 0x00000800 1452 #define CPU_SCS_NVIC_ISPR0_SETPEND11_S 11 1459 #define CPU_SCS_NVIC_ISPR0_SETPEND10 0x00000400 1460 #define CPU_SCS_NVIC_ISPR0_SETPEND10_BITN 10 1461 #define CPU_SCS_NVIC_ISPR0_SETPEND10_M 0x00000400 1462 #define CPU_SCS_NVIC_ISPR0_SETPEND10_S 10 1469 #define CPU_SCS_NVIC_ISPR0_SETPEND9 0x00000200 1470 #define CPU_SCS_NVIC_ISPR0_SETPEND9_BITN 9 1471 #define CPU_SCS_NVIC_ISPR0_SETPEND9_M 0x00000200 1472 #define CPU_SCS_NVIC_ISPR0_SETPEND9_S 9 1479 #define CPU_SCS_NVIC_ISPR0_SETPEND8 0x00000100 1480 #define CPU_SCS_NVIC_ISPR0_SETPEND8_BITN 8 1481 #define CPU_SCS_NVIC_ISPR0_SETPEND8_M 0x00000100 1482 #define CPU_SCS_NVIC_ISPR0_SETPEND8_S 8 1489 #define CPU_SCS_NVIC_ISPR0_SETPEND7 0x00000080 1490 #define CPU_SCS_NVIC_ISPR0_SETPEND7_BITN 7 1491 #define CPU_SCS_NVIC_ISPR0_SETPEND7_M 0x00000080 1492 #define CPU_SCS_NVIC_ISPR0_SETPEND7_S 7 1499 #define CPU_SCS_NVIC_ISPR0_SETPEND6 0x00000040 1500 #define CPU_SCS_NVIC_ISPR0_SETPEND6_BITN 6 1501 #define CPU_SCS_NVIC_ISPR0_SETPEND6_M 0x00000040 1502 #define CPU_SCS_NVIC_ISPR0_SETPEND6_S 6 1509 #define CPU_SCS_NVIC_ISPR0_SETPEND5 0x00000020 1510 #define CPU_SCS_NVIC_ISPR0_SETPEND5_BITN 5 1511 #define CPU_SCS_NVIC_ISPR0_SETPEND5_M 0x00000020 1512 #define CPU_SCS_NVIC_ISPR0_SETPEND5_S 5 1519 #define CPU_SCS_NVIC_ISPR0_SETPEND4 0x00000010 1520 #define CPU_SCS_NVIC_ISPR0_SETPEND4_BITN 4 1521 #define CPU_SCS_NVIC_ISPR0_SETPEND4_M 0x00000010 1522 #define CPU_SCS_NVIC_ISPR0_SETPEND4_S 4 1529 #define CPU_SCS_NVIC_ISPR0_SETPEND3 0x00000008 1530 #define CPU_SCS_NVIC_ISPR0_SETPEND3_BITN 3 1531 #define CPU_SCS_NVIC_ISPR0_SETPEND3_M 0x00000008 1532 #define CPU_SCS_NVIC_ISPR0_SETPEND3_S 3 1539 #define CPU_SCS_NVIC_ISPR0_SETPEND2 0x00000004 1540 #define CPU_SCS_NVIC_ISPR0_SETPEND2_BITN 2 1541 #define CPU_SCS_NVIC_ISPR0_SETPEND2_M 0x00000004 1542 #define CPU_SCS_NVIC_ISPR0_SETPEND2_S 2 1549 #define CPU_SCS_NVIC_ISPR0_SETPEND1 0x00000002 1550 #define CPU_SCS_NVIC_ISPR0_SETPEND1_BITN 1 1551 #define CPU_SCS_NVIC_ISPR0_SETPEND1_M 0x00000002 1552 #define CPU_SCS_NVIC_ISPR0_SETPEND1_S 1 1559 #define CPU_SCS_NVIC_ISPR0_SETPEND0 0x00000001 1560 #define CPU_SCS_NVIC_ISPR0_SETPEND0_BITN 0 1561 #define CPU_SCS_NVIC_ISPR0_SETPEND0_M 0x00000001 1562 #define CPU_SCS_NVIC_ISPR0_SETPEND0_S 0 1574 #define CPU_SCS_NVIC_ISPR1_SETPEND37 0x00000020 1575 #define CPU_SCS_NVIC_ISPR1_SETPEND37_BITN 5 1576 #define CPU_SCS_NVIC_ISPR1_SETPEND37_M 0x00000020 1577 #define CPU_SCS_NVIC_ISPR1_SETPEND37_S 5 1584 #define CPU_SCS_NVIC_ISPR1_SETPEND36 0x00000010 1585 #define CPU_SCS_NVIC_ISPR1_SETPEND36_BITN 4 1586 #define CPU_SCS_NVIC_ISPR1_SETPEND36_M 0x00000010 1587 #define CPU_SCS_NVIC_ISPR1_SETPEND36_S 4 1594 #define CPU_SCS_NVIC_ISPR1_SETPEND35 0x00000008 1595 #define CPU_SCS_NVIC_ISPR1_SETPEND35_BITN 3 1596 #define CPU_SCS_NVIC_ISPR1_SETPEND35_M 0x00000008 1597 #define CPU_SCS_NVIC_ISPR1_SETPEND35_S 3 1604 #define CPU_SCS_NVIC_ISPR1_SETPEND34 0x00000004 1605 #define CPU_SCS_NVIC_ISPR1_SETPEND34_BITN 2 1606 #define CPU_SCS_NVIC_ISPR1_SETPEND34_M 0x00000004 1607 #define CPU_SCS_NVIC_ISPR1_SETPEND34_S 2 1614 #define CPU_SCS_NVIC_ISPR1_SETPEND33 0x00000002 1615 #define CPU_SCS_NVIC_ISPR1_SETPEND33_BITN 1 1616 #define CPU_SCS_NVIC_ISPR1_SETPEND33_M 0x00000002 1617 #define CPU_SCS_NVIC_ISPR1_SETPEND33_S 1 1624 #define CPU_SCS_NVIC_ISPR1_SETPEND32 0x00000001 1625 #define CPU_SCS_NVIC_ISPR1_SETPEND32_BITN 0 1626 #define CPU_SCS_NVIC_ISPR1_SETPEND32_M 0x00000001 1627 #define CPU_SCS_NVIC_ISPR1_SETPEND32_S 0 1639 #define CPU_SCS_NVIC_ICPR0_CLRPEND31 0x80000000 1640 #define CPU_SCS_NVIC_ICPR0_CLRPEND31_BITN 31 1641 #define CPU_SCS_NVIC_ICPR0_CLRPEND31_M 0x80000000 1642 #define CPU_SCS_NVIC_ICPR0_CLRPEND31_S 31 1649 #define CPU_SCS_NVIC_ICPR0_CLRPEND30 0x40000000 1650 #define CPU_SCS_NVIC_ICPR0_CLRPEND30_BITN 30 1651 #define CPU_SCS_NVIC_ICPR0_CLRPEND30_M 0x40000000 1652 #define CPU_SCS_NVIC_ICPR0_CLRPEND30_S 30 1659 #define CPU_SCS_NVIC_ICPR0_CLRPEND29 0x20000000 1660 #define CPU_SCS_NVIC_ICPR0_CLRPEND29_BITN 29 1661 #define CPU_SCS_NVIC_ICPR0_CLRPEND29_M 0x20000000 1662 #define CPU_SCS_NVIC_ICPR0_CLRPEND29_S 29 1669 #define CPU_SCS_NVIC_ICPR0_CLRPEND28 0x10000000 1670 #define CPU_SCS_NVIC_ICPR0_CLRPEND28_BITN 28 1671 #define CPU_SCS_NVIC_ICPR0_CLRPEND28_M 0x10000000 1672 #define CPU_SCS_NVIC_ICPR0_CLRPEND28_S 28 1679 #define CPU_SCS_NVIC_ICPR0_CLRPEND27 0x08000000 1680 #define CPU_SCS_NVIC_ICPR0_CLRPEND27_BITN 27 1681 #define CPU_SCS_NVIC_ICPR0_CLRPEND27_M 0x08000000 1682 #define CPU_SCS_NVIC_ICPR0_CLRPEND27_S 27 1689 #define CPU_SCS_NVIC_ICPR0_CLRPEND26 0x04000000 1690 #define CPU_SCS_NVIC_ICPR0_CLRPEND26_BITN 26 1691 #define CPU_SCS_NVIC_ICPR0_CLRPEND26_M 0x04000000 1692 #define CPU_SCS_NVIC_ICPR0_CLRPEND26_S 26 1699 #define CPU_SCS_NVIC_ICPR0_CLRPEND25 0x02000000 1700 #define CPU_SCS_NVIC_ICPR0_CLRPEND25_BITN 25 1701 #define CPU_SCS_NVIC_ICPR0_CLRPEND25_M 0x02000000 1702 #define CPU_SCS_NVIC_ICPR0_CLRPEND25_S 25 1709 #define CPU_SCS_NVIC_ICPR0_CLRPEND24 0x01000000 1710 #define CPU_SCS_NVIC_ICPR0_CLRPEND24_BITN 24 1711 #define CPU_SCS_NVIC_ICPR0_CLRPEND24_M 0x01000000 1712 #define CPU_SCS_NVIC_ICPR0_CLRPEND24_S 24 1719 #define CPU_SCS_NVIC_ICPR0_CLRPEND23 0x00800000 1720 #define CPU_SCS_NVIC_ICPR0_CLRPEND23_BITN 23 1721 #define CPU_SCS_NVIC_ICPR0_CLRPEND23_M 0x00800000 1722 #define CPU_SCS_NVIC_ICPR0_CLRPEND23_S 23 1729 #define CPU_SCS_NVIC_ICPR0_CLRPEND22 0x00400000 1730 #define CPU_SCS_NVIC_ICPR0_CLRPEND22_BITN 22 1731 #define CPU_SCS_NVIC_ICPR0_CLRPEND22_M 0x00400000 1732 #define CPU_SCS_NVIC_ICPR0_CLRPEND22_S 22 1739 #define CPU_SCS_NVIC_ICPR0_CLRPEND21 0x00200000 1740 #define CPU_SCS_NVIC_ICPR0_CLRPEND21_BITN 21 1741 #define CPU_SCS_NVIC_ICPR0_CLRPEND21_M 0x00200000 1742 #define CPU_SCS_NVIC_ICPR0_CLRPEND21_S 21 1749 #define CPU_SCS_NVIC_ICPR0_CLRPEND20 0x00100000 1750 #define CPU_SCS_NVIC_ICPR0_CLRPEND20_BITN 20 1751 #define CPU_SCS_NVIC_ICPR0_CLRPEND20_M 0x00100000 1752 #define CPU_SCS_NVIC_ICPR0_CLRPEND20_S 20 1759 #define CPU_SCS_NVIC_ICPR0_CLRPEND19 0x00080000 1760 #define CPU_SCS_NVIC_ICPR0_CLRPEND19_BITN 19 1761 #define CPU_SCS_NVIC_ICPR0_CLRPEND19_M 0x00080000 1762 #define CPU_SCS_NVIC_ICPR0_CLRPEND19_S 19 1769 #define CPU_SCS_NVIC_ICPR0_CLRPEND18 0x00040000 1770 #define CPU_SCS_NVIC_ICPR0_CLRPEND18_BITN 18 1771 #define CPU_SCS_NVIC_ICPR0_CLRPEND18_M 0x00040000 1772 #define CPU_SCS_NVIC_ICPR0_CLRPEND18_S 18 1779 #define CPU_SCS_NVIC_ICPR0_CLRPEND17 0x00020000 1780 #define CPU_SCS_NVIC_ICPR0_CLRPEND17_BITN 17 1781 #define CPU_SCS_NVIC_ICPR0_CLRPEND17_M 0x00020000 1782 #define CPU_SCS_NVIC_ICPR0_CLRPEND17_S 17 1789 #define CPU_SCS_NVIC_ICPR0_CLRPEND16 0x00010000 1790 #define CPU_SCS_NVIC_ICPR0_CLRPEND16_BITN 16 1791 #define CPU_SCS_NVIC_ICPR0_CLRPEND16_M 0x00010000 1792 #define CPU_SCS_NVIC_ICPR0_CLRPEND16_S 16 1799 #define CPU_SCS_NVIC_ICPR0_CLRPEND15 0x00008000 1800 #define CPU_SCS_NVIC_ICPR0_CLRPEND15_BITN 15 1801 #define CPU_SCS_NVIC_ICPR0_CLRPEND15_M 0x00008000 1802 #define CPU_SCS_NVIC_ICPR0_CLRPEND15_S 15 1809 #define CPU_SCS_NVIC_ICPR0_CLRPEND14 0x00004000 1810 #define CPU_SCS_NVIC_ICPR0_CLRPEND14_BITN 14 1811 #define CPU_SCS_NVIC_ICPR0_CLRPEND14_M 0x00004000 1812 #define CPU_SCS_NVIC_ICPR0_CLRPEND14_S 14 1819 #define CPU_SCS_NVIC_ICPR0_CLRPEND13 0x00002000 1820 #define CPU_SCS_NVIC_ICPR0_CLRPEND13_BITN 13 1821 #define CPU_SCS_NVIC_ICPR0_CLRPEND13_M 0x00002000 1822 #define CPU_SCS_NVIC_ICPR0_CLRPEND13_S 13 1829 #define CPU_SCS_NVIC_ICPR0_CLRPEND12 0x00001000 1830 #define CPU_SCS_NVIC_ICPR0_CLRPEND12_BITN 12 1831 #define CPU_SCS_NVIC_ICPR0_CLRPEND12_M 0x00001000 1832 #define CPU_SCS_NVIC_ICPR0_CLRPEND12_S 12 1839 #define CPU_SCS_NVIC_ICPR0_CLRPEND11 0x00000800 1840 #define CPU_SCS_NVIC_ICPR0_CLRPEND11_BITN 11 1841 #define CPU_SCS_NVIC_ICPR0_CLRPEND11_M 0x00000800 1842 #define CPU_SCS_NVIC_ICPR0_CLRPEND11_S 11 1849 #define CPU_SCS_NVIC_ICPR0_CLRPEND10 0x00000400 1850 #define CPU_SCS_NVIC_ICPR0_CLRPEND10_BITN 10 1851 #define CPU_SCS_NVIC_ICPR0_CLRPEND10_M 0x00000400 1852 #define CPU_SCS_NVIC_ICPR0_CLRPEND10_S 10 1859 #define CPU_SCS_NVIC_ICPR0_CLRPEND9 0x00000200 1860 #define CPU_SCS_NVIC_ICPR0_CLRPEND9_BITN 9 1861 #define CPU_SCS_NVIC_ICPR0_CLRPEND9_M 0x00000200 1862 #define CPU_SCS_NVIC_ICPR0_CLRPEND9_S 9 1869 #define CPU_SCS_NVIC_ICPR0_CLRPEND8 0x00000100 1870 #define CPU_SCS_NVIC_ICPR0_CLRPEND8_BITN 8 1871 #define CPU_SCS_NVIC_ICPR0_CLRPEND8_M 0x00000100 1872 #define CPU_SCS_NVIC_ICPR0_CLRPEND8_S 8 1879 #define CPU_SCS_NVIC_ICPR0_CLRPEND7 0x00000080 1880 #define CPU_SCS_NVIC_ICPR0_CLRPEND7_BITN 7 1881 #define CPU_SCS_NVIC_ICPR0_CLRPEND7_M 0x00000080 1882 #define CPU_SCS_NVIC_ICPR0_CLRPEND7_S 7 1889 #define CPU_SCS_NVIC_ICPR0_CLRPEND6 0x00000040 1890 #define CPU_SCS_NVIC_ICPR0_CLRPEND6_BITN 6 1891 #define CPU_SCS_NVIC_ICPR0_CLRPEND6_M 0x00000040 1892 #define CPU_SCS_NVIC_ICPR0_CLRPEND6_S 6 1899 #define CPU_SCS_NVIC_ICPR0_CLRPEND5 0x00000020 1900 #define CPU_SCS_NVIC_ICPR0_CLRPEND5_BITN 5 1901 #define CPU_SCS_NVIC_ICPR0_CLRPEND5_M 0x00000020 1902 #define CPU_SCS_NVIC_ICPR0_CLRPEND5_S 5 1909 #define CPU_SCS_NVIC_ICPR0_CLRPEND4 0x00000010 1910 #define CPU_SCS_NVIC_ICPR0_CLRPEND4_BITN 4 1911 #define CPU_SCS_NVIC_ICPR0_CLRPEND4_M 0x00000010 1912 #define CPU_SCS_NVIC_ICPR0_CLRPEND4_S 4 1919 #define CPU_SCS_NVIC_ICPR0_CLRPEND3 0x00000008 1920 #define CPU_SCS_NVIC_ICPR0_CLRPEND3_BITN 3 1921 #define CPU_SCS_NVIC_ICPR0_CLRPEND3_M 0x00000008 1922 #define CPU_SCS_NVIC_ICPR0_CLRPEND3_S 3 1929 #define CPU_SCS_NVIC_ICPR0_CLRPEND2 0x00000004 1930 #define CPU_SCS_NVIC_ICPR0_CLRPEND2_BITN 2 1931 #define CPU_SCS_NVIC_ICPR0_CLRPEND2_M 0x00000004 1932 #define CPU_SCS_NVIC_ICPR0_CLRPEND2_S 2 1939 #define CPU_SCS_NVIC_ICPR0_CLRPEND1 0x00000002 1940 #define CPU_SCS_NVIC_ICPR0_CLRPEND1_BITN 1 1941 #define CPU_SCS_NVIC_ICPR0_CLRPEND1_M 0x00000002 1942 #define CPU_SCS_NVIC_ICPR0_CLRPEND1_S 1 1949 #define CPU_SCS_NVIC_ICPR0_CLRPEND0 0x00000001 1950 #define CPU_SCS_NVIC_ICPR0_CLRPEND0_BITN 0 1951 #define CPU_SCS_NVIC_ICPR0_CLRPEND0_M 0x00000001 1952 #define CPU_SCS_NVIC_ICPR0_CLRPEND0_S 0 1964 #define CPU_SCS_NVIC_ICPR1_CLRPEND37 0x00000020 1965 #define CPU_SCS_NVIC_ICPR1_CLRPEND37_BITN 5 1966 #define CPU_SCS_NVIC_ICPR1_CLRPEND37_M 0x00000020 1967 #define CPU_SCS_NVIC_ICPR1_CLRPEND37_S 5 1974 #define CPU_SCS_NVIC_ICPR1_CLRPEND36 0x00000010 1975 #define CPU_SCS_NVIC_ICPR1_CLRPEND36_BITN 4 1976 #define CPU_SCS_NVIC_ICPR1_CLRPEND36_M 0x00000010 1977 #define CPU_SCS_NVIC_ICPR1_CLRPEND36_S 4 1984 #define CPU_SCS_NVIC_ICPR1_CLRPEND35 0x00000008 1985 #define CPU_SCS_NVIC_ICPR1_CLRPEND35_BITN 3 1986 #define CPU_SCS_NVIC_ICPR1_CLRPEND35_M 0x00000008 1987 #define CPU_SCS_NVIC_ICPR1_CLRPEND35_S 3 1994 #define CPU_SCS_NVIC_ICPR1_CLRPEND34 0x00000004 1995 #define CPU_SCS_NVIC_ICPR1_CLRPEND34_BITN 2 1996 #define CPU_SCS_NVIC_ICPR1_CLRPEND34_M 0x00000004 1997 #define CPU_SCS_NVIC_ICPR1_CLRPEND34_S 2 2004 #define CPU_SCS_NVIC_ICPR1_CLRPEND33 0x00000002 2005 #define CPU_SCS_NVIC_ICPR1_CLRPEND33_BITN 1 2006 #define CPU_SCS_NVIC_ICPR1_CLRPEND33_M 0x00000002 2007 #define CPU_SCS_NVIC_ICPR1_CLRPEND33_S 1 2014 #define CPU_SCS_NVIC_ICPR1_CLRPEND32 0x00000001 2015 #define CPU_SCS_NVIC_ICPR1_CLRPEND32_BITN 0 2016 #define CPU_SCS_NVIC_ICPR1_CLRPEND32_M 0x00000001 2017 #define CPU_SCS_NVIC_ICPR1_CLRPEND32_S 0 2029 #define CPU_SCS_NVIC_IABR0_ACTIVE31 0x80000000 2030 #define CPU_SCS_NVIC_IABR0_ACTIVE31_BITN 31 2031 #define CPU_SCS_NVIC_IABR0_ACTIVE31_M 0x80000000 2032 #define CPU_SCS_NVIC_IABR0_ACTIVE31_S 31 2039 #define CPU_SCS_NVIC_IABR0_ACTIVE30 0x40000000 2040 #define CPU_SCS_NVIC_IABR0_ACTIVE30_BITN 30 2041 #define CPU_SCS_NVIC_IABR0_ACTIVE30_M 0x40000000 2042 #define CPU_SCS_NVIC_IABR0_ACTIVE30_S 30 2049 #define CPU_SCS_NVIC_IABR0_ACTIVE29 0x20000000 2050 #define CPU_SCS_NVIC_IABR0_ACTIVE29_BITN 29 2051 #define CPU_SCS_NVIC_IABR0_ACTIVE29_M 0x20000000 2052 #define CPU_SCS_NVIC_IABR0_ACTIVE29_S 29 2059 #define CPU_SCS_NVIC_IABR0_ACTIVE28 0x10000000 2060 #define CPU_SCS_NVIC_IABR0_ACTIVE28_BITN 28 2061 #define CPU_SCS_NVIC_IABR0_ACTIVE28_M 0x10000000 2062 #define CPU_SCS_NVIC_IABR0_ACTIVE28_S 28 2069 #define CPU_SCS_NVIC_IABR0_ACTIVE27 0x08000000 2070 #define CPU_SCS_NVIC_IABR0_ACTIVE27_BITN 27 2071 #define CPU_SCS_NVIC_IABR0_ACTIVE27_M 0x08000000 2072 #define CPU_SCS_NVIC_IABR0_ACTIVE27_S 27 2079 #define CPU_SCS_NVIC_IABR0_ACTIVE26 0x04000000 2080 #define CPU_SCS_NVIC_IABR0_ACTIVE26_BITN 26 2081 #define CPU_SCS_NVIC_IABR0_ACTIVE26_M 0x04000000 2082 #define CPU_SCS_NVIC_IABR0_ACTIVE26_S 26 2089 #define CPU_SCS_NVIC_IABR0_ACTIVE25 0x02000000 2090 #define CPU_SCS_NVIC_IABR0_ACTIVE25_BITN 25 2091 #define CPU_SCS_NVIC_IABR0_ACTIVE25_M 0x02000000 2092 #define CPU_SCS_NVIC_IABR0_ACTIVE25_S 25 2099 #define CPU_SCS_NVIC_IABR0_ACTIVE24 0x01000000 2100 #define CPU_SCS_NVIC_IABR0_ACTIVE24_BITN 24 2101 #define CPU_SCS_NVIC_IABR0_ACTIVE24_M 0x01000000 2102 #define CPU_SCS_NVIC_IABR0_ACTIVE24_S 24 2109 #define CPU_SCS_NVIC_IABR0_ACTIVE23 0x00800000 2110 #define CPU_SCS_NVIC_IABR0_ACTIVE23_BITN 23 2111 #define CPU_SCS_NVIC_IABR0_ACTIVE23_M 0x00800000 2112 #define CPU_SCS_NVIC_IABR0_ACTIVE23_S 23 2119 #define CPU_SCS_NVIC_IABR0_ACTIVE22 0x00400000 2120 #define CPU_SCS_NVIC_IABR0_ACTIVE22_BITN 22 2121 #define CPU_SCS_NVIC_IABR0_ACTIVE22_M 0x00400000 2122 #define CPU_SCS_NVIC_IABR0_ACTIVE22_S 22 2129 #define CPU_SCS_NVIC_IABR0_ACTIVE21 0x00200000 2130 #define CPU_SCS_NVIC_IABR0_ACTIVE21_BITN 21 2131 #define CPU_SCS_NVIC_IABR0_ACTIVE21_M 0x00200000 2132 #define CPU_SCS_NVIC_IABR0_ACTIVE21_S 21 2139 #define CPU_SCS_NVIC_IABR0_ACTIVE20 0x00100000 2140 #define CPU_SCS_NVIC_IABR0_ACTIVE20_BITN 20 2141 #define CPU_SCS_NVIC_IABR0_ACTIVE20_M 0x00100000 2142 #define CPU_SCS_NVIC_IABR0_ACTIVE20_S 20 2149 #define CPU_SCS_NVIC_IABR0_ACTIVE19 0x00080000 2150 #define CPU_SCS_NVIC_IABR0_ACTIVE19_BITN 19 2151 #define CPU_SCS_NVIC_IABR0_ACTIVE19_M 0x00080000 2152 #define CPU_SCS_NVIC_IABR0_ACTIVE19_S 19 2159 #define CPU_SCS_NVIC_IABR0_ACTIVE18 0x00040000 2160 #define CPU_SCS_NVIC_IABR0_ACTIVE18_BITN 18 2161 #define CPU_SCS_NVIC_IABR0_ACTIVE18_M 0x00040000 2162 #define CPU_SCS_NVIC_IABR0_ACTIVE18_S 18 2169 #define CPU_SCS_NVIC_IABR0_ACTIVE17 0x00020000 2170 #define CPU_SCS_NVIC_IABR0_ACTIVE17_BITN 17 2171 #define CPU_SCS_NVIC_IABR0_ACTIVE17_M 0x00020000 2172 #define CPU_SCS_NVIC_IABR0_ACTIVE17_S 17 2179 #define CPU_SCS_NVIC_IABR0_ACTIVE16 0x00010000 2180 #define CPU_SCS_NVIC_IABR0_ACTIVE16_BITN 16 2181 #define CPU_SCS_NVIC_IABR0_ACTIVE16_M 0x00010000 2182 #define CPU_SCS_NVIC_IABR0_ACTIVE16_S 16 2189 #define CPU_SCS_NVIC_IABR0_ACTIVE15 0x00008000 2190 #define CPU_SCS_NVIC_IABR0_ACTIVE15_BITN 15 2191 #define CPU_SCS_NVIC_IABR0_ACTIVE15_M 0x00008000 2192 #define CPU_SCS_NVIC_IABR0_ACTIVE15_S 15 2199 #define CPU_SCS_NVIC_IABR0_ACTIVE14 0x00004000 2200 #define CPU_SCS_NVIC_IABR0_ACTIVE14_BITN 14 2201 #define CPU_SCS_NVIC_IABR0_ACTIVE14_M 0x00004000 2202 #define CPU_SCS_NVIC_IABR0_ACTIVE14_S 14 2209 #define CPU_SCS_NVIC_IABR0_ACTIVE13 0x00002000 2210 #define CPU_SCS_NVIC_IABR0_ACTIVE13_BITN 13 2211 #define CPU_SCS_NVIC_IABR0_ACTIVE13_M 0x00002000 2212 #define CPU_SCS_NVIC_IABR0_ACTIVE13_S 13 2219 #define CPU_SCS_NVIC_IABR0_ACTIVE12 0x00001000 2220 #define CPU_SCS_NVIC_IABR0_ACTIVE12_BITN 12 2221 #define CPU_SCS_NVIC_IABR0_ACTIVE12_M 0x00001000 2222 #define CPU_SCS_NVIC_IABR0_ACTIVE12_S 12 2229 #define CPU_SCS_NVIC_IABR0_ACTIVE11 0x00000800 2230 #define CPU_SCS_NVIC_IABR0_ACTIVE11_BITN 11 2231 #define CPU_SCS_NVIC_IABR0_ACTIVE11_M 0x00000800 2232 #define CPU_SCS_NVIC_IABR0_ACTIVE11_S 11 2239 #define CPU_SCS_NVIC_IABR0_ACTIVE10 0x00000400 2240 #define CPU_SCS_NVIC_IABR0_ACTIVE10_BITN 10 2241 #define CPU_SCS_NVIC_IABR0_ACTIVE10_M 0x00000400 2242 #define CPU_SCS_NVIC_IABR0_ACTIVE10_S 10 2249 #define CPU_SCS_NVIC_IABR0_ACTIVE9 0x00000200 2250 #define CPU_SCS_NVIC_IABR0_ACTIVE9_BITN 9 2251 #define CPU_SCS_NVIC_IABR0_ACTIVE9_M 0x00000200 2252 #define CPU_SCS_NVIC_IABR0_ACTIVE9_S 9 2259 #define CPU_SCS_NVIC_IABR0_ACTIVE8 0x00000100 2260 #define CPU_SCS_NVIC_IABR0_ACTIVE8_BITN 8 2261 #define CPU_SCS_NVIC_IABR0_ACTIVE8_M 0x00000100 2262 #define CPU_SCS_NVIC_IABR0_ACTIVE8_S 8 2269 #define CPU_SCS_NVIC_IABR0_ACTIVE7 0x00000080 2270 #define CPU_SCS_NVIC_IABR0_ACTIVE7_BITN 7 2271 #define CPU_SCS_NVIC_IABR0_ACTIVE7_M 0x00000080 2272 #define CPU_SCS_NVIC_IABR0_ACTIVE7_S 7 2279 #define CPU_SCS_NVIC_IABR0_ACTIVE6 0x00000040 2280 #define CPU_SCS_NVIC_IABR0_ACTIVE6_BITN 6 2281 #define CPU_SCS_NVIC_IABR0_ACTIVE6_M 0x00000040 2282 #define CPU_SCS_NVIC_IABR0_ACTIVE6_S 6 2289 #define CPU_SCS_NVIC_IABR0_ACTIVE5 0x00000020 2290 #define CPU_SCS_NVIC_IABR0_ACTIVE5_BITN 5 2291 #define CPU_SCS_NVIC_IABR0_ACTIVE5_M 0x00000020 2292 #define CPU_SCS_NVIC_IABR0_ACTIVE5_S 5 2299 #define CPU_SCS_NVIC_IABR0_ACTIVE4 0x00000010 2300 #define CPU_SCS_NVIC_IABR0_ACTIVE4_BITN 4 2301 #define CPU_SCS_NVIC_IABR0_ACTIVE4_M 0x00000010 2302 #define CPU_SCS_NVIC_IABR0_ACTIVE4_S 4 2309 #define CPU_SCS_NVIC_IABR0_ACTIVE3 0x00000008 2310 #define CPU_SCS_NVIC_IABR0_ACTIVE3_BITN 3 2311 #define CPU_SCS_NVIC_IABR0_ACTIVE3_M 0x00000008 2312 #define CPU_SCS_NVIC_IABR0_ACTIVE3_S 3 2319 #define CPU_SCS_NVIC_IABR0_ACTIVE2 0x00000004 2320 #define CPU_SCS_NVIC_IABR0_ACTIVE2_BITN 2 2321 #define CPU_SCS_NVIC_IABR0_ACTIVE2_M 0x00000004 2322 #define CPU_SCS_NVIC_IABR0_ACTIVE2_S 2 2329 #define CPU_SCS_NVIC_IABR0_ACTIVE1 0x00000002 2330 #define CPU_SCS_NVIC_IABR0_ACTIVE1_BITN 1 2331 #define CPU_SCS_NVIC_IABR0_ACTIVE1_M 0x00000002 2332 #define CPU_SCS_NVIC_IABR0_ACTIVE1_S 1 2339 #define CPU_SCS_NVIC_IABR0_ACTIVE0 0x00000001 2340 #define CPU_SCS_NVIC_IABR0_ACTIVE0_BITN 0 2341 #define CPU_SCS_NVIC_IABR0_ACTIVE0_M 0x00000001 2342 #define CPU_SCS_NVIC_IABR0_ACTIVE0_S 0 2354 #define CPU_SCS_NVIC_IABR1_ACTIVE37 0x00000020 2355 #define CPU_SCS_NVIC_IABR1_ACTIVE37_BITN 5 2356 #define CPU_SCS_NVIC_IABR1_ACTIVE37_M 0x00000020 2357 #define CPU_SCS_NVIC_IABR1_ACTIVE37_S 5 2364 #define CPU_SCS_NVIC_IABR1_ACTIVE36 0x00000010 2365 #define CPU_SCS_NVIC_IABR1_ACTIVE36_BITN 4 2366 #define CPU_SCS_NVIC_IABR1_ACTIVE36_M 0x00000010 2367 #define CPU_SCS_NVIC_IABR1_ACTIVE36_S 4 2374 #define CPU_SCS_NVIC_IABR1_ACTIVE35 0x00000008 2375 #define CPU_SCS_NVIC_IABR1_ACTIVE35_BITN 3 2376 #define CPU_SCS_NVIC_IABR1_ACTIVE35_M 0x00000008 2377 #define CPU_SCS_NVIC_IABR1_ACTIVE35_S 3 2384 #define CPU_SCS_NVIC_IABR1_ACTIVE34 0x00000004 2385 #define CPU_SCS_NVIC_IABR1_ACTIVE34_BITN 2 2386 #define CPU_SCS_NVIC_IABR1_ACTIVE34_M 0x00000004 2387 #define CPU_SCS_NVIC_IABR1_ACTIVE34_S 2 2394 #define CPU_SCS_NVIC_IABR1_ACTIVE33 0x00000002 2395 #define CPU_SCS_NVIC_IABR1_ACTIVE33_BITN 1 2396 #define CPU_SCS_NVIC_IABR1_ACTIVE33_M 0x00000002 2397 #define CPU_SCS_NVIC_IABR1_ACTIVE33_S 1 2404 #define CPU_SCS_NVIC_IABR1_ACTIVE32 0x00000001 2405 #define CPU_SCS_NVIC_IABR1_ACTIVE32_BITN 0 2406 #define CPU_SCS_NVIC_IABR1_ACTIVE32_M 0x00000001 2407 #define CPU_SCS_NVIC_IABR1_ACTIVE32_S 0 2417 #define CPU_SCS_NVIC_IPR0_PRI_3_W 8 2418 #define CPU_SCS_NVIC_IPR0_PRI_3_M 0xFF000000 2419 #define CPU_SCS_NVIC_IPR0_PRI_3_S 24 2424 #define CPU_SCS_NVIC_IPR0_PRI_2_W 8 2425 #define CPU_SCS_NVIC_IPR0_PRI_2_M 0x00FF0000 2426 #define CPU_SCS_NVIC_IPR0_PRI_2_S 16 2431 #define CPU_SCS_NVIC_IPR0_PRI_1_W 8 2432 #define CPU_SCS_NVIC_IPR0_PRI_1_M 0x0000FF00 2433 #define CPU_SCS_NVIC_IPR0_PRI_1_S 8 2438 #define CPU_SCS_NVIC_IPR0_PRI_0_W 8 2439 #define CPU_SCS_NVIC_IPR0_PRI_0_M 0x000000FF 2440 #define CPU_SCS_NVIC_IPR0_PRI_0_S 0 2450 #define CPU_SCS_NVIC_IPR1_PRI_7_W 8 2451 #define CPU_SCS_NVIC_IPR1_PRI_7_M 0xFF000000 2452 #define CPU_SCS_NVIC_IPR1_PRI_7_S 24 2457 #define CPU_SCS_NVIC_IPR1_PRI_6_W 8 2458 #define CPU_SCS_NVIC_IPR1_PRI_6_M 0x00FF0000 2459 #define CPU_SCS_NVIC_IPR1_PRI_6_S 16 2464 #define CPU_SCS_NVIC_IPR1_PRI_5_W 8 2465 #define CPU_SCS_NVIC_IPR1_PRI_5_M 0x0000FF00 2466 #define CPU_SCS_NVIC_IPR1_PRI_5_S 8 2471 #define CPU_SCS_NVIC_IPR1_PRI_4_W 8 2472 #define CPU_SCS_NVIC_IPR1_PRI_4_M 0x000000FF 2473 #define CPU_SCS_NVIC_IPR1_PRI_4_S 0 2483 #define CPU_SCS_NVIC_IPR2_PRI_11_W 8 2484 #define CPU_SCS_NVIC_IPR2_PRI_11_M 0xFF000000 2485 #define CPU_SCS_NVIC_IPR2_PRI_11_S 24 2490 #define CPU_SCS_NVIC_IPR2_PRI_10_W 8 2491 #define CPU_SCS_NVIC_IPR2_PRI_10_M 0x00FF0000 2492 #define CPU_SCS_NVIC_IPR2_PRI_10_S 16 2497 #define CPU_SCS_NVIC_IPR2_PRI_9_W 8 2498 #define CPU_SCS_NVIC_IPR2_PRI_9_M 0x0000FF00 2499 #define CPU_SCS_NVIC_IPR2_PRI_9_S 8 2504 #define CPU_SCS_NVIC_IPR2_PRI_8_W 8 2505 #define CPU_SCS_NVIC_IPR2_PRI_8_M 0x000000FF 2506 #define CPU_SCS_NVIC_IPR2_PRI_8_S 0 2516 #define CPU_SCS_NVIC_IPR3_PRI_15_W 8 2517 #define CPU_SCS_NVIC_IPR3_PRI_15_M 0xFF000000 2518 #define CPU_SCS_NVIC_IPR3_PRI_15_S 24 2523 #define CPU_SCS_NVIC_IPR3_PRI_14_W 8 2524 #define CPU_SCS_NVIC_IPR3_PRI_14_M 0x00FF0000 2525 #define CPU_SCS_NVIC_IPR3_PRI_14_S 16 2530 #define CPU_SCS_NVIC_IPR3_PRI_13_W 8 2531 #define CPU_SCS_NVIC_IPR3_PRI_13_M 0x0000FF00 2532 #define CPU_SCS_NVIC_IPR3_PRI_13_S 8 2537 #define CPU_SCS_NVIC_IPR3_PRI_12_W 8 2538 #define CPU_SCS_NVIC_IPR3_PRI_12_M 0x000000FF 2539 #define CPU_SCS_NVIC_IPR3_PRI_12_S 0 2549 #define CPU_SCS_NVIC_IPR4_PRI_19_W 8 2550 #define CPU_SCS_NVIC_IPR4_PRI_19_M 0xFF000000 2551 #define CPU_SCS_NVIC_IPR4_PRI_19_S 24 2556 #define CPU_SCS_NVIC_IPR4_PRI_18_W 8 2557 #define CPU_SCS_NVIC_IPR4_PRI_18_M 0x00FF0000 2558 #define CPU_SCS_NVIC_IPR4_PRI_18_S 16 2563 #define CPU_SCS_NVIC_IPR4_PRI_17_W 8 2564 #define CPU_SCS_NVIC_IPR4_PRI_17_M 0x0000FF00 2565 #define CPU_SCS_NVIC_IPR4_PRI_17_S 8 2570 #define CPU_SCS_NVIC_IPR4_PRI_16_W 8 2571 #define CPU_SCS_NVIC_IPR4_PRI_16_M 0x000000FF 2572 #define CPU_SCS_NVIC_IPR4_PRI_16_S 0 2582 #define CPU_SCS_NVIC_IPR5_PRI_23_W 8 2583 #define CPU_SCS_NVIC_IPR5_PRI_23_M 0xFF000000 2584 #define CPU_SCS_NVIC_IPR5_PRI_23_S 24 2589 #define CPU_SCS_NVIC_IPR5_PRI_22_W 8 2590 #define CPU_SCS_NVIC_IPR5_PRI_22_M 0x00FF0000 2591 #define CPU_SCS_NVIC_IPR5_PRI_22_S 16 2596 #define CPU_SCS_NVIC_IPR5_PRI_21_W 8 2597 #define CPU_SCS_NVIC_IPR5_PRI_21_M 0x0000FF00 2598 #define CPU_SCS_NVIC_IPR5_PRI_21_S 8 2603 #define CPU_SCS_NVIC_IPR5_PRI_20_W 8 2604 #define CPU_SCS_NVIC_IPR5_PRI_20_M 0x000000FF 2605 #define CPU_SCS_NVIC_IPR5_PRI_20_S 0 2615 #define CPU_SCS_NVIC_IPR6_PRI_27_W 8 2616 #define CPU_SCS_NVIC_IPR6_PRI_27_M 0xFF000000 2617 #define CPU_SCS_NVIC_IPR6_PRI_27_S 24 2622 #define CPU_SCS_NVIC_IPR6_PRI_26_W 8 2623 #define CPU_SCS_NVIC_IPR6_PRI_26_M 0x00FF0000 2624 #define CPU_SCS_NVIC_IPR6_PRI_26_S 16 2629 #define CPU_SCS_NVIC_IPR6_PRI_25_W 8 2630 #define CPU_SCS_NVIC_IPR6_PRI_25_M 0x0000FF00 2631 #define CPU_SCS_NVIC_IPR6_PRI_25_S 8 2636 #define CPU_SCS_NVIC_IPR6_PRI_24_W 8 2637 #define CPU_SCS_NVIC_IPR6_PRI_24_M 0x000000FF 2638 #define CPU_SCS_NVIC_IPR6_PRI_24_S 0 2648 #define CPU_SCS_NVIC_IPR7_PRI_31_W 8 2649 #define CPU_SCS_NVIC_IPR7_PRI_31_M 0xFF000000 2650 #define CPU_SCS_NVIC_IPR7_PRI_31_S 24 2655 #define CPU_SCS_NVIC_IPR7_PRI_30_W 8 2656 #define CPU_SCS_NVIC_IPR7_PRI_30_M 0x00FF0000 2657 #define CPU_SCS_NVIC_IPR7_PRI_30_S 16 2662 #define CPU_SCS_NVIC_IPR7_PRI_29_W 8 2663 #define CPU_SCS_NVIC_IPR7_PRI_29_M 0x0000FF00 2664 #define CPU_SCS_NVIC_IPR7_PRI_29_S 8 2669 #define CPU_SCS_NVIC_IPR7_PRI_28_W 8 2670 #define CPU_SCS_NVIC_IPR7_PRI_28_M 0x000000FF 2671 #define CPU_SCS_NVIC_IPR7_PRI_28_S 0 2681 #define CPU_SCS_NVIC_IPR8_PRI_35_W 8 2682 #define CPU_SCS_NVIC_IPR8_PRI_35_M 0xFF000000 2683 #define CPU_SCS_NVIC_IPR8_PRI_35_S 24 2688 #define CPU_SCS_NVIC_IPR8_PRI_34_W 8 2689 #define CPU_SCS_NVIC_IPR8_PRI_34_M 0x00FF0000 2690 #define CPU_SCS_NVIC_IPR8_PRI_34_S 16 2695 #define CPU_SCS_NVIC_IPR8_PRI_33_W 8 2696 #define CPU_SCS_NVIC_IPR8_PRI_33_M 0x0000FF00 2697 #define CPU_SCS_NVIC_IPR8_PRI_33_S 8 2702 #define CPU_SCS_NVIC_IPR8_PRI_32_W 8 2703 #define CPU_SCS_NVIC_IPR8_PRI_32_M 0x000000FF 2704 #define CPU_SCS_NVIC_IPR8_PRI_32_S 0 2714 #define CPU_SCS_NVIC_IPR9_PRI_37_W 8 2715 #define CPU_SCS_NVIC_IPR9_PRI_37_M 0x0000FF00 2716 #define CPU_SCS_NVIC_IPR9_PRI_37_S 8 2721 #define CPU_SCS_NVIC_IPR9_PRI_36_W 8 2722 #define CPU_SCS_NVIC_IPR9_PRI_36_M 0x000000FF 2723 #define CPU_SCS_NVIC_IPR9_PRI_36_S 0 2733 #define CPU_SCS_CPUID_IMPLEMENTER_W 8 2734 #define CPU_SCS_CPUID_IMPLEMENTER_M 0xFF000000 2735 #define CPU_SCS_CPUID_IMPLEMENTER_S 24 2740 #define CPU_SCS_CPUID_VARIANT_W 4 2741 #define CPU_SCS_CPUID_VARIANT_M 0x00F00000 2742 #define CPU_SCS_CPUID_VARIANT_S 20 2747 #define CPU_SCS_CPUID_CONSTANT_W 4 2748 #define CPU_SCS_CPUID_CONSTANT_M 0x000F0000 2749 #define CPU_SCS_CPUID_CONSTANT_S 16 2754 #define CPU_SCS_CPUID_PARTNO_W 12 2755 #define CPU_SCS_CPUID_PARTNO_M 0x0000FFF0 2756 #define CPU_SCS_CPUID_PARTNO_S 4 2761 #define CPU_SCS_CPUID_REVISION_W 4 2762 #define CPU_SCS_CPUID_REVISION_M 0x0000000F 2763 #define CPU_SCS_CPUID_REVISION_S 0 2778 #define CPU_SCS_ICSR_NMIPENDSET 0x80000000 2779 #define CPU_SCS_ICSR_NMIPENDSET_BITN 31 2780 #define CPU_SCS_ICSR_NMIPENDSET_M 0x80000000 2781 #define CPU_SCS_ICSR_NMIPENDSET_S 31 2789 #define CPU_SCS_ICSR_PENDSVSET 0x10000000 2790 #define CPU_SCS_ICSR_PENDSVSET_BITN 28 2791 #define CPU_SCS_ICSR_PENDSVSET_M 0x10000000 2792 #define CPU_SCS_ICSR_PENDSVSET_S 28 2800 #define CPU_SCS_ICSR_PENDSVCLR 0x08000000 2801 #define CPU_SCS_ICSR_PENDSVCLR_BITN 27 2802 #define CPU_SCS_ICSR_PENDSVCLR_M 0x08000000 2803 #define CPU_SCS_ICSR_PENDSVCLR_S 27 2811 #define CPU_SCS_ICSR_PENDSTSET 0x04000000 2812 #define CPU_SCS_ICSR_PENDSTSET_BITN 26 2813 #define CPU_SCS_ICSR_PENDSTSET_M 0x04000000 2814 #define CPU_SCS_ICSR_PENDSTSET_S 26 2822 #define CPU_SCS_ICSR_PENDSTCLR 0x02000000 2823 #define CPU_SCS_ICSR_PENDSTCLR_BITN 25 2824 #define CPU_SCS_ICSR_PENDSTCLR_M 0x02000000 2825 #define CPU_SCS_ICSR_PENDSTCLR_S 25 2835 #define CPU_SCS_ICSR_ISRPREEMPT 0x00800000 2836 #define CPU_SCS_ICSR_ISRPREEMPT_BITN 23 2837 #define CPU_SCS_ICSR_ISRPREEMPT_M 0x00800000 2838 #define CPU_SCS_ICSR_ISRPREEMPT_S 23 2846 #define CPU_SCS_ICSR_ISRPENDING 0x00400000 2847 #define CPU_SCS_ICSR_ISRPENDING_BITN 22 2848 #define CPU_SCS_ICSR_ISRPENDING_M 0x00400000 2849 #define CPU_SCS_ICSR_ISRPENDING_S 22 2855 #define CPU_SCS_ICSR_VECTPENDING_W 6 2856 #define CPU_SCS_ICSR_VECTPENDING_M 0x0003F000 2857 #define CPU_SCS_ICSR_VECTPENDING_S 12 2866 #define CPU_SCS_ICSR_RETTOBASE 0x00000800 2867 #define CPU_SCS_ICSR_RETTOBASE_BITN 11 2868 #define CPU_SCS_ICSR_RETTOBASE_M 0x00000800 2869 #define CPU_SCS_ICSR_RETTOBASE_S 11 2874 #define CPU_SCS_ICSR_VECTACTIVE_W 9 2875 #define CPU_SCS_ICSR_VECTACTIVE_M 0x000001FF 2876 #define CPU_SCS_ICSR_VECTACTIVE_S 0 2886 #define CPU_SCS_VTOR_TBLOFF_W 23 2887 #define CPU_SCS_VTOR_TBLOFF_M 0x3FFFFF80 2888 #define CPU_SCS_VTOR_TBLOFF_S 7 2899 #define CPU_SCS_AIRCR_VECTKEY_W 16 2900 #define CPU_SCS_AIRCR_VECTKEY_M 0xFFFF0000 2901 #define CPU_SCS_AIRCR_VECTKEY_S 16 2909 #define CPU_SCS_AIRCR_ENDIANESS 0x00008000 2910 #define CPU_SCS_AIRCR_ENDIANESS_BITN 15 2911 #define CPU_SCS_AIRCR_ENDIANESS_M 0x00008000 2912 #define CPU_SCS_AIRCR_ENDIANESS_S 15 2913 #define CPU_SCS_AIRCR_ENDIANESS_BIG 0x00008000 2914 #define CPU_SCS_AIRCR_ENDIANESS_LITTLE 0x00000000 2926 #define CPU_SCS_AIRCR_PRIGROUP_W 3 2927 #define CPU_SCS_AIRCR_PRIGROUP_M 0x00000700 2928 #define CPU_SCS_AIRCR_PRIGROUP_S 8 2934 #define CPU_SCS_AIRCR_SYSRESETREQ 0x00000004 2935 #define CPU_SCS_AIRCR_SYSRESETREQ_BITN 2 2936 #define CPU_SCS_AIRCR_SYSRESETREQ_M 0x00000004 2937 #define CPU_SCS_AIRCR_SYSRESETREQ_S 2 2947 #define CPU_SCS_AIRCR_VECTCLRACTIVE 0x00000002 2948 #define CPU_SCS_AIRCR_VECTCLRACTIVE_BITN 1 2949 #define CPU_SCS_AIRCR_VECTCLRACTIVE_M 0x00000002 2950 #define CPU_SCS_AIRCR_VECTCLRACTIVE_S 1 2958 #define CPU_SCS_AIRCR_VECTRESET 0x00000001 2959 #define CPU_SCS_AIRCR_VECTRESET_BITN 0 2960 #define CPU_SCS_AIRCR_VECTRESET_M 0x00000001 2961 #define CPU_SCS_AIRCR_VECTRESET_S 0 2982 #define CPU_SCS_SCR_SEVONPEND 0x00000010 2983 #define CPU_SCS_SCR_SEVONPEND_BITN 4 2984 #define CPU_SCS_SCR_SEVONPEND_M 0x00000010 2985 #define CPU_SCS_SCR_SEVONPEND_S 4 2994 #define CPU_SCS_SCR_SLEEPDEEP 0x00000004 2995 #define CPU_SCS_SCR_SLEEPDEEP_BITN 2 2996 #define CPU_SCS_SCR_SLEEPDEEP_M 0x00000004 2997 #define CPU_SCS_SCR_SLEEPDEEP_S 2 2998 #define CPU_SCS_SCR_SLEEPDEEP_DEEPSLEEP 0x00000004 2999 #define CPU_SCS_SCR_SLEEPDEEP_SLEEP 0x00000000 3008 #define CPU_SCS_SCR_SLEEPONEXIT 0x00000002 3009 #define CPU_SCS_SCR_SLEEPONEXIT_BITN 1 3010 #define CPU_SCS_SCR_SLEEPONEXIT_M 0x00000002 3011 #define CPU_SCS_SCR_SLEEPONEXIT_S 1 3027 #define CPU_SCS_CCR_STKALIGN 0x00000200 3028 #define CPU_SCS_CCR_STKALIGN_BITN 9 3029 #define CPU_SCS_CCR_STKALIGN_M 0x00000200 3030 #define CPU_SCS_CCR_STKALIGN_S 9 3044 #define CPU_SCS_CCR_BFHFNMIGN 0x00000100 3045 #define CPU_SCS_CCR_BFHFNMIGN_BITN 8 3046 #define CPU_SCS_CCR_BFHFNMIGN_M 0x00000100 3047 #define CPU_SCS_CCR_BFHFNMIGN_S 8 3058 #define CPU_SCS_CCR_DIV_0_TRP 0x00000010 3059 #define CPU_SCS_CCR_DIV_0_TRP_BITN 4 3060 #define CPU_SCS_CCR_DIV_0_TRP_M 0x00000010 3061 #define CPU_SCS_CCR_DIV_0_TRP_S 4 3074 #define CPU_SCS_CCR_UNALIGN_TRP 0x00000008 3075 #define CPU_SCS_CCR_UNALIGN_TRP_BITN 3 3076 #define CPU_SCS_CCR_UNALIGN_TRP_M 0x00000008 3077 #define CPU_SCS_CCR_UNALIGN_TRP_S 3 3088 #define CPU_SCS_CCR_USERSETMPEND 0x00000002 3089 #define CPU_SCS_CCR_USERSETMPEND_BITN 1 3090 #define CPU_SCS_CCR_USERSETMPEND_M 0x00000002 3091 #define CPU_SCS_CCR_USERSETMPEND_S 1 3108 #define CPU_SCS_CCR_NONBASETHREDENA 0x00000001 3109 #define CPU_SCS_CCR_NONBASETHREDENA_BITN 0 3110 #define CPU_SCS_CCR_NONBASETHREDENA_M 0x00000001 3111 #define CPU_SCS_CCR_NONBASETHREDENA_S 0 3121 #define CPU_SCS_SHPR1_PRI_6_W 8 3122 #define CPU_SCS_SHPR1_PRI_6_M 0x00FF0000 3123 #define CPU_SCS_SHPR1_PRI_6_S 16 3128 #define CPU_SCS_SHPR1_PRI_5_W 8 3129 #define CPU_SCS_SHPR1_PRI_5_M 0x0000FF00 3130 #define CPU_SCS_SHPR1_PRI_5_S 8 3135 #define CPU_SCS_SHPR1_PRI_4_W 8 3136 #define CPU_SCS_SHPR1_PRI_4_M 0x000000FF 3137 #define CPU_SCS_SHPR1_PRI_4_S 0 3147 #define CPU_SCS_SHPR2_PRI_11_W 8 3148 #define CPU_SCS_SHPR2_PRI_11_M 0xFF000000 3149 #define CPU_SCS_SHPR2_PRI_11_S 24 3159 #define CPU_SCS_SHPR3_PRI_15_W 8 3160 #define CPU_SCS_SHPR3_PRI_15_M 0xFF000000 3161 #define CPU_SCS_SHPR3_PRI_15_S 24 3166 #define CPU_SCS_SHPR3_PRI_14_W 8 3167 #define CPU_SCS_SHPR3_PRI_14_M 0x00FF0000 3168 #define CPU_SCS_SHPR3_PRI_14_S 16 3173 #define CPU_SCS_SHPR3_PRI_12_W 8 3174 #define CPU_SCS_SHPR3_PRI_12_M 0x000000FF 3175 #define CPU_SCS_SHPR3_PRI_12_S 0 3188 #define CPU_SCS_SHCSR_USGFAULTENA 0x00040000 3189 #define CPU_SCS_SHCSR_USGFAULTENA_BITN 18 3190 #define CPU_SCS_SHCSR_USGFAULTENA_M 0x00040000 3191 #define CPU_SCS_SHCSR_USGFAULTENA_S 18 3192 #define CPU_SCS_SHCSR_USGFAULTENA_EN 0x00040000 3193 #define CPU_SCS_SHCSR_USGFAULTENA_DIS 0x00000000 3201 #define CPU_SCS_SHCSR_BUSFAULTENA 0x00020000 3202 #define CPU_SCS_SHCSR_BUSFAULTENA_BITN 17 3203 #define CPU_SCS_SHCSR_BUSFAULTENA_M 0x00020000 3204 #define CPU_SCS_SHCSR_BUSFAULTENA_S 17 3205 #define CPU_SCS_SHCSR_BUSFAULTENA_EN 0x00020000 3206 #define CPU_SCS_SHCSR_BUSFAULTENA_DIS 0x00000000 3214 #define CPU_SCS_SHCSR_MEMFAULTENA 0x00010000 3215 #define CPU_SCS_SHCSR_MEMFAULTENA_BITN 16 3216 #define CPU_SCS_SHCSR_MEMFAULTENA_M 0x00010000 3217 #define CPU_SCS_SHCSR_MEMFAULTENA_S 16 3218 #define CPU_SCS_SHCSR_MEMFAULTENA_EN 0x00010000 3219 #define CPU_SCS_SHCSR_MEMFAULTENA_DIS 0x00000000 3227 #define CPU_SCS_SHCSR_SVCALLPENDED 0x00008000 3228 #define CPU_SCS_SHCSR_SVCALLPENDED_BITN 15 3229 #define CPU_SCS_SHCSR_SVCALLPENDED_M 0x00008000 3230 #define CPU_SCS_SHCSR_SVCALLPENDED_S 15 3231 #define CPU_SCS_SHCSR_SVCALLPENDED_PENDING 0x00008000 3232 #define CPU_SCS_SHCSR_SVCALLPENDED_NOTPENDING 0x00000000 3240 #define CPU_SCS_SHCSR_BUSFAULTPENDED 0x00004000 3241 #define CPU_SCS_SHCSR_BUSFAULTPENDED_BITN 14 3242 #define CPU_SCS_SHCSR_BUSFAULTPENDED_M 0x00004000 3243 #define CPU_SCS_SHCSR_BUSFAULTPENDED_S 14 3244 #define CPU_SCS_SHCSR_BUSFAULTPENDED_PENDING 0x00004000 3245 #define CPU_SCS_SHCSR_BUSFAULTPENDED_NOTPENDING 0x00000000 3253 #define CPU_SCS_SHCSR_MEMFAULTPENDED 0x00002000 3254 #define CPU_SCS_SHCSR_MEMFAULTPENDED_BITN 13 3255 #define CPU_SCS_SHCSR_MEMFAULTPENDED_M 0x00002000 3256 #define CPU_SCS_SHCSR_MEMFAULTPENDED_S 13 3257 #define CPU_SCS_SHCSR_MEMFAULTPENDED_PENDING 0x00002000 3258 #define CPU_SCS_SHCSR_MEMFAULTPENDED_NOTPENDING 0x00000000 3266 #define CPU_SCS_SHCSR_USGFAULTPENDED 0x00001000 3267 #define CPU_SCS_SHCSR_USGFAULTPENDED_BITN 12 3268 #define CPU_SCS_SHCSR_USGFAULTPENDED_M 0x00001000 3269 #define CPU_SCS_SHCSR_USGFAULTPENDED_S 12 3270 #define CPU_SCS_SHCSR_USGFAULTPENDED_PENDING 0x00001000 3271 #define CPU_SCS_SHCSR_USGFAULTPENDED_NOTPENDING 0x00000000 3282 #define CPU_SCS_SHCSR_SYSTICKACT 0x00000800 3283 #define CPU_SCS_SHCSR_SYSTICKACT_BITN 11 3284 #define CPU_SCS_SHCSR_SYSTICKACT_M 0x00000800 3285 #define CPU_SCS_SHCSR_SYSTICKACT_S 11 3286 #define CPU_SCS_SHCSR_SYSTICKACT_ACTIVE 0x00000800 3287 #define CPU_SCS_SHCSR_SYSTICKACT_NOTACTIVE 0x00000000 3295 #define CPU_SCS_SHCSR_PENDSVACT 0x00000400 3296 #define CPU_SCS_SHCSR_PENDSVACT_BITN 10 3297 #define CPU_SCS_SHCSR_PENDSVACT_M 0x00000400 3298 #define CPU_SCS_SHCSR_PENDSVACT_S 10 3306 #define CPU_SCS_SHCSR_MONITORACT 0x00000100 3307 #define CPU_SCS_SHCSR_MONITORACT_BITN 8 3308 #define CPU_SCS_SHCSR_MONITORACT_M 0x00000100 3309 #define CPU_SCS_SHCSR_MONITORACT_S 8 3310 #define CPU_SCS_SHCSR_MONITORACT_ACTIVE 0x00000100 3311 #define CPU_SCS_SHCSR_MONITORACT_NOTACTIVE 0x00000000 3319 #define CPU_SCS_SHCSR_SVCALLACT 0x00000080 3320 #define CPU_SCS_SHCSR_SVCALLACT_BITN 7 3321 #define CPU_SCS_SHCSR_SVCALLACT_M 0x00000080 3322 #define CPU_SCS_SHCSR_SVCALLACT_S 7 3323 #define CPU_SCS_SHCSR_SVCALLACT_ACTIVE 0x00000080 3324 #define CPU_SCS_SHCSR_SVCALLACT_NOTACTIVE 0x00000000 3332 #define CPU_SCS_SHCSR_USGFAULTACT 0x00000008 3333 #define CPU_SCS_SHCSR_USGFAULTACT_BITN 3 3334 #define CPU_SCS_SHCSR_USGFAULTACT_M 0x00000008 3335 #define CPU_SCS_SHCSR_USGFAULTACT_S 3 3336 #define CPU_SCS_SHCSR_USGFAULTACT_ACTIVE 0x00000008 3337 #define CPU_SCS_SHCSR_USGFAULTACT_NOTACTIVE 0x00000000 3345 #define CPU_SCS_SHCSR_BUSFAULTACT 0x00000002 3346 #define CPU_SCS_SHCSR_BUSFAULTACT_BITN 1 3347 #define CPU_SCS_SHCSR_BUSFAULTACT_M 0x00000002 3348 #define CPU_SCS_SHCSR_BUSFAULTACT_S 1 3349 #define CPU_SCS_SHCSR_BUSFAULTACT_ACTIVE 0x00000002 3350 #define CPU_SCS_SHCSR_BUSFAULTACT_NOTACTIVE 0x00000000 3358 #define CPU_SCS_SHCSR_MEMFAULTACT 0x00000001 3359 #define CPU_SCS_SHCSR_MEMFAULTACT_BITN 0 3360 #define CPU_SCS_SHCSR_MEMFAULTACT_M 0x00000001 3361 #define CPU_SCS_SHCSR_MEMFAULTACT_S 0 3362 #define CPU_SCS_SHCSR_MEMFAULTACT_ACTIVE 0x00000001 3363 #define CPU_SCS_SHCSR_MEMFAULTACT_NOTACTIVE 0x00000000 3376 #define CPU_SCS_CFSR_DIVBYZERO 0x02000000 3377 #define CPU_SCS_CFSR_DIVBYZERO_BITN 25 3378 #define CPU_SCS_CFSR_DIVBYZERO_M 0x02000000 3379 #define CPU_SCS_CFSR_DIVBYZERO_S 25 3386 #define CPU_SCS_CFSR_UNALIGNED 0x01000000 3387 #define CPU_SCS_CFSR_UNALIGNED_BITN 24 3388 #define CPU_SCS_CFSR_UNALIGNED_M 0x01000000 3389 #define CPU_SCS_CFSR_UNALIGNED_S 24 3395 #define CPU_SCS_CFSR_NOCP 0x00080000 3396 #define CPU_SCS_CFSR_NOCP_BITN 19 3397 #define CPU_SCS_CFSR_NOCP_M 0x00080000 3398 #define CPU_SCS_CFSR_NOCP_S 19 3405 #define CPU_SCS_CFSR_INVPC 0x00040000 3406 #define CPU_SCS_CFSR_INVPC_BITN 18 3407 #define CPU_SCS_CFSR_INVPC_M 0x00040000 3408 #define CPU_SCS_CFSR_INVPC_S 18 3416 #define CPU_SCS_CFSR_INVSTATE 0x00020000 3417 #define CPU_SCS_CFSR_INVSTATE_BITN 17 3418 #define CPU_SCS_CFSR_INVSTATE_M 0x00020000 3419 #define CPU_SCS_CFSR_INVSTATE_S 17 3426 #define CPU_SCS_CFSR_UNDEFINSTR 0x00010000 3427 #define CPU_SCS_CFSR_UNDEFINSTR_BITN 16 3428 #define CPU_SCS_CFSR_UNDEFINSTR_M 0x00010000 3429 #define CPU_SCS_CFSR_UNDEFINSTR_S 16 3439 #define CPU_SCS_CFSR_BFARVALID 0x00008000 3440 #define CPU_SCS_CFSR_BFARVALID_BITN 15 3441 #define CPU_SCS_CFSR_BFARVALID_M 0x00008000 3442 #define CPU_SCS_CFSR_BFARVALID_S 15 3449 #define CPU_SCS_CFSR_STKERR 0x00001000 3450 #define CPU_SCS_CFSR_STKERR_BITN 12 3451 #define CPU_SCS_CFSR_STKERR_M 0x00001000 3452 #define CPU_SCS_CFSR_STKERR_S 12 3460 #define CPU_SCS_CFSR_UNSTKERR 0x00000800 3461 #define CPU_SCS_CFSR_UNSTKERR_BITN 11 3462 #define CPU_SCS_CFSR_UNSTKERR_M 0x00000800 3463 #define CPU_SCS_CFSR_UNSTKERR_S 11 3474 #define CPU_SCS_CFSR_IMPRECISERR 0x00000400 3475 #define CPU_SCS_CFSR_IMPRECISERR_BITN 10 3476 #define CPU_SCS_CFSR_IMPRECISERR_M 0x00000400 3477 #define CPU_SCS_CFSR_IMPRECISERR_S 10 3482 #define CPU_SCS_CFSR_PRECISERR 0x00000200 3483 #define CPU_SCS_CFSR_PRECISERR_BITN 9 3484 #define CPU_SCS_CFSR_PRECISERR_M 0x00000200 3485 #define CPU_SCS_CFSR_PRECISERR_S 9 3492 #define CPU_SCS_CFSR_IBUSERR 0x00000100 3493 #define CPU_SCS_CFSR_IBUSERR_BITN 8 3494 #define CPU_SCS_CFSR_IBUSERR_M 0x00000100 3495 #define CPU_SCS_CFSR_IBUSERR_S 8 3504 #define CPU_SCS_CFSR_MMARVALID 0x00000080 3505 #define CPU_SCS_CFSR_MMARVALID_BITN 7 3506 #define CPU_SCS_CFSR_MMARVALID_M 0x00000080 3507 #define CPU_SCS_CFSR_MMARVALID_S 7 3514 #define CPU_SCS_CFSR_MSTKERR 0x00000010 3515 #define CPU_SCS_CFSR_MSTKERR_BITN 4 3516 #define CPU_SCS_CFSR_MSTKERR_M 0x00000010 3517 #define CPU_SCS_CFSR_MSTKERR_S 4 3525 #define CPU_SCS_CFSR_MUNSTKERR 0x00000008 3526 #define CPU_SCS_CFSR_MUNSTKERR_BITN 3 3527 #define CPU_SCS_CFSR_MUNSTKERR_M 0x00000008 3528 #define CPU_SCS_CFSR_MUNSTKERR_S 3 3536 #define CPU_SCS_CFSR_DACCVIOL 0x00000002 3537 #define CPU_SCS_CFSR_DACCVIOL_BITN 1 3538 #define CPU_SCS_CFSR_DACCVIOL_M 0x00000002 3539 #define CPU_SCS_CFSR_DACCVIOL_S 1 3547 #define CPU_SCS_CFSR_IACCVIOL 0x00000001 3548 #define CPU_SCS_CFSR_IACCVIOL_BITN 0 3549 #define CPU_SCS_CFSR_IACCVIOL_M 0x00000001 3550 #define CPU_SCS_CFSR_IACCVIOL_S 0 3565 #define CPU_SCS_HFSR_DEBUGEVT 0x80000000 3566 #define CPU_SCS_HFSR_DEBUGEVT_BITN 31 3567 #define CPU_SCS_HFSR_DEBUGEVT_M 0x80000000 3568 #define CPU_SCS_HFSR_DEBUGEVT_S 31 3576 #define CPU_SCS_HFSR_FORCED 0x40000000 3577 #define CPU_SCS_HFSR_FORCED_BITN 30 3578 #define CPU_SCS_HFSR_FORCED_M 0x40000000 3579 #define CPU_SCS_HFSR_FORCED_S 30 3586 #define CPU_SCS_HFSR_VECTTBL 0x00000002 3587 #define CPU_SCS_HFSR_VECTTBL_BITN 1 3588 #define CPU_SCS_HFSR_VECTTBL_M 0x00000002 3589 #define CPU_SCS_HFSR_VECTTBL_S 1 3603 #define CPU_SCS_DFSR_EXTERNAL 0x00000010 3604 #define CPU_SCS_DFSR_EXTERNAL_BITN 4 3605 #define CPU_SCS_DFSR_EXTERNAL_M 0x00000010 3606 #define CPU_SCS_DFSR_EXTERNAL_S 4 3615 #define CPU_SCS_DFSR_VCATCH 0x00000008 3616 #define CPU_SCS_DFSR_VCATCH_BITN 3 3617 #define CPU_SCS_DFSR_VCATCH_M 0x00000008 3618 #define CPU_SCS_DFSR_VCATCH_S 3 3627 #define CPU_SCS_DFSR_DWTTRAP 0x00000004 3628 #define CPU_SCS_DFSR_DWTTRAP_BITN 2 3629 #define CPU_SCS_DFSR_DWTTRAP_M 0x00000004 3630 #define CPU_SCS_DFSR_DWTTRAP_S 2 3640 #define CPU_SCS_DFSR_BKPT 0x00000002 3641 #define CPU_SCS_DFSR_BKPT_BITN 1 3642 #define CPU_SCS_DFSR_BKPT_M 0x00000002 3643 #define CPU_SCS_DFSR_BKPT_S 1 3651 #define CPU_SCS_DFSR_HALTED 0x00000001 3652 #define CPU_SCS_DFSR_HALTED_BITN 0 3653 #define CPU_SCS_DFSR_HALTED_M 0x00000001 3654 #define CPU_SCS_DFSR_HALTED_S 0 3670 #define CPU_SCS_MMFAR_ADDRESS_W 32 3671 #define CPU_SCS_MMFAR_ADDRESS_M 0xFFFFFFFF 3672 #define CPU_SCS_MMFAR_ADDRESS_S 0 3687 #define CPU_SCS_BFAR_ADDRESS_W 32 3688 #define CPU_SCS_BFAR_ADDRESS_M 0xFFFFFFFF 3689 #define CPU_SCS_BFAR_ADDRESS_S 0 3700 #define CPU_SCS_AFSR_IMPDEF_W 32 3701 #define CPU_SCS_AFSR_IMPDEF_M 0xFFFFFFFF 3702 #define CPU_SCS_AFSR_IMPDEF_S 0 3720 #define CPU_SCS_ID_PFR0_STATE1_W 4 3721 #define CPU_SCS_ID_PFR0_STATE1_M 0x000000F0 3722 #define CPU_SCS_ID_PFR0_STATE1_S 4 3730 #define CPU_SCS_ID_PFR0_STATE0_W 4 3731 #define CPU_SCS_ID_PFR0_STATE0_M 0x0000000F 3732 #define CPU_SCS_ID_PFR0_STATE0_S 0 3745 #define CPU_SCS_ID_PFR1_MICROCONTROLLER_PROGRAMMERS_MODEL_W 4 3746 #define CPU_SCS_ID_PFR1_MICROCONTROLLER_PROGRAMMERS_MODEL_M 0x00000F00 3747 #define CPU_SCS_ID_PFR1_MICROCONTROLLER_PROGRAMMERS_MODEL_S 8 3760 #define CPU_SCS_ID_DFR0_MICROCONTROLLER_DEBUG_MODEL_W 4 3761 #define CPU_SCS_ID_DFR0_MICROCONTROLLER_DEBUG_MODEL_M 0x00F00000 3762 #define CPU_SCS_ID_DFR0_MICROCONTROLLER_DEBUG_MODEL_S 20 3790 #define CPU_SCS_ID_MMFR2_WAIT_FOR_INTERRUPT_STALLING 0x01000000 3791 #define CPU_SCS_ID_MMFR2_WAIT_FOR_INTERRUPT_STALLING_BITN 24 3792 #define CPU_SCS_ID_MMFR2_WAIT_FOR_INTERRUPT_STALLING_M 0x01000000 3793 #define CPU_SCS_ID_MMFR2_WAIT_FOR_INTERRUPT_STALLING_S 24 3838 #define CPU_SCS_MPU_TYPE_IREGION_W 8 3839 #define CPU_SCS_MPU_TYPE_IREGION_M 0x00FF0000 3840 #define CPU_SCS_MPU_TYPE_IREGION_S 16 3846 #define CPU_SCS_MPU_TYPE_DREGION_W 8 3847 #define CPU_SCS_MPU_TYPE_DREGION_M 0x0000FF00 3848 #define CPU_SCS_MPU_TYPE_DREGION_S 8 3853 #define CPU_SCS_MPU_TYPE_SEPARATE 0x00000001 3854 #define CPU_SCS_MPU_TYPE_SEPARATE_BITN 0 3855 #define CPU_SCS_MPU_TYPE_SEPARATE_M 0x00000001 3856 #define CPU_SCS_MPU_TYPE_SEPARATE_S 0 3876 #define CPU_SCS_MPU_CTRL_PRIVDEFENA 0x00000004 3877 #define CPU_SCS_MPU_CTRL_PRIVDEFENA_BITN 2 3878 #define CPU_SCS_MPU_CTRL_PRIVDEFENA_M 0x00000004 3879 #define CPU_SCS_MPU_CTRL_PRIVDEFENA_S 2 3888 #define CPU_SCS_MPU_CTRL_HFNMIENA 0x00000002 3889 #define CPU_SCS_MPU_CTRL_HFNMIENA_BITN 1 3890 #define CPU_SCS_MPU_CTRL_HFNMIENA_M 0x00000002 3891 #define CPU_SCS_MPU_CTRL_HFNMIENA_S 1 3899 #define CPU_SCS_MPU_CTRL_ENABLE 0x00000001 3900 #define CPU_SCS_MPU_CTRL_ENABLE_BITN 0 3901 #define CPU_SCS_MPU_CTRL_ENABLE_M 0x00000001 3902 #define CPU_SCS_MPU_CTRL_ENABLE_S 0 3915 #define CPU_SCS_MPU_RNR_REGION_W 8 3916 #define CPU_SCS_MPU_RNR_REGION_M 0x000000FF 3917 #define CPU_SCS_MPU_RNR_REGION_S 0 3931 #define CPU_SCS_MPU_RBAR_ADDR_W 27 3932 #define CPU_SCS_MPU_RBAR_ADDR_M 0xFFFFFFE0 3933 #define CPU_SCS_MPU_RBAR_ADDR_S 5 3940 #define CPU_SCS_MPU_RBAR_VALID 0x00000010 3941 #define CPU_SCS_MPU_RBAR_VALID_BITN 4 3942 #define CPU_SCS_MPU_RBAR_VALID_M 0x00000010 3943 #define CPU_SCS_MPU_RBAR_VALID_S 4 3948 #define CPU_SCS_MPU_RBAR_REGION_W 4 3949 #define CPU_SCS_MPU_RBAR_REGION_M 0x0000000F 3950 #define CPU_SCS_MPU_RBAR_REGION_S 0 3962 #define CPU_SCS_MPU_RASR_XN 0x10000000 3963 #define CPU_SCS_MPU_RASR_XN_BITN 28 3964 #define CPU_SCS_MPU_RASR_XN_M 0x10000000 3965 #define CPU_SCS_MPU_RASR_XN_S 28 3978 #define CPU_SCS_MPU_RASR_AP_W 3 3979 #define CPU_SCS_MPU_RASR_AP_M 0x07000000 3980 #define CPU_SCS_MPU_RASR_AP_S 24 3985 #define CPU_SCS_MPU_RASR_TEX_W 3 3986 #define CPU_SCS_MPU_RASR_TEX_M 0x00380000 3987 #define CPU_SCS_MPU_RASR_TEX_S 19 3994 #define CPU_SCS_MPU_RASR_S 0x00040000 3995 #define CPU_SCS_MPU_RASR_S_BITN 18 3996 #define CPU_SCS_MPU_RASR_S_M 0x00040000 3997 #define CPU_SCS_MPU_RASR_S_S 18 4004 #define CPU_SCS_MPU_RASR_C 0x00020000 4005 #define CPU_SCS_MPU_RASR_C_BITN 17 4006 #define CPU_SCS_MPU_RASR_C_M 0x00020000 4007 #define CPU_SCS_MPU_RASR_C_S 17 4014 #define CPU_SCS_MPU_RASR_B 0x00010000 4015 #define CPU_SCS_MPU_RASR_B_BITN 16 4016 #define CPU_SCS_MPU_RASR_B_M 0x00010000 4017 #define CPU_SCS_MPU_RASR_B_S 16 4025 #define CPU_SCS_MPU_RASR_SRD_W 8 4026 #define CPU_SCS_MPU_RASR_SRD_M 0x0000FF00 4027 #define CPU_SCS_MPU_RASR_SRD_S 8 4060 #define CPU_SCS_MPU_RASR_SIZE_W 5 4061 #define CPU_SCS_MPU_RASR_SIZE_M 0x0000003E 4062 #define CPU_SCS_MPU_RASR_SIZE_S 1 4069 #define CPU_SCS_MPU_RASR_ENABLE 0x00000001 4070 #define CPU_SCS_MPU_RASR_ENABLE_BITN 0 4071 #define CPU_SCS_MPU_RASR_ENABLE_M 0x00000001 4072 #define CPU_SCS_MPU_RASR_ENABLE_S 0 4082 #define CPU_SCS_MPU_RBAR_A1_MPU_RBAR_A1_W 32 4083 #define CPU_SCS_MPU_RBAR_A1_MPU_RBAR_A1_M 0xFFFFFFFF 4084 #define CPU_SCS_MPU_RBAR_A1_MPU_RBAR_A1_S 0 4094 #define CPU_SCS_MPU_RASR_A1_MPU_RASR_A1_W 32 4095 #define CPU_SCS_MPU_RASR_A1_MPU_RASR_A1_M 0xFFFFFFFF 4096 #define CPU_SCS_MPU_RASR_A1_MPU_RASR_A1_S 0 4106 #define CPU_SCS_MPU_RBAR_A2_MPU_RBAR_A2_W 32 4107 #define CPU_SCS_MPU_RBAR_A2_MPU_RBAR_A2_M 0xFFFFFFFF 4108 #define CPU_SCS_MPU_RBAR_A2_MPU_RBAR_A2_S 0 4118 #define CPU_SCS_MPU_RASR_A2_MPU_RASR_A2_W 32 4119 #define CPU_SCS_MPU_RASR_A2_MPU_RASR_A2_M 0xFFFFFFFF 4120 #define CPU_SCS_MPU_RASR_A2_MPU_RASR_A2_S 0 4130 #define CPU_SCS_MPU_RBAR_A3_MPU_RBAR_A3_W 32 4131 #define CPU_SCS_MPU_RBAR_A3_MPU_RBAR_A3_M 0xFFFFFFFF 4132 #define CPU_SCS_MPU_RBAR_A3_MPU_RBAR_A3_S 0 4142 #define CPU_SCS_MPU_RASR_A3_MPU_RASR_A3_W 32 4143 #define CPU_SCS_MPU_RASR_A3_MPU_RASR_A3_M 0xFFFFFFFF 4144 #define CPU_SCS_MPU_RASR_A3_MPU_RASR_A3_S 0 4160 #define CPU_SCS_DHCSR_S_RESET_ST 0x02000000 4161 #define CPU_SCS_DHCSR_S_RESET_ST_BITN 25 4162 #define CPU_SCS_DHCSR_S_RESET_ST_M 0x02000000 4163 #define CPU_SCS_DHCSR_S_RESET_ST_S 25 4172 #define CPU_SCS_DHCSR_S_RETIRE_ST 0x01000000 4173 #define CPU_SCS_DHCSR_S_RETIRE_ST_BITN 24 4174 #define CPU_SCS_DHCSR_S_RETIRE_ST_M 0x01000000 4175 #define CPU_SCS_DHCSR_S_RETIRE_ST_S 24 4183 #define CPU_SCS_DHCSR_S_LOCKUP 0x00080000 4184 #define CPU_SCS_DHCSR_S_LOCKUP_BITN 19 4185 #define CPU_SCS_DHCSR_S_LOCKUP_M 0x00080000 4186 #define CPU_SCS_DHCSR_S_LOCKUP_S 19 4194 #define CPU_SCS_DHCSR_S_SLEEP 0x00040000 4195 #define CPU_SCS_DHCSR_S_SLEEP_BITN 18 4196 #define CPU_SCS_DHCSR_S_SLEEP_M 0x00040000 4197 #define CPU_SCS_DHCSR_S_SLEEP_S 18 4204 #define CPU_SCS_DHCSR_S_HALT 0x00020000 4205 #define CPU_SCS_DHCSR_S_HALT_BITN 17 4206 #define CPU_SCS_DHCSR_S_HALT_M 0x00020000 4207 #define CPU_SCS_DHCSR_S_HALT_S 17 4215 #define CPU_SCS_DHCSR_S_REGRDY 0x00010000 4216 #define CPU_SCS_DHCSR_S_REGRDY_BITN 16 4217 #define CPU_SCS_DHCSR_S_REGRDY_M 0x00010000 4218 #define CPU_SCS_DHCSR_S_REGRDY_S 16 4228 #define CPU_SCS_DHCSR_C_SNAPSTALL 0x00000020 4229 #define CPU_SCS_DHCSR_C_SNAPSTALL_BITN 5 4230 #define CPU_SCS_DHCSR_C_SNAPSTALL_M 0x00000020 4231 #define CPU_SCS_DHCSR_C_SNAPSTALL_S 5 4243 #define CPU_SCS_DHCSR_C_MASKINTS 0x00000008 4244 #define CPU_SCS_DHCSR_C_MASKINTS_BITN 3 4245 #define CPU_SCS_DHCSR_C_MASKINTS_M 0x00000008 4246 #define CPU_SCS_DHCSR_C_MASKINTS_S 3 4254 #define CPU_SCS_DHCSR_C_STEP 0x00000004 4255 #define CPU_SCS_DHCSR_C_STEP_BITN 2 4256 #define CPU_SCS_DHCSR_C_STEP_M 0x00000004 4257 #define CPU_SCS_DHCSR_C_STEP_S 2 4263 #define CPU_SCS_DHCSR_C_HALT 0x00000002 4264 #define CPU_SCS_DHCSR_C_HALT_BITN 1 4265 #define CPU_SCS_DHCSR_C_HALT_M 0x00000002 4266 #define CPU_SCS_DHCSR_C_HALT_S 1 4276 #define CPU_SCS_DHCSR_C_DEBUGEN 0x00000001 4277 #define CPU_SCS_DHCSR_C_DEBUGEN_BITN 0 4278 #define CPU_SCS_DHCSR_C_DEBUGEN_M 0x00000001 4279 #define CPU_SCS_DHCSR_C_DEBUGEN_S 0 4290 #define CPU_SCS_DCRSR_REGWNR 0x00010000 4291 #define CPU_SCS_DCRSR_REGWNR_BITN 16 4292 #define CPU_SCS_DCRSR_REGWNR_M 0x00010000 4293 #define CPU_SCS_DCRSR_REGWNR_S 16 4319 #define CPU_SCS_DCRSR_REGSEL_W 5 4320 #define CPU_SCS_DCRSR_REGSEL_M 0x0000001F 4321 #define CPU_SCS_DCRSR_REGSEL_S 0 4338 #define CPU_SCS_DCRDR_DCRDR_W 32 4339 #define CPU_SCS_DCRDR_DCRDR_M 0xFFFFFFFF 4340 #define CPU_SCS_DCRDR_DCRDR_S 0 4353 #define CPU_SCS_DEMCR_TRCENA 0x01000000 4354 #define CPU_SCS_DEMCR_TRCENA_BITN 24 4355 #define CPU_SCS_DEMCR_TRCENA_M 0x01000000 4356 #define CPU_SCS_DEMCR_TRCENA_S 24 4365 #define CPU_SCS_DEMCR_MON_REQ 0x00080000 4366 #define CPU_SCS_DEMCR_MON_REQ_BITN 19 4367 #define CPU_SCS_DEMCR_MON_REQ_M 0x00080000 4368 #define CPU_SCS_DEMCR_MON_REQ_S 19 4376 #define CPU_SCS_DEMCR_MON_STEP 0x00040000 4377 #define CPU_SCS_DEMCR_MON_STEP_BITN 18 4378 #define CPU_SCS_DEMCR_MON_STEP_M 0x00040000 4379 #define CPU_SCS_DEMCR_MON_STEP_S 18 4388 #define CPU_SCS_DEMCR_MON_PEND 0x00020000 4389 #define CPU_SCS_DEMCR_MON_PEND_BITN 17 4390 #define CPU_SCS_DEMCR_MON_PEND_M 0x00020000 4391 #define CPU_SCS_DEMCR_MON_PEND_S 17 4408 #define CPU_SCS_DEMCR_MON_EN 0x00010000 4409 #define CPU_SCS_DEMCR_MON_EN_BITN 16 4410 #define CPU_SCS_DEMCR_MON_EN_M 0x00010000 4411 #define CPU_SCS_DEMCR_MON_EN_S 16 4416 #define CPU_SCS_DEMCR_VC_HARDERR 0x00000400 4417 #define CPU_SCS_DEMCR_VC_HARDERR_BITN 10 4418 #define CPU_SCS_DEMCR_VC_HARDERR_M 0x00000400 4419 #define CPU_SCS_DEMCR_VC_HARDERR_S 10 4425 #define CPU_SCS_DEMCR_VC_INTERR 0x00000200 4426 #define CPU_SCS_DEMCR_VC_INTERR_BITN 9 4427 #define CPU_SCS_DEMCR_VC_INTERR_M 0x00000200 4428 #define CPU_SCS_DEMCR_VC_INTERR_S 9 4433 #define CPU_SCS_DEMCR_VC_BUSERR 0x00000100 4434 #define CPU_SCS_DEMCR_VC_BUSERR_BITN 8 4435 #define CPU_SCS_DEMCR_VC_BUSERR_M 0x00000100 4436 #define CPU_SCS_DEMCR_VC_BUSERR_S 8 4442 #define CPU_SCS_DEMCR_VC_STATERR 0x00000080 4443 #define CPU_SCS_DEMCR_VC_STATERR_BITN 7 4444 #define CPU_SCS_DEMCR_VC_STATERR_M 0x00000080 4445 #define CPU_SCS_DEMCR_VC_STATERR_S 7 4451 #define CPU_SCS_DEMCR_VC_CHKERR 0x00000040 4452 #define CPU_SCS_DEMCR_VC_CHKERR_BITN 6 4453 #define CPU_SCS_DEMCR_VC_CHKERR_M 0x00000040 4454 #define CPU_SCS_DEMCR_VC_CHKERR_S 6 4460 #define CPU_SCS_DEMCR_VC_NOCPERR 0x00000020 4461 #define CPU_SCS_DEMCR_VC_NOCPERR_BITN 5 4462 #define CPU_SCS_DEMCR_VC_NOCPERR_M 0x00000020 4463 #define CPU_SCS_DEMCR_VC_NOCPERR_S 5 4469 #define CPU_SCS_DEMCR_VC_MMERR 0x00000010 4470 #define CPU_SCS_DEMCR_VC_MMERR_BITN 4 4471 #define CPU_SCS_DEMCR_VC_MMERR_M 0x00000010 4472 #define CPU_SCS_DEMCR_VC_MMERR_S 4 4478 #define CPU_SCS_DEMCR_VC_CORERESET 0x00000001 4479 #define CPU_SCS_DEMCR_VC_CORERESET_BITN 0 4480 #define CPU_SCS_DEMCR_VC_CORERESET_M 0x00000001 4481 #define CPU_SCS_DEMCR_VC_CORERESET_S 0 4493 #define CPU_SCS_STIR_INTID_W 9 4494 #define CPU_SCS_STIR_INTID_M 0x000001FF 4495 #define CPU_SCS_STIR_INTID_S 0 4509 #define CPU_SCS_FPCCR_ASPEN 0x80000000 4510 #define CPU_SCS_FPCCR_ASPEN_BITN 31 4511 #define CPU_SCS_FPCCR_ASPEN_M 0x80000000 4512 #define CPU_SCS_FPCCR_ASPEN_S 31 4522 #define CPU_SCS_FPCCR_LSPEN 0x40000000 4523 #define CPU_SCS_FPCCR_LSPEN_BITN 30 4524 #define CPU_SCS_FPCCR_LSPEN_M 0x40000000 4525 #define CPU_SCS_FPCCR_LSPEN_S 30 4535 #define CPU_SCS_FPCCR_MONRDY 0x00000100 4536 #define CPU_SCS_FPCCR_MONRDY_BITN 8 4537 #define CPU_SCS_FPCCR_MONRDY_M 0x00000100 4538 #define CPU_SCS_FPCCR_MONRDY_S 8 4549 #define CPU_SCS_FPCCR_BFRDY 0x00000040 4550 #define CPU_SCS_FPCCR_BFRDY_BITN 6 4551 #define CPU_SCS_FPCCR_BFRDY_M 0x00000040 4552 #define CPU_SCS_FPCCR_BFRDY_S 6 4563 #define CPU_SCS_FPCCR_MMRDY 0x00000020 4564 #define CPU_SCS_FPCCR_MMRDY_BITN 5 4565 #define CPU_SCS_FPCCR_MMRDY_M 0x00000020 4566 #define CPU_SCS_FPCCR_MMRDY_S 5 4576 #define CPU_SCS_FPCCR_HFRDY 0x00000010 4577 #define CPU_SCS_FPCCR_HFRDY_BITN 4 4578 #define CPU_SCS_FPCCR_HFRDY_M 0x00000010 4579 #define CPU_SCS_FPCCR_HFRDY_S 4 4588 #define CPU_SCS_FPCCR_THREAD 0x00000008 4589 #define CPU_SCS_FPCCR_THREAD_BITN 3 4590 #define CPU_SCS_FPCCR_THREAD_M 0x00000008 4591 #define CPU_SCS_FPCCR_THREAD_S 3 4601 #define CPU_SCS_FPCCR_USER 0x00000002 4602 #define CPU_SCS_FPCCR_USER_BITN 1 4603 #define CPU_SCS_FPCCR_USER_M 0x00000002 4604 #define CPU_SCS_FPCCR_USER_S 1 4612 #define CPU_SCS_FPCCR_LSPACT 0x00000001 4613 #define CPU_SCS_FPCCR_LSPACT_BITN 0 4614 #define CPU_SCS_FPCCR_LSPACT_M 0x00000001 4615 #define CPU_SCS_FPCCR_LSPACT_S 0 4626 #define CPU_SCS_FPCAR_ADDRESS_W 30 4627 #define CPU_SCS_FPCAR_ADDRESS_M 0xFFFFFFFC 4628 #define CPU_SCS_FPCAR_ADDRESS_S 2 4639 #define CPU_SCS_FPDSCR_AHP 0x04000000 4640 #define CPU_SCS_FPDSCR_AHP_BITN 26 4641 #define CPU_SCS_FPDSCR_AHP_M 0x04000000 4642 #define CPU_SCS_FPDSCR_AHP_S 26 4648 #define CPU_SCS_FPDSCR_DN 0x02000000 4649 #define CPU_SCS_FPDSCR_DN_BITN 25 4650 #define CPU_SCS_FPDSCR_DN_M 0x02000000 4651 #define CPU_SCS_FPDSCR_DN_S 25 4657 #define CPU_SCS_FPDSCR_FZ 0x01000000 4658 #define CPU_SCS_FPDSCR_FZ_BITN 24 4659 #define CPU_SCS_FPDSCR_FZ_M 0x01000000 4660 #define CPU_SCS_FPDSCR_FZ_S 24 4672 #define CPU_SCS_FPDSCR_RMODE_W 2 4673 #define CPU_SCS_FPDSCR_RMODE_M 0x00C00000 4674 #define CPU_SCS_FPDSCR_RMODE_S 22 4685 #define CPU_SCS_MVFR0_FP_ROUNDING_MODES_W 4 4686 #define CPU_SCS_MVFR0_FP_ROUNDING_MODES_M 0xF0000000 4687 #define CPU_SCS_MVFR0_FP_ROUNDING_MODES_S 28 4693 #define CPU_SCS_MVFR0_SHORT_VECTORS_W 4 4694 #define CPU_SCS_MVFR0_SHORT_VECTORS_M 0x0F000000 4695 #define CPU_SCS_MVFR0_SHORT_VECTORS_S 24 4701 #define CPU_SCS_MVFR0_SQUARE_ROOT_W 4 4702 #define CPU_SCS_MVFR0_SQUARE_ROOT_M 0x00F00000 4703 #define CPU_SCS_MVFR0_SQUARE_ROOT_S 20 4709 #define CPU_SCS_MVFR0_DIVIDE_W 4 4710 #define CPU_SCS_MVFR0_DIVIDE_M 0x000F0000 4711 #define CPU_SCS_MVFR0_DIVIDE_S 16 4717 #define CPU_SCS_MVFR0_FP_EXCEPTION_TRAPPING_W 4 4718 #define CPU_SCS_MVFR0_FP_EXCEPTION_TRAPPING_M 0x0000F000 4719 #define CPU_SCS_MVFR0_FP_EXCEPTION_TRAPPING_S 12 4725 #define CPU_SCS_MVFR0_DOUBLE_PRECISION_W 4 4726 #define CPU_SCS_MVFR0_DOUBLE_PRECISION_M 0x00000F00 4727 #define CPU_SCS_MVFR0_DOUBLE_PRECISION_S 8 4733 #define CPU_SCS_MVFR0_SINGLE_PRECISION_W 4 4734 #define CPU_SCS_MVFR0_SINGLE_PRECISION_M 0x000000F0 4735 #define CPU_SCS_MVFR0_SINGLE_PRECISION_S 4 4741 #define CPU_SCS_MVFR0_A_SIMD_W 4 4742 #define CPU_SCS_MVFR0_A_SIMD_M 0x0000000F 4743 #define CPU_SCS_MVFR0_A_SIMD_S 0 4754 #define CPU_SCS_MVFR1_FP_FUSED_MAC_W 4 4755 #define CPU_SCS_MVFR1_FP_FUSED_MAC_M 0xF0000000 4756 #define CPU_SCS_MVFR1_FP_FUSED_MAC_S 28 4762 #define CPU_SCS_MVFR1_FP_HPFP_W 4 4763 #define CPU_SCS_MVFR1_FP_HPFP_M 0x0F000000 4764 #define CPU_SCS_MVFR1_FP_HPFP_S 24 4771 #define CPU_SCS_MVFR1_D_NAN_MODE_W 4 4772 #define CPU_SCS_MVFR1_D_NAN_MODE_M 0x000000F0 4773 #define CPU_SCS_MVFR1_D_NAN_MODE_S 4 4780 #define CPU_SCS_MVFR1_FTZ_MODE_W 4 4781 #define CPU_SCS_MVFR1_FTZ_MODE_M 0x0000000F 4782 #define CPU_SCS_MVFR1_FTZ_MODE_S 0 4784 #endif // __CPU_SCS__
© Copyright 1995-2022, Texas Instruments Incorporated. All rights reserved.
Trademarks | Privacy policy | Terms of use | Terms of sale