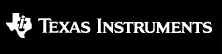 |
 |
Go to the documentation of this file. 34 #ifndef __HW_CPU_ITM_H__ 35 #define __HW_CPU_ITM_H__ 44 #define CPU_ITM_O_STIM0 0x00000000 47 #define CPU_ITM_O_STIM1 0x00000004 50 #define CPU_ITM_O_STIM2 0x00000008 53 #define CPU_ITM_O_STIM3 0x0000000C 56 #define CPU_ITM_O_STIM4 0x00000010 59 #define CPU_ITM_O_STIM5 0x00000014 62 #define CPU_ITM_O_STIM6 0x00000018 65 #define CPU_ITM_O_STIM7 0x0000001C 68 #define CPU_ITM_O_STIM8 0x00000020 71 #define CPU_ITM_O_STIM9 0x00000024 74 #define CPU_ITM_O_STIM10 0x00000028 77 #define CPU_ITM_O_STIM11 0x0000002C 80 #define CPU_ITM_O_STIM12 0x00000030 83 #define CPU_ITM_O_STIM13 0x00000034 86 #define CPU_ITM_O_STIM14 0x00000038 89 #define CPU_ITM_O_STIM15 0x0000003C 92 #define CPU_ITM_O_STIM16 0x00000040 95 #define CPU_ITM_O_STIM17 0x00000044 98 #define CPU_ITM_O_STIM18 0x00000048 101 #define CPU_ITM_O_STIM19 0x0000004C 104 #define CPU_ITM_O_STIM20 0x00000050 107 #define CPU_ITM_O_STIM21 0x00000054 110 #define CPU_ITM_O_STIM22 0x00000058 113 #define CPU_ITM_O_STIM23 0x0000005C 116 #define CPU_ITM_O_STIM24 0x00000060 119 #define CPU_ITM_O_STIM25 0x00000064 122 #define CPU_ITM_O_STIM26 0x00000068 125 #define CPU_ITM_O_STIM27 0x0000006C 128 #define CPU_ITM_O_STIM28 0x00000070 131 #define CPU_ITM_O_STIM29 0x00000074 134 #define CPU_ITM_O_STIM30 0x00000078 137 #define CPU_ITM_O_STIM31 0x0000007C 140 #define CPU_ITM_O_TER 0x00000E00 143 #define CPU_ITM_O_TPR 0x00000E40 146 #define CPU_ITM_O_TCR 0x00000E80 149 #define CPU_ITM_O_LAR 0x00000FB0 152 #define CPU_ITM_O_LSR 0x00000FB4 167 #define CPU_ITM_STIM0_STIM0_W 32 168 #define CPU_ITM_STIM0_STIM0_M 0xFFFFFFFF 169 #define CPU_ITM_STIM0_STIM0_S 0 184 #define CPU_ITM_STIM1_STIM1_W 32 185 #define CPU_ITM_STIM1_STIM1_M 0xFFFFFFFF 186 #define CPU_ITM_STIM1_STIM1_S 0 201 #define CPU_ITM_STIM2_STIM2_W 32 202 #define CPU_ITM_STIM2_STIM2_M 0xFFFFFFFF 203 #define CPU_ITM_STIM2_STIM2_S 0 218 #define CPU_ITM_STIM3_STIM3_W 32 219 #define CPU_ITM_STIM3_STIM3_M 0xFFFFFFFF 220 #define CPU_ITM_STIM3_STIM3_S 0 235 #define CPU_ITM_STIM4_STIM4_W 32 236 #define CPU_ITM_STIM4_STIM4_M 0xFFFFFFFF 237 #define CPU_ITM_STIM4_STIM4_S 0 252 #define CPU_ITM_STIM5_STIM5_W 32 253 #define CPU_ITM_STIM5_STIM5_M 0xFFFFFFFF 254 #define CPU_ITM_STIM5_STIM5_S 0 269 #define CPU_ITM_STIM6_STIM6_W 32 270 #define CPU_ITM_STIM6_STIM6_M 0xFFFFFFFF 271 #define CPU_ITM_STIM6_STIM6_S 0 286 #define CPU_ITM_STIM7_STIM7_W 32 287 #define CPU_ITM_STIM7_STIM7_M 0xFFFFFFFF 288 #define CPU_ITM_STIM7_STIM7_S 0 303 #define CPU_ITM_STIM8_STIM8_W 32 304 #define CPU_ITM_STIM8_STIM8_M 0xFFFFFFFF 305 #define CPU_ITM_STIM8_STIM8_S 0 320 #define CPU_ITM_STIM9_STIM9_W 32 321 #define CPU_ITM_STIM9_STIM9_M 0xFFFFFFFF 322 #define CPU_ITM_STIM9_STIM9_S 0 337 #define CPU_ITM_STIM10_STIM10_W 32 338 #define CPU_ITM_STIM10_STIM10_M 0xFFFFFFFF 339 #define CPU_ITM_STIM10_STIM10_S 0 354 #define CPU_ITM_STIM11_STIM11_W 32 355 #define CPU_ITM_STIM11_STIM11_M 0xFFFFFFFF 356 #define CPU_ITM_STIM11_STIM11_S 0 371 #define CPU_ITM_STIM12_STIM12_W 32 372 #define CPU_ITM_STIM12_STIM12_M 0xFFFFFFFF 373 #define CPU_ITM_STIM12_STIM12_S 0 388 #define CPU_ITM_STIM13_STIM13_W 32 389 #define CPU_ITM_STIM13_STIM13_M 0xFFFFFFFF 390 #define CPU_ITM_STIM13_STIM13_S 0 405 #define CPU_ITM_STIM14_STIM14_W 32 406 #define CPU_ITM_STIM14_STIM14_M 0xFFFFFFFF 407 #define CPU_ITM_STIM14_STIM14_S 0 422 #define CPU_ITM_STIM15_STIM15_W 32 423 #define CPU_ITM_STIM15_STIM15_M 0xFFFFFFFF 424 #define CPU_ITM_STIM15_STIM15_S 0 439 #define CPU_ITM_STIM16_STIM16_W 32 440 #define CPU_ITM_STIM16_STIM16_M 0xFFFFFFFF 441 #define CPU_ITM_STIM16_STIM16_S 0 456 #define CPU_ITM_STIM17_STIM17_W 32 457 #define CPU_ITM_STIM17_STIM17_M 0xFFFFFFFF 458 #define CPU_ITM_STIM17_STIM17_S 0 473 #define CPU_ITM_STIM18_STIM18_W 32 474 #define CPU_ITM_STIM18_STIM18_M 0xFFFFFFFF 475 #define CPU_ITM_STIM18_STIM18_S 0 490 #define CPU_ITM_STIM19_STIM19_W 32 491 #define CPU_ITM_STIM19_STIM19_M 0xFFFFFFFF 492 #define CPU_ITM_STIM19_STIM19_S 0 507 #define CPU_ITM_STIM20_STIM20_W 32 508 #define CPU_ITM_STIM20_STIM20_M 0xFFFFFFFF 509 #define CPU_ITM_STIM20_STIM20_S 0 524 #define CPU_ITM_STIM21_STIM21_W 32 525 #define CPU_ITM_STIM21_STIM21_M 0xFFFFFFFF 526 #define CPU_ITM_STIM21_STIM21_S 0 541 #define CPU_ITM_STIM22_STIM22_W 32 542 #define CPU_ITM_STIM22_STIM22_M 0xFFFFFFFF 543 #define CPU_ITM_STIM22_STIM22_S 0 558 #define CPU_ITM_STIM23_STIM23_W 32 559 #define CPU_ITM_STIM23_STIM23_M 0xFFFFFFFF 560 #define CPU_ITM_STIM23_STIM23_S 0 575 #define CPU_ITM_STIM24_STIM24_W 32 576 #define CPU_ITM_STIM24_STIM24_M 0xFFFFFFFF 577 #define CPU_ITM_STIM24_STIM24_S 0 592 #define CPU_ITM_STIM25_STIM25_W 32 593 #define CPU_ITM_STIM25_STIM25_M 0xFFFFFFFF 594 #define CPU_ITM_STIM25_STIM25_S 0 609 #define CPU_ITM_STIM26_STIM26_W 32 610 #define CPU_ITM_STIM26_STIM26_M 0xFFFFFFFF 611 #define CPU_ITM_STIM26_STIM26_S 0 626 #define CPU_ITM_STIM27_STIM27_W 32 627 #define CPU_ITM_STIM27_STIM27_M 0xFFFFFFFF 628 #define CPU_ITM_STIM27_STIM27_S 0 643 #define CPU_ITM_STIM28_STIM28_W 32 644 #define CPU_ITM_STIM28_STIM28_M 0xFFFFFFFF 645 #define CPU_ITM_STIM28_STIM28_S 0 660 #define CPU_ITM_STIM29_STIM29_W 32 661 #define CPU_ITM_STIM29_STIM29_M 0xFFFFFFFF 662 #define CPU_ITM_STIM29_STIM29_S 0 677 #define CPU_ITM_STIM30_STIM30_W 32 678 #define CPU_ITM_STIM30_STIM30_M 0xFFFFFFFF 679 #define CPU_ITM_STIM30_STIM30_S 0 694 #define CPU_ITM_STIM31_STIM31_W 32 695 #define CPU_ITM_STIM31_STIM31_M 0xFFFFFFFF 696 #define CPU_ITM_STIM31_STIM31_S 0 706 #define CPU_ITM_TER_STIMENA31 0x80000000 707 #define CPU_ITM_TER_STIMENA31_BITN 31 708 #define CPU_ITM_TER_STIMENA31_M 0x80000000 709 #define CPU_ITM_TER_STIMENA31_S 31 714 #define CPU_ITM_TER_STIMENA30 0x40000000 715 #define CPU_ITM_TER_STIMENA30_BITN 30 716 #define CPU_ITM_TER_STIMENA30_M 0x40000000 717 #define CPU_ITM_TER_STIMENA30_S 30 722 #define CPU_ITM_TER_STIMENA29 0x20000000 723 #define CPU_ITM_TER_STIMENA29_BITN 29 724 #define CPU_ITM_TER_STIMENA29_M 0x20000000 725 #define CPU_ITM_TER_STIMENA29_S 29 730 #define CPU_ITM_TER_STIMENA28 0x10000000 731 #define CPU_ITM_TER_STIMENA28_BITN 28 732 #define CPU_ITM_TER_STIMENA28_M 0x10000000 733 #define CPU_ITM_TER_STIMENA28_S 28 738 #define CPU_ITM_TER_STIMENA27 0x08000000 739 #define CPU_ITM_TER_STIMENA27_BITN 27 740 #define CPU_ITM_TER_STIMENA27_M 0x08000000 741 #define CPU_ITM_TER_STIMENA27_S 27 746 #define CPU_ITM_TER_STIMENA26 0x04000000 747 #define CPU_ITM_TER_STIMENA26_BITN 26 748 #define CPU_ITM_TER_STIMENA26_M 0x04000000 749 #define CPU_ITM_TER_STIMENA26_S 26 754 #define CPU_ITM_TER_STIMENA25 0x02000000 755 #define CPU_ITM_TER_STIMENA25_BITN 25 756 #define CPU_ITM_TER_STIMENA25_M 0x02000000 757 #define CPU_ITM_TER_STIMENA25_S 25 762 #define CPU_ITM_TER_STIMENA24 0x01000000 763 #define CPU_ITM_TER_STIMENA24_BITN 24 764 #define CPU_ITM_TER_STIMENA24_M 0x01000000 765 #define CPU_ITM_TER_STIMENA24_S 24 770 #define CPU_ITM_TER_STIMENA23 0x00800000 771 #define CPU_ITM_TER_STIMENA23_BITN 23 772 #define CPU_ITM_TER_STIMENA23_M 0x00800000 773 #define CPU_ITM_TER_STIMENA23_S 23 778 #define CPU_ITM_TER_STIMENA22 0x00400000 779 #define CPU_ITM_TER_STIMENA22_BITN 22 780 #define CPU_ITM_TER_STIMENA22_M 0x00400000 781 #define CPU_ITM_TER_STIMENA22_S 22 786 #define CPU_ITM_TER_STIMENA21 0x00200000 787 #define CPU_ITM_TER_STIMENA21_BITN 21 788 #define CPU_ITM_TER_STIMENA21_M 0x00200000 789 #define CPU_ITM_TER_STIMENA21_S 21 794 #define CPU_ITM_TER_STIMENA20 0x00100000 795 #define CPU_ITM_TER_STIMENA20_BITN 20 796 #define CPU_ITM_TER_STIMENA20_M 0x00100000 797 #define CPU_ITM_TER_STIMENA20_S 20 802 #define CPU_ITM_TER_STIMENA19 0x00080000 803 #define CPU_ITM_TER_STIMENA19_BITN 19 804 #define CPU_ITM_TER_STIMENA19_M 0x00080000 805 #define CPU_ITM_TER_STIMENA19_S 19 810 #define CPU_ITM_TER_STIMENA18 0x00040000 811 #define CPU_ITM_TER_STIMENA18_BITN 18 812 #define CPU_ITM_TER_STIMENA18_M 0x00040000 813 #define CPU_ITM_TER_STIMENA18_S 18 818 #define CPU_ITM_TER_STIMENA17 0x00020000 819 #define CPU_ITM_TER_STIMENA17_BITN 17 820 #define CPU_ITM_TER_STIMENA17_M 0x00020000 821 #define CPU_ITM_TER_STIMENA17_S 17 826 #define CPU_ITM_TER_STIMENA16 0x00010000 827 #define CPU_ITM_TER_STIMENA16_BITN 16 828 #define CPU_ITM_TER_STIMENA16_M 0x00010000 829 #define CPU_ITM_TER_STIMENA16_S 16 834 #define CPU_ITM_TER_STIMENA15 0x00008000 835 #define CPU_ITM_TER_STIMENA15_BITN 15 836 #define CPU_ITM_TER_STIMENA15_M 0x00008000 837 #define CPU_ITM_TER_STIMENA15_S 15 842 #define CPU_ITM_TER_STIMENA14 0x00004000 843 #define CPU_ITM_TER_STIMENA14_BITN 14 844 #define CPU_ITM_TER_STIMENA14_M 0x00004000 845 #define CPU_ITM_TER_STIMENA14_S 14 850 #define CPU_ITM_TER_STIMENA13 0x00002000 851 #define CPU_ITM_TER_STIMENA13_BITN 13 852 #define CPU_ITM_TER_STIMENA13_M 0x00002000 853 #define CPU_ITM_TER_STIMENA13_S 13 858 #define CPU_ITM_TER_STIMENA12 0x00001000 859 #define CPU_ITM_TER_STIMENA12_BITN 12 860 #define CPU_ITM_TER_STIMENA12_M 0x00001000 861 #define CPU_ITM_TER_STIMENA12_S 12 866 #define CPU_ITM_TER_STIMENA11 0x00000800 867 #define CPU_ITM_TER_STIMENA11_BITN 11 868 #define CPU_ITM_TER_STIMENA11_M 0x00000800 869 #define CPU_ITM_TER_STIMENA11_S 11 874 #define CPU_ITM_TER_STIMENA10 0x00000400 875 #define CPU_ITM_TER_STIMENA10_BITN 10 876 #define CPU_ITM_TER_STIMENA10_M 0x00000400 877 #define CPU_ITM_TER_STIMENA10_S 10 882 #define CPU_ITM_TER_STIMENA9 0x00000200 883 #define CPU_ITM_TER_STIMENA9_BITN 9 884 #define CPU_ITM_TER_STIMENA9_M 0x00000200 885 #define CPU_ITM_TER_STIMENA9_S 9 890 #define CPU_ITM_TER_STIMENA8 0x00000100 891 #define CPU_ITM_TER_STIMENA8_BITN 8 892 #define CPU_ITM_TER_STIMENA8_M 0x00000100 893 #define CPU_ITM_TER_STIMENA8_S 8 898 #define CPU_ITM_TER_STIMENA7 0x00000080 899 #define CPU_ITM_TER_STIMENA7_BITN 7 900 #define CPU_ITM_TER_STIMENA7_M 0x00000080 901 #define CPU_ITM_TER_STIMENA7_S 7 906 #define CPU_ITM_TER_STIMENA6 0x00000040 907 #define CPU_ITM_TER_STIMENA6_BITN 6 908 #define CPU_ITM_TER_STIMENA6_M 0x00000040 909 #define CPU_ITM_TER_STIMENA6_S 6 914 #define CPU_ITM_TER_STIMENA5 0x00000020 915 #define CPU_ITM_TER_STIMENA5_BITN 5 916 #define CPU_ITM_TER_STIMENA5_M 0x00000020 917 #define CPU_ITM_TER_STIMENA5_S 5 922 #define CPU_ITM_TER_STIMENA4 0x00000010 923 #define CPU_ITM_TER_STIMENA4_BITN 4 924 #define CPU_ITM_TER_STIMENA4_M 0x00000010 925 #define CPU_ITM_TER_STIMENA4_S 4 930 #define CPU_ITM_TER_STIMENA3 0x00000008 931 #define CPU_ITM_TER_STIMENA3_BITN 3 932 #define CPU_ITM_TER_STIMENA3_M 0x00000008 933 #define CPU_ITM_TER_STIMENA3_S 3 938 #define CPU_ITM_TER_STIMENA2 0x00000004 939 #define CPU_ITM_TER_STIMENA2_BITN 2 940 #define CPU_ITM_TER_STIMENA2_M 0x00000004 941 #define CPU_ITM_TER_STIMENA2_S 2 946 #define CPU_ITM_TER_STIMENA1 0x00000002 947 #define CPU_ITM_TER_STIMENA1_BITN 1 948 #define CPU_ITM_TER_STIMENA1_M 0x00000002 949 #define CPU_ITM_TER_STIMENA1_S 1 954 #define CPU_ITM_TER_STIMENA0 0x00000001 955 #define CPU_ITM_TER_STIMENA0_BITN 0 956 #define CPU_ITM_TER_STIMENA0_M 0x00000001 957 #define CPU_ITM_TER_STIMENA0_S 0 975 #define CPU_ITM_TPR_PRIVMASK_W 4 976 #define CPU_ITM_TPR_PRIVMASK_M 0x0000000F 977 #define CPU_ITM_TPR_PRIVMASK_S 0 987 #define CPU_ITM_TCR_BUSY 0x00800000 988 #define CPU_ITM_TCR_BUSY_BITN 23 989 #define CPU_ITM_TCR_BUSY_M 0x00800000 990 #define CPU_ITM_TCR_BUSY_S 23 997 #define CPU_ITM_TCR_ATBID_W 7 998 #define CPU_ITM_TCR_ATBID_M 0x007F0000 999 #define CPU_ITM_TCR_ATBID_S 16 1009 #define CPU_ITM_TCR_TSPRESCALE_W 2 1010 #define CPU_ITM_TCR_TSPRESCALE_M 0x00000300 1011 #define CPU_ITM_TCR_TSPRESCALE_S 8 1012 #define CPU_ITM_TCR_TSPRESCALE_DIV64 0x00000300 1013 #define CPU_ITM_TCR_TSPRESCALE_DIV16 0x00000200 1014 #define CPU_ITM_TCR_TSPRESCALE_DIV4 0x00000100 1015 #define CPU_ITM_TCR_TSPRESCALE_NOPRESCALING 0x00000000 1028 #define CPU_ITM_TCR_SWOENA 0x00000010 1029 #define CPU_ITM_TCR_SWOENA_BITN 4 1030 #define CPU_ITM_TCR_SWOENA_M 0x00000010 1031 #define CPU_ITM_TCR_SWOENA_S 4 1037 #define CPU_ITM_TCR_DWTENA 0x00000008 1038 #define CPU_ITM_TCR_DWTENA_BITN 3 1039 #define CPU_ITM_TCR_DWTENA_M 0x00000008 1040 #define CPU_ITM_TCR_DWTENA_S 3 1047 #define CPU_ITM_TCR_SYNCENA 0x00000004 1048 #define CPU_ITM_TCR_SYNCENA_BITN 2 1049 #define CPU_ITM_TCR_SYNCENA_M 0x00000004 1050 #define CPU_ITM_TCR_SYNCENA_S 2 1061 #define CPU_ITM_TCR_TSENA 0x00000002 1062 #define CPU_ITM_TCR_TSENA_BITN 1 1063 #define CPU_ITM_TCR_TSENA_M 0x00000002 1064 #define CPU_ITM_TCR_TSENA_S 1 1070 #define CPU_ITM_TCR_ITMENA 0x00000001 1071 #define CPU_ITM_TCR_ITMENA_BITN 0 1072 #define CPU_ITM_TCR_ITMENA_M 0x00000001 1073 #define CPU_ITM_TCR_ITMENA_S 0 1084 #define CPU_ITM_LAR_LOCK_ACCESS_W 32 1085 #define CPU_ITM_LAR_LOCK_ACCESS_M 0xFFFFFFFF 1086 #define CPU_ITM_LAR_LOCK_ACCESS_S 0 1096 #define CPU_ITM_LSR_BYTEACC 0x00000004 1097 #define CPU_ITM_LSR_BYTEACC_BITN 2 1098 #define CPU_ITM_LSR_BYTEACC_M 0x00000004 1099 #define CPU_ITM_LSR_BYTEACC_S 2 1105 #define CPU_ITM_LSR_ACCESS 0x00000002 1106 #define CPU_ITM_LSR_ACCESS_BITN 1 1107 #define CPU_ITM_LSR_ACCESS_M 0x00000002 1108 #define CPU_ITM_LSR_ACCESS_S 1 1113 #define CPU_ITM_LSR_PRESENT 0x00000001 1114 #define CPU_ITM_LSR_PRESENT_BITN 0 1115 #define CPU_ITM_LSR_PRESENT_M 0x00000001 1116 #define CPU_ITM_LSR_PRESENT_S 0 1119 #endif // __CPU_ITM__
© Copyright 1995-2021, Texas Instruments Incorporated. All rights reserved.
Trademarks | Privacy policy | Terms of use | Terms of sale