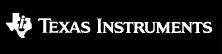 |
 |
Go to the documentation of this file. 33 #ifndef __HW_CPU_TPIU_H__ 34 #define __HW_CPU_TPIU_H__ 43 #define CPU_TPIU_O_SSPSR 0x00000000 46 #define CPU_TPIU_O_CSPSR 0x00000004 49 #define CPU_TPIU_O_ACPR 0x00000010 52 #define CPU_TPIU_O_SPPR 0x000000F0 55 #define CPU_TPIU_O_FFSR 0x00000300 58 #define CPU_TPIU_O_FFCR 0x00000304 61 #define CPU_TPIU_O_FSCR 0x00000308 64 #define CPU_TPIU_O_CLAIMMASK 0x00000FA0 67 #define CPU_TPIU_O_CLAIMSET 0x00000FA0 70 #define CPU_TPIU_O_CLAIMTAG 0x00000FA4 73 #define CPU_TPIU_O_CLAIMCLR 0x00000FA4 76 #define CPU_TPIU_O_LAR 0x00000FB0 79 #define CPU_TPIU_O_DEVID 0x00000FC8 92 #define CPU_TPIU_SSPSR_FOUR 0x00000008 93 #define CPU_TPIU_SSPSR_FOUR_BITN 3 94 #define CPU_TPIU_SSPSR_FOUR_M 0x00000008 95 #define CPU_TPIU_SSPSR_FOUR_S 3 103 #define CPU_TPIU_SSPSR_THREE 0x00000004 104 #define CPU_TPIU_SSPSR_THREE_BITN 2 105 #define CPU_TPIU_SSPSR_THREE_M 0x00000004 106 #define CPU_TPIU_SSPSR_THREE_S 2 114 #define CPU_TPIU_SSPSR_TWO 0x00000002 115 #define CPU_TPIU_SSPSR_TWO_BITN 1 116 #define CPU_TPIU_SSPSR_TWO_M 0x00000002 117 #define CPU_TPIU_SSPSR_TWO_S 1 125 #define CPU_TPIU_SSPSR_ONE 0x00000001 126 #define CPU_TPIU_SSPSR_ONE_BITN 0 127 #define CPU_TPIU_SSPSR_ONE_M 0x00000001 128 #define CPU_TPIU_SSPSR_ONE_S 0 140 #define CPU_TPIU_CSPSR_FOUR 0x00000008 141 #define CPU_TPIU_CSPSR_FOUR_BITN 3 142 #define CPU_TPIU_CSPSR_FOUR_M 0x00000008 143 #define CPU_TPIU_CSPSR_FOUR_S 3 150 #define CPU_TPIU_CSPSR_THREE 0x00000004 151 #define CPU_TPIU_CSPSR_THREE_BITN 2 152 #define CPU_TPIU_CSPSR_THREE_M 0x00000004 153 #define CPU_TPIU_CSPSR_THREE_S 2 160 #define CPU_TPIU_CSPSR_TWO 0x00000002 161 #define CPU_TPIU_CSPSR_TWO_BITN 1 162 #define CPU_TPIU_CSPSR_TWO_M 0x00000002 163 #define CPU_TPIU_CSPSR_TWO_S 1 170 #define CPU_TPIU_CSPSR_ONE 0x00000001 171 #define CPU_TPIU_CSPSR_ONE_BITN 0 172 #define CPU_TPIU_CSPSR_ONE_M 0x00000001 173 #define CPU_TPIU_CSPSR_ONE_S 0 183 #define CPU_TPIU_ACPR_PRESCALER_W 13 184 #define CPU_TPIU_ACPR_PRESCALER_M 0x00001FFF 185 #define CPU_TPIU_ACPR_PRESCALER_S 0 200 #define CPU_TPIU_SPPR_PROTOCOL_W 2 201 #define CPU_TPIU_SPPR_PROTOCOL_M 0x00000003 202 #define CPU_TPIU_SPPR_PROTOCOL_S 0 203 #define CPU_TPIU_SPPR_PROTOCOL_SWO_NRZ 0x00000002 204 #define CPU_TPIU_SPPR_PROTOCOL_SWO_MANCHESTER 0x00000001 205 #define CPU_TPIU_SPPR_PROTOCOL_TRACEPORT 0x00000000 216 #define CPU_TPIU_FFSR_FTNONSTOP 0x00000008 217 #define CPU_TPIU_FFSR_FTNONSTOP_BITN 3 218 #define CPU_TPIU_FFSR_FTNONSTOP_M 0x00000008 219 #define CPU_TPIU_FFSR_FTNONSTOP_S 3 229 #define CPU_TPIU_FFCR_TRIGIN 0x00000100 230 #define CPU_TPIU_FFCR_TRIGIN_BITN 8 231 #define CPU_TPIU_FFCR_TRIGIN_M 0x00000100 232 #define CPU_TPIU_FFCR_TRIGIN_S 8 240 #define CPU_TPIU_FFCR_ENFCONT 0x00000002 241 #define CPU_TPIU_FFCR_ENFCONT_BITN 1 242 #define CPU_TPIU_FFCR_ENFCONT_M 0x00000002 243 #define CPU_TPIU_FFCR_ENFCONT_S 1 255 #define CPU_TPIU_FSCR_FSCR_W 32 256 #define CPU_TPIU_FSCR_FSCR_M 0xFFFFFFFF 257 #define CPU_TPIU_FSCR_FSCR_S 0 274 #define CPU_TPIU_CLAIMMASK_CLAIMMASK_W 32 275 #define CPU_TPIU_CLAIMMASK_CLAIMMASK_M 0xFFFFFFFF 276 #define CPU_TPIU_CLAIMMASK_CLAIMMASK_S 0 293 #define CPU_TPIU_CLAIMSET_CLAIMSET_W 32 294 #define CPU_TPIU_CLAIMSET_CLAIMSET_M 0xFFFFFFFF 295 #define CPU_TPIU_CLAIMSET_CLAIMSET_S 0 309 #define CPU_TPIU_CLAIMTAG_CLAIMTAG_W 32 310 #define CPU_TPIU_CLAIMTAG_CLAIMTAG_M 0xFFFFFFFF 311 #define CPU_TPIU_CLAIMTAG_CLAIMTAG_S 0 328 #define CPU_TPIU_CLAIMCLR_CLAIMCLR_W 32 329 #define CPU_TPIU_CLAIMCLR_CLAIMCLR_M 0xFFFFFFFF 330 #define CPU_TPIU_CLAIMCLR_CLAIMCLR_S 0 342 #define CPU_TPIU_DEVID_NRZ_SWO 0x00000400 343 #define CPU_TPIU_DEVID_NRZ_SWO_BITN 11 344 #define CPU_TPIU_DEVID_NRZ_SWO_M 0x00000400 345 #define CPU_TPIU_DEVID_NRZ_SWO_S 11 350 #define CPU_TPIU_DEVID_MANCHESTER_SWO 0x00000200 351 #define CPU_TPIU_DEVID_MANCHESTER_SWO_BITN 10 352 #define CPU_TPIU_DEVID_MANCHESTER_SWO_M 0x00000200 353 #define CPU_TPIU_DEVID_MANCHESTER_SWO_S 10 359 #define CPU_TPIU_DEVID_PARALLEL_TRACE 0x00000100 360 #define CPU_TPIU_DEVID_PARALLEL_TRACE_BITN 9 361 #define CPU_TPIU_DEVID_PARALLEL_TRACE_M 0x00000100 362 #define CPU_TPIU_DEVID_PARALLEL_TRACE_S 9 367 #define CPU_TPIU_DEVID_FIFO_SIZE_W 2 368 #define CPU_TPIU_DEVID_FIFO_SIZE_M 0x000001C0 369 #define CPU_TPIU_DEVID_FIFO_SIZE_S 6 375 #define CPU_TPIU_DEVID_ASYNC_TRACECLKIN 0x00000020 376 #define CPU_TPIU_DEVID_ASYNC_TRACECLKIN_BITN 5 377 #define CPU_TPIU_DEVID_ASYNC_TRACECLKIN_M 0x00000020 378 #define CPU_TPIU_DEVID_ASYNC_TRACECLKIN_S 5 387 #define CPU_TPIU_DEVID_NUM_INPUTS_W 5 388 #define CPU_TPIU_DEVID_NUM_INPUTS_M 0x0000001F 389 #define CPU_TPIU_DEVID_NUM_INPUTS_S 0 390 #define CPU_TPIU_DEVID_NUM_INPUTS_ONE 0x00000000 391 #define CPU_TPIU_DEVID_NUM_INPUTS_TWO 0x00000001 393 #endif // __CPU_TPIU__
© Copyright 1995-2022, Texas Instruments Incorporated. All rights reserved.
Trademarks | Privacy policy | Terms of use | Terms of sale