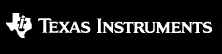 |
 |
Go to the documentation of this file.
23 #define TIMER_CNT_UP 0x0000
24 #define TIMER_CNT_DN 0x0001
25 #define TIMER_CNT_UPDN 0x0002
26 #define TIMER_STOP 0x0003
28 #define CNTLD_ENABLE 0x0004
29 #define CNTLD_DISABLE 0x0000
31 #define PRDLD_IMMEDIATE 0x0008
32 #define PRDLD_SHADOW 0x0000
34 #define SYNCOSEL_EPWMSYNCI 0x0000
35 #define SYNCOSEL_CNT_EQ 0x0010
36 #define SYNCOSEL_CMPB_EQ 0x0020
37 #define SYNCOSEL_DISABLE 0x0030
39 #define HSPCLKDIV_PRESCALE_X_1 0x0000
40 #define HSPCLKDIV_PRESCALE_X_2 0x0080
41 #define HSPCLKDIV_PRESCALE_X_4 0x0100
42 #define HSPCLKDIV_PRESCALE_X_6 0x0180
43 #define HSPCLKDIV_PRESCALE_X_8 0x0200
44 #define HSPCLKDIV_PRESCALE_X_10 0x0280
45 #define HSPCLKDIV_PRESCALE_X_12 0x0300
46 #define HSPCLKDIV_PRESCALE_X_14 0x0380
48 #define CLKDIV_PRESCALE_X_1 0x0000
49 #define CLKDIV_PRESCALE_X_2 0x0400
50 #define CLKDIV_PRESCALE_X_4 0x0800
51 #define CLKDIV_PRESCALE_X_8 0x0C00
52 #define CLKDIV_PRESCALE_X_16 0x1000
53 #define CLKDIV_PRESCALE_X_32 0x1400
54 #define CLKDIV_PRESCALE_X_64 0x1800
55 #define CLKDIV_PRESCALE_X_128 0x1C00
57 #define PHSDIR_CNT_UP 0x2000
58 #define PHSDIR_CNT_DN 0x0000
60 #define FREE_RUN_FLAG 0x8000
61 #define SOFT_STOP_FLAG 0x4000
66 #define LOADAMODE_ZRO 0x0000
67 #define LOADAMODE_PRD 0x0001
68 #define LOADAMODE_ZRO_PRD 0x0002
69 #define LOADAMODE_FRZ 0x0003
71 #define LOADBMODE_ZRO 0x0000
72 #define LOADBMODE_PRD 0x0004
73 #define LOADBMODE_ZRO_PRD 0x0008
74 #define LOADBMODE_FRZ 0x000C
76 #define SHDWAMODE_IMMEDIATE 0x0010
77 #define SHDWAMODE_SHADOW 0x0000
79 #define SHDWBMODE_IMMEDIATE 0x0040
80 #define SHDWBMODE_SHADOW 0x0000
85 #define ZRO_NOTHING 0x0000
86 #define ZRO_CLEAR 0x0001
87 #define ZRO_SET 0x0002
88 #define ZRO_TOGGLE 0x0003
90 #define PRD_NOTHING 0x0000
91 #define PRD_CLEAR 0x0004
92 #define PRD_SET 0x0008
93 #define PRD_TOGGLE 0x000C
95 #define CAU_NOTHING 0x0000
96 #define CAU_CLEAR 0x0010
97 #define CAU_SET 0x0020
98 #define CAU_TOGGLE 0x0030
100 #define CAD_NOTHING 0x0000
101 #define CAD_CLEAR 0x0040
102 #define CAD_SET 0x0080
103 #define CAD_TOGGLE 0x00C0
105 #define CBU_NOTHING 0x0000
106 #define CBU_CLEAR 0x0100
107 #define CBU_SET 0x0200
108 #define CBU_TOGGLE 0x0300
110 #define CBD_NOTHING 0x0000
111 #define CBD_CLEAR 0x0400
112 #define CBD_SET 0x0800
113 #define CBD_TOGGLE 0x0C00
118 #define ACTSFA_NOTHING 0x0000
119 #define ACTSFA_CLEAR 0x0001
120 #define ACTSFA_SET 0x0002
121 #define ACTSFA_TOGGLE 0x0003
123 #define ACTSFB_NOTHING 0x0000
124 #define ACTSFB_CLEAR 0x0008
125 #define ACTSFB_SET 0x0010
126 #define ACTSFB_TOGGLE 0x0018
128 #define RLDCSF_ZRO 0x0000
129 #define RLDCSF_PRD 0x0040
130 #define RLDCSF_ZRO_PRD 0x0080
131 #define RLDCSF_IMMEDIATE 0x00C0
136 #define CSFA_DISABLE 0x0000
137 #define CSFA_LOW 0x0001
138 #define CSFA_HIGH 0x0002
140 #define CSFB_DISABLE 0x0000
141 #define CSFB_LOW 0x0004
142 #define CSFB_HIGH 0x0008
147 #define BP_DISABLE 0x0000
148 #define BP_BYPASSA_FLA 0x0001
149 #define BP_BYPASSB_RSA 0x0002
150 #define BP_ENABLE 0x0003
152 #define POLSEL_ACTIVE_HI 0x0000
153 #define POLSEL_ACTIVE_LO_CMP 0x0004
154 #define POLSEL_ACTIVE_HI_CMP 0x0008
155 #define POLSEL_ACTIVE_LO 0x000C
160 #define CHPEN_ENABLE 0x0001
161 #define CHPEN_DISABLE 0x0000
163 #define OSHTWTH_X_1 0x0000
164 #define OSHTWTH_X_2 0x0002
165 #define OSHTWTH_X_3 0x0004
166 #define OSHTWTH_X_4 0x0006
167 #define OSHTWTH_X_5 0x0008
168 #define OSHTWTH_X_6 0x000A
169 #define OSHTWTH_X_7 0x000C
170 #define OSHTWTH_X_8 0x000E
171 #define OSHTWTH_X_9 0x0010
172 #define OSHTWTH_X_10 0x0012
173 #define OSHTWTH_X_11 0x0014
174 #define OSHTWTH_X_12 0x0016
175 #define OSHTWTH_X_13 0x0018
176 #define OSHTWTH_X_14 0x001A
177 #define OSHTWTH_X_15 0x001C
178 #define OSHTWTH_X_16 0x001E
180 #define CHPFREQ_X_1 0x0000
181 #define CHPFREQ_X_2 0x0020
182 #define CHPFREQ_X_3 0x0040
183 #define CHPFREQ_X_4 0x0060
184 #define CHPFREQ_X_5 0x0080
185 #define CHPFREQ_X_6 0x00A0
186 #define CHPFREQ_X_7 0x00C0
187 #define CHPFREQ_X_8 0x00E0
189 #define CHPDUTY_X_1 0x0000
190 #define CHPDUTY_X_2 0x0100
191 #define CHPDUTY_X_3 0x0200
192 #define CHPDUTY_X_4 0x0300
193 #define CHPDUTY_X_5 0x0400
194 #define CHPDUTY_X_6 0x0500
195 #define CHPDUTY_X_7 0x0600
200 #define DISABLE_TZSEL 0x0000
202 #define DISABLE_TZ1_CBC 0x0000
203 #define ENABLE_TZ1_CBC 0x0001
205 #define DISABLE_TZ2_CBC 0x0000
206 #define ENABLE_TZ2_CBC 0x0002
208 #define DISABLE_TZ3_CBC 0x0000
209 #define ENABLE_TZ3_CBC 0x0004
211 #define DISABLE_TZ4_CBC 0x0000
212 #define ENABLE_TZ4_CBC 0x0008
214 #define DISABLE_TZ5_CBC 0x0000
215 #define ENABLE_TZ5_CBC 0x0010
217 #define DISABLE_TZ6_CBC 0x0000
218 #define ENABLE_TZ6_CBC 0x0020
220 #define DISABLE_DCAEVT2_CBC 0x0000
221 #define ENABLE_DCAEVT2_CBC 0x0040
223 #define DISABLE_DCBEVT2_CBC 0x0000
224 #define ENABLE_DCBEVT2_CBC 0x0080
226 #define DISABLE_TZ1_OST 0x0000
227 #define ENABLE_TZ1_OST 0x0100
229 #define DISABLE_TZ2_OST 0x0000
230 #define ENABLE_TZ2_OST 0x0200
232 #define DISABLE_TZ3_OST 0x0000
233 #define ENABLE_TZ3_OST 0x0400
235 #define DISABLE_TZ4_OST 0x0000
236 #define ENABLE_TZ4_OST 0x0800
238 #define DISABLE_TZ5_OST 0x0000
239 #define ENABLE_TZ5_OST 0x1000
241 #define DISABLE_TZ6_OST 0x0000
242 #define ENABLE_TZ6_OST 0x2000
244 #define DISABLE_DCAEVT1_OST 0x0000
245 #define ENABLE_DCAEVT1_OST 0x4000
247 #define DISABLE_DCBEVT1_OST 0x0000
248 #define ENABLE_DCBEVT1_OST 0x8000
253 #define TZA_HI_Z 0x0000
254 #define TZA_FORCE_HI 0x0001
255 #define TZA_FORCE_LO 0x0002
256 #define TZA_NOTHING 0x0003
258 #define TZB_HI_Z 0x0000
259 #define TZB_FORCE_HI 0x0004
260 #define TZB_FORCE_LO 0x0008
261 #define TZB_NOTHING 0x000C
263 #define DCAEVT1_HI_Z 0x0000
264 #define DCAEVT1_FORCE_HI 0x0010
265 #define DCAEVT1_FORCE_LO 0x0020
266 #define DCAEVT1_NOTHING 0x0030
268 #define DCAEVT2_HI_Z 0x0000
269 #define DCAEVT2_FORCE_HI 0x0040
270 #define DCAEVT2_FORCE_LO 0x0080
271 #define DCAEVT2_NOTHING 0x00C0
273 #define DCBEVT1_HI_Z 0x0000
274 #define DCBEVT1_FORCE_HI 0x0100
275 #define DCBEVT1_FORCE_LO 0x0200
276 #define DCBEVT1_NOTHING 0x0300
278 #define DCBEVT2_HI_Z 0x0000
279 #define DCBEVT2_FORCE_HI 0x0400
280 #define DCBEVT2_FORCE_LO 0x0800
281 #define DCBEVT2_NOTHING 0x0C00
286 #define DISABLE_CBC_INT 0x0000
287 #define ENABLE_CBC_INT 0x0002
289 #define DISABLE_OST_INT 0x0000
290 #define ENABLE_OST_INT 0x0004
292 #define DISABLE_DCAEVT1_INT 0x0000
293 #define ENABLE_DCAEVT1_INT 0x0008
295 #define DISABLE_DCAEVT2_INT 0x0000
296 #define ENABLE_DCAEVT2_INT 0x0010
298 #define DISABLE_DCBEVT1_INT 0x0000
299 #define ENABLE_DCBEVT1_INT 0x0020
301 #define DISABLE_DCBEVT2_INT 0x0000
302 #define ENABLE_DCBEVT2_INT 0x0040
307 #define INTSEL_CNT_ZERO 0x0001
308 #define INTSEL_PRD_EQ 0x0002
309 #define INTSEL_CMPA_EQ_UC 0x0004
310 #define INTSEL_CMPA_EQ_DC 0x0005
311 #define INTSEL_CMPB_EQ_UC 0x0006
312 #define INTSEL_CMPB_EQ_DC 0x0007
314 #define INTSEL_DISABLE 0x0000
315 #define INTSEL_ENABLE 0x0008
317 #define SOCASEL_CNT_ZERO 0x0100
318 #define SOCASEL_PRD_EQ 0x0200
319 #define SOCASEL_CMPA_EQ_UC 0x0400
320 #define SOCASEL_CMPA_EQ_DC 0x0500
321 #define SOCASEL_CMPB_EQ_UC 0x0600
322 #define SOCASEL_CMPB_EQ_DC 0x0700
324 #define SOCASEL_DISABLE 0x0000
325 #define SOCASEL_ENABLE 0x0800
327 #define SOCBSEL_CNT_ZERO 0x1000
328 #define SOCBSEL_PRD_EQ 0x2000
329 #define SOCBSEL_CMPA_EQ_UC 0x4000
330 #define SOCBSEL_CMPA_EQ_DC 0x5000
331 #define SOCBSEL_CMPB_EQ_UC 0x6000
332 #define SOCBSEL_CMPB_EQ_DC 0x7000
334 #define SOCBSEL_DISABLE 0x0000
335 #define SOCBSEL_ENABLE 0x8000
340 #define INTPRD_DISABLE 0x0000
341 #define INTPRD_ONE_EVENT 0x0001
342 #define INTPRD_TWO_EVENT 0x0002
343 #define INTPRD_THREE_EVENT 0x0003
345 #define SOCAPRD_DISABLE 0x0000
346 #define SOCAPRD_ONE_EVENT 0x0100
347 #define SOCAPRD_TWO_EVENT 0x0200
348 #define SOCAPRD_THREE_EVENT 0x0300
350 #define SOCBPRD_DISABLE 0x0000
351 #define SOCBPRD_ONE_EVENT 0x1000
352 #define SOCBPRD_TWO_EVENT 0x2000
353 #define SOCBPRD_THREE_EVENT 0x3000
358 #define CAP1POL_RISING_EDGE 0x0000
359 #define CAP1POL_FALLING_EDGE 0x0001
361 #define CTRRST1_ABSOLUTE_TS 0x0000
362 #define CTRRST1_DIFFERENCE_TS 0x0002
364 #define CAP2POL_RISING_EDGE 0x0000
365 #define CAP2POL_FALLING_EDGE 0x0004
367 #define CTRRST2_ABSOLUTE_TS 0x0000
368 #define CTRRST2_DIFFERENCE_TS 0x0008
370 #define CAP3POL_RISING_EDGE 0x0000
371 #define CAP3POL_FALLING_EDGE 0x0010
373 #define CTRRST3_ABSOLUTE_TS 0x0000
374 #define CTRRST3_DIFFERENCE_TS 0x0020
376 #define CAP4POL_RISING_EDGE 0x0000
377 #define CAP4POL_FALLING_EDGE 0x0040
379 #define CTRRST4_ABSOLUTE_TS 0x0000
380 #define CTRRST4_DIFFERENCE_TS 0x0080
382 #define CAPLDEN_DISABLE 0x0000
383 #define CAPLDEN_ENABLE 0x0100
385 #define EVTFLTPS_X_1 0x0000
386 #define EVTFLTPS_X_2 0x0200
387 #define EVTFLTPS_X_4 0x0400
388 #define EVTFLTPS_X_6 0x0600
389 #define EVTFLTPS_X_8 0x0800
390 #define EVTFLTPS_X_10 0x0A00
391 #define EVTFLTPS_X_12 0x0C00
392 #define EVTFLTPS_X_14 0x0E00
393 #define EVTFLTPS_X_16 0x1000
394 #define EVTFLTPS_X_18 0x1200
395 #define EVTFLTPS_X_20 0x1400
396 #define EVTFLTPS_X_22 0x1600
397 #define EVTFLTPS_X_24 0x1800
398 #define EVTFLTPS_X_26 0x1A00
399 #define EVTFLTPS_X_28 0x1C00
400 #define EVTFLTPS_X_30 0x1E00
401 #define EVTFLTPS_X_32 0x2000
402 #define EVTFLTPS_X_34 0x2200
403 #define EVTFLTPS_X_36 0x2400
404 #define EVTFLTPS_X_38 0x2600
405 #define EVTFLTPS_X_40 0x2800
406 #define EVTFLTPS_X_42 0x2A00
407 #define EVTFLTPS_X_44 0x2C00
408 #define EVTFLTPS_X_46 0x2E00
409 #define EVTFLTPS_X_48 0x3000
410 #define EVTFLTPS_X_50 0x3200
411 #define EVTFLTPS_X_52 0x3400
412 #define EVTFLTPS_X_54 0x3600
413 #define EVTFLTPS_X_56 0x3800
414 #define EVTFLTPS_X_58 0x3A00
415 #define EVTFLTPS_X_60 0x3C00
416 #define EVTFLTPS_X_62 0x3E00
418 #define EMULATION_SOFT 0x0000
419 #define EMULATION_FREE 0x8000
424 #define CONTINUOUS_MODE 0x0000
425 #define ONE_SHOT_MODE 0x0001
427 #define ONESHT_CAP_EV1 0x0000
428 #define ONESHT_CAP_EV2 0x0002
429 #define ONESHT_CAP_EV3 0x0004
430 #define ONESHT_CAP_EV4 0x0006
432 #define TSCNTSTP_STOP 0x0000
433 #define TSCNTSTP_FREE 0x0010
435 #define SYNCI_DISABLE 0x0000
436 #define SYNCI_ENABLE 0x0020
438 #define SYNCO_SYNC_IN 0x0000
439 #define SYNCO_PRD_EQ 0x0040
440 #define SYNCO_DISABLE 0x0080
442 #define CAPTURE_MODE 0x0000
443 #define APWM_MODE 0x0200
445 #define APWMPOL_HIGH 0x0000
446 #define APWMPOL_LOW 0x0400
451 #define QSP_NO_EFFECT 0x0000
452 #define QSP_NEGATE 0x0020
454 #define QIP_NO_EFFECT 0x0000
455 #define QIP_NEGATE 0x0040
457 #define QBP_NO_EFFECT 0x0000
458 #define QBP_NEGATE 0x0080
460 #define QAP_NO_EFFECT 0x0000
461 #define QAP_NEGATE 0x0100
463 #define IGATE_DISABLE 0x0000
464 #define IGATE_WITH_STROBE 0x0200
466 #define SWAP_DISABLE 0x0000
467 #define SWAP_ENABLE 0x0400
469 #define XCR_X2 0x0000
470 #define XCR_X1 0x0800
472 #define SPSEL_INDEX 0x0000
473 #define SPSEL_STROBE 0x1000
475 #define SOEN_DISABLE 0x0000
476 #define SOEN_ENABLE 0x2000
478 #define QSRC_QUAD_MODE 0x0000
479 #define QSRC_DIR_MODE 0x4000
480 #define QSRC_UPCNT_MODE 0x8000
481 #define QSRC_DWNCNT_MODE 0xC000
486 #define WDE_DISABLE 0x0000
487 #define WDE_ENABLE 0x0001
489 #define UTE_DISABLE 0x0000
490 #define UTE_ENABLE 0x0002
492 #define QCLM_POSCNT 0x0000
493 #define QCLM_TIME_OUT 0x0004
495 #define QPEN_RESET 0x0000
496 #define QPEN_ENABLE 0x0008
498 #define IEL_RISING 0x0010
499 #define IEL_FALLING 0x0020
500 #define IEL_SOFTWARE 0x0030
502 #define SEL_RISING 0x0000
503 #define SEL_RISING_FALLING 0x0040
505 #define SWI_DISABLE 0x0000
506 #define SWI_POSCNT_INIT 0x0080
508 #define IEI_RISING 0x0200
509 #define IEI_FALLING 0x0300
511 #define SEI_RISING 0x0800
512 #define SEI_RISING_FALLING 0x0C00
514 #define PCRM_INDEX 0x0000
515 #define PCRM_POSMAX 0x1000
516 #define PCRM_FIRST_INDEX 0x2000
517 #define PCRM_TIME_EVENT 0x3000
519 #define QEP_EMULATION_SOFT 0x4000
520 #define QEP_EMULATION_FREE 0x8000
525 #define PCE_DISABLE 0x0000
526 #define PCE_ENABLE 0x1000
528 #define PCPOL_HIGH 0x0000
529 #define PCPOL_LOW 0x2000
531 #define PCLOAD_ZERO 0x0000
532 #define PCLOAD_QPOSCMP 0x4000
534 #define PCSHDW_DISABLE 0x0000
535 #define PCSHDW_ENABLE 0x8000
540 #define UPPS_X1 0x0000
541 #define UPPS_X2 0x0001
542 #define UPPS_X3 0x0002
543 #define UPPS_X8 0x0003
544 #define UPPS_X16 0x0004
545 #define UPPS_X32 0x0005
546 #define UPPS_X64 0x0006
547 #define UPPS_X128 0x0007
548 #define UPPS_X256 0x0008
549 #define UPPS_X512 0x0009
550 #define UPPS_X1024 0x000A
551 #define UPPS_X2048 0x000B
553 #define CCPS_X1 0x0000
554 #define CCPS_X2 0x0010
555 #define CCPS_X4 0x0020
556 #define CCPS_X8 0x0030
557 #define CCPS_X16 0x0040
558 #define CCPS_X32 0x0050
559 #define CCPS_X64 0x0060
560 #define CCPS_X128 0x0070
562 #define CEN_DISABLE 0x0000
563 #define CEN_ENABLE 0x8000
568 #define ADC_SUS_MODE0 0x0000
569 #define ADC_SUS_MODE1 0X1000
570 #define ADC_SUS_MODE2 0x2000
571 #define ADC_SUS_MODE3 0X3000
572 #define ADC_RESET_FLAG 0x4000
574 #define ADC_ACQ_PS_1 0x0000
575 #define ADC_ACQ_PS_2 0x0100
576 #define ADC_ACQ_PS_3 0x0200
577 #define ADC_ACQ_PS_4 0x0300
578 #define ADC_ACQ_PS_5 0x0400
579 #define ADC_ACQ_PS_6 0x0500
580 #define ADC_ACQ_PS_7 0x0600
581 #define ADC_ACQ_PS_8 0x0700
582 #define ADC_ACQ_PS_9 0x0800
583 #define ADC_ACQ_PS_10 0x0900
584 #define ADC_ACQ_PS_11 0x0A00
585 #define ADC_ACQ_PS_12 0x0B00
586 #define ADC_ACQ_PS_13 0x0C00
587 #define ADC_ACQ_PS_14 0x0D00
588 #define ADC_ACQ_PS_15 0x0E00
589 #define ADC_ACQ_PS_16 0x0F00
591 #define ADC_CPS_1 0x0000
592 #define ADC_CPS_2 0x0080
593 #define ADC_CONT_RUN 0x0040
594 #define ADC_SEQ_CASC 0x0010
595 #define ADC_SEQ_DUAL 0x0000
600 #define ADC_EPWM_SOCB 0x8000
601 #define ADC_RST_SEQ1 0x4000
602 #define ADC_SOC_SEQ1 0x2000
604 #define ADC_INT_ENA_SEQ1 0x0800
605 #define ADC_INT_MODE_SEQ1 0X0400
606 #define ADC_EPWM_SOCA_SEQ1 0x0100
608 #define ADC_EXT_SOC_SEQ1 0x0080
609 #define ADC_RST_SEQ2 0x0040
610 #define ADC_SOC_SEQ2 0x0020
612 #define ADC_INT_ENA_SEQ2 0x0008
613 #define ADC_INT_MODE_SEQ2 0x0004
614 #define ADC_EPWM_SOCB_SEQ2 0x0001
619 #define ADC_RFDN 0x0080
620 #define ADC_BGDN 0x0040
621 #define ADC_PWDN 0x0020
623 #define ADC_CLKPS_X_1 0x0000
624 #define ADC_CLKPS_X_2 0x0002
625 #define ADC_CLKPS_X_4 0x0004
626 #define ADC_CLKPS_X_6 0x0006
627 #define ADC_CLKPS_X_8 0x0008
628 #define ADC_CLKPS_X_10 0x000A
629 #define ADC_CLKPS_X_12 0x000C
630 #define ADC_CLKPS_X_14 0x000E
631 #define ADC_CLKPS_X_16 0x0010
632 #define ADC_CLKPS_X_18 0x0012
633 #define ADC_CLKPS_X_20 0x0014
634 #define ADC_CLKPS_X_22 0x0016
635 #define ADC_CLKPS_X_24 0x0018
636 #define ADC_CLKPS_X_26 0x001A
637 #define ADC_CLKPS_X_28 0x001C
638 #define ADC_CLKPS_X_30 0x001E
640 #define ADC_SMODE_SIMULTANEOUS 0x0001
641 #define ADC_SMODE_SEQUENTIAL 0x0000
643 #endif // end of F28_BMSK_H definition
Copyright 2023, Texas Instruments Incorporated