Application States and Scheduling Policies¶
The complete set of scheduling rules used by DMM consists of what we call the Global Priority Table (GPT) and the DMM Policy Table. DMM uses both tables to deduce the priority of any stack at any given time, depending on scheduled stack activities and current application states.
Global Priority Table (GPT)¶
GPT defines the relative priorities of the two stacks, assigning a priority value for all combinations of each stack activity and priority levels. GPT consists of three parameters:
Stack activity
Priority level (Normal, High, Urgent)
GPT value
When a stack schedules an RF command, a stack activity and priority level is provided with the scheduled RF command as part of the schedule parameters. This is used by DMM to perform a lookup into GPT to find the corresponding GPT value for this scheduled RF command.
Sample GPTs¶
There is a number of sample GPTs that are provided by TI in the SimpleLink CC13xx/CC26xx SDK. While the given combination of stack activities and relative priorities can be tweaked by the application developer, this policy should serve as a starting point when developing a DMM application. The sample GPTs are designed to work for most use cases of that particular combination of stacks.
Sample GPTs are available and can be found in the DMM source directory in the
SimpleLink CC13xx/CC26xx SDK: <SDK>/source/ti/dmm/dmm_priority_{stacks}.[c/h]
.
As an example, the dmm_priority_ble_wsn
sample GPT showcases the GPT for
BLE5 and WSN in the general case. It is recommended to read the associated
source code for a more detailed overview of the GPT.
Stack Activities¶
The stack activities are a set of 16-bit values mapping to a given stack’s current activity. How many stack activities a given stack has, and which numbers these are assigned/mapped to, is up to the stack developer to decide. For TI stacks that are already supporting the DMM, the activities has been predefined and can in most cases not be changed or updated by the user. See the tables below for a summery of the stack activities defined for TI provided stacks.
Stack Activity |
Numeric Value |
Description |
---|---|---|
Connection establishment |
1000 |
Activities related to establishing a connection, such as connectable advertisements and connection request events. |
Connected |
2000 |
Activities related to an active connection, such as connection events. |
Broadcasting |
3000 |
Activities related to broadcasting only, such as non-connectable advertisements. |
Observing |
4000 |
Activities related to observing only, such as scan events. |
Stack Activity |
Numeric Value |
Description |
---|---|---|
Link establishment |
1 |
Activities related MAC association events. |
Beacon TX |
2 |
Used when transmitting beacon packets |
Beacon RX |
3 |
Used while receiving beacon packets |
Frequency hopping |
4 |
Used when transmitting unicast or broadcast packets |
Network Scan |
5 |
Used while performing scan events |
Data RX/TX |
6 |
Gathers remaining transmitting and receiving type activity such as asynchronous frequency hopping and non-beacon events |
RX on in idle |
7 |
Used when leaving the receiver on in idle |
Stack Activity |
Numeric Value |
Description |
---|---|---|
Transmit |
777 |
EasyLink APIs with transmit in the name. |
Receive |
775 |
EasyLink APIs with receive in the name. |
On the other hand, when implementing a custom stack with the DMM, the user needs to define a set of stack activities to be used when later creating the GPT for the stack. The example below shows how the stack activities for BLE is defined in code.
/* BLE Activity */
typedef enum
{
DMM_BLE_CONNECTION =0x07D0,
DMM_BLE_CON_EST =0x03E8,
DMM_BLE_BROADCASTING =0x0BB8,
DMM_BLE_OBSERVING =0x0FA0,
} DMMStackActivityBLE;
After defining the activities for the custom stack, the user should make sure to use them during command scheduling in order to fully leverage the DMM GPT. On more information on how to do this, see the Communicating Stack Activity section below.
Creating a GPT¶
GPTs consists of two arrays, one for each stack. Each array defines the GPT value for all combinations of stack activities and priority levels for that stack. Each entry in the table is defined by the DMM_GLOBAL_PRIORITY() macro, which takes a stack activity, priority level and GPT value as arguments. DMM expects each stack activity to be defined in order, and per priority level in ascending order.
GPT values are chosen entirely by the developer, and can be any positive integer between 0 and 250 where a value of 250 will give highest priority. The only clear constraint of values assigned is that no GPT value can be shared between both stacks. This mean a single stack can have multiple GPT entries with the same GPT value, but not multiple GPT entries with the same GPT value between both stacks.
StackActivity activity_wsn[ACTIVITY_NUM_WSN * PRIORITY_NUM] =
{
DMM_GLOBAL_PRIORITY(DMM_WSN_RETRANSMIT, DMM_StackPNormal, 90),
DMM_GLOBAL_PRIORITY(DMM_WSN_RETRANSMIT, DMM_StackPHigh, 180),
DMM_GLOBAL_PRIORITY(DMM_WSN_RETRANSMIT, DMM_StackPUrgent, 240),
DMM_GLOBAL_PRIORITY(DMM_WSN_TRANSMIT, DMM_StackPNormal, 90),
DMM_GLOBAL_PRIORITY(DMM_WSN_TRANSMIT, DMM_StackPHigh, 180),
DMM_GLOBAL_PRIORITY(DMM_WSN_TRANSMIT, DMM_StackPUrgent, 240),
DMM_GLOBAL_PRIORITY(DMM_WSN_RECEIVE, DMM_StackPNormal, 90),
DMM_GLOBAL_PRIORITY(DMM_WSN_RECEIVE, DMM_StackPHigh, 180),
DMM_GLOBAL_PRIORITY(DMM_WSN_RECEIVE, DMM_StackPUrgent, 240),
};
The final GPT is simply both GPTs for both stacks.
GlobalTable gpt_ble_wsn[DMMPOLICY_NUM_STACKS] =
{
{
.globalTableArray = activity_ble,
.tableSize = (uint8_t)(ACTIVITY_NUM_BLE * PRIORITY_NUM),
.stackRole = DMMPolicy_StackRole_BlePeripheral,
},
{
.globalTableArray = activity_wsn,
.tableSize = (uint8_t)(ACTIVITY_NUM_WSN * PRIORITY_NUM),
.stackRole = DMMPolicy_StackRole_WsnNode,
},
};
Communicating Stack Activity¶
In order for the DMM to leverage the GPT, the stack is required to communicate the current stack activity and priority level for each scheduled activity. For software provided by TI, such as the BLE, TI-15.4, Zigbee stack and the EasyLink layer, this is internally taken care of and the user does not need to explicitly consider this.
In the custom stack use-case, the user must provide this information during command scheduling. This is done by assigning the activityInfo field in the RF_ScheduleCmdParams structure passed as an argument to the RF_scheduleCmd() API. The activityInfo need to be assigned the resulting value of combining the stack activity and expected priority level:
DMM Policy Table¶
The DMM policy table is the complete set of DMM policies for the DMM application. A DMM policy provides the following application level information:
Application state: the current application state of the stack application
Priority weight: unsigned integer for which the priority may be adjusted with, 0 being the lowest weight
Applied stack activities: set of stack activities for which the defined weight is to be applied to
Pause policy: pause policy to indicate to the application
The DMM policy table contains one or more policies, where the last DMM policy is the default policy. The order of which these policies are entered matters, as the first DMM policy which matches the current application states is returned.
Warning
The default policy has some implicit functionality compared to any other DMM policy entry. There must be different weights for both stack roles, as the default policy is used as a tie breaker in any circumstances if both stack roles end up with the same priority value.
A application can have multiple possible states. For instance, while a BLE stack application is in connected state with a high priority, a Sub-1 GHz connection might be in idle state or transmit state or might receive an acknowledgment. That is why a flag type is used for application states.
For each policy there is also an optional balanced mode which provides a way to dynamically switch priorities between the two applications. This enables time based dynamic priority switching between the applications without application state transitions.
Balanced Mode¶
In balanced mode, the “high priority” application, as defined by the weight, is given guard times to guarantee it high priority for a certain amount of time. The guard times consist of an On MIN and Off MAX guard time where the MIN time sets the minimum time window for which the application is considered high priority. The MAX time sets the maximum time the “high priority” application will be considered low priority following a switch in priorities between the applications.
Note
In balanced mode, it is important to distinguish between the priority assigned to the application in the weight field and actual priority. The priority set by the weight parameter only defines which application sets the guard time.
When the minimum guard time has passed for the “high priority” application stack, the priority may be shifted following a preemption from another application stack. At this point, the maximum off time will take effect. When the maximum off time has passed, the original stack may again regain high priority following a preemption.
Note
If any of the guard time expires, but no preemption between the two application stacks occurs, the priority will not switch. Due to this, balanced mode is best fitted for applications where one stack uses “Always in RX” or “RX on when idle” features, for example the Zigbee router + BLE peripheral example.
The diagram below shows Stack A
defining guard times, and how these
impact the co-existence between Stack A
and Stack B
.
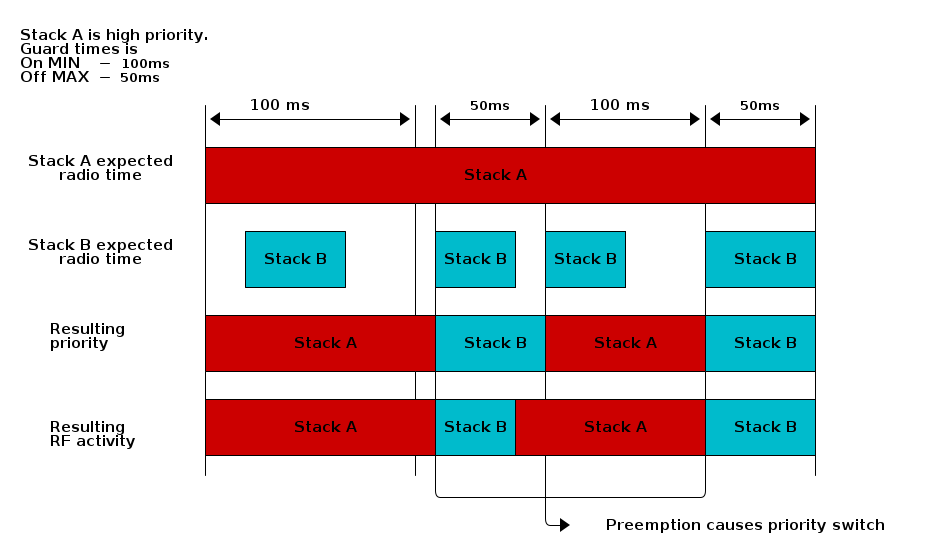
Creating A Policy Table¶
DMM policy tables are generated by SysConfig. DMM examples by the SDK already provide pre-made policy tables, and can be seen in SysConfig.
Please refer to the DMM-specific SysConfig section for more in-depth explanation on how to create a DMM policy table and DMM policies.
Updating Application State¶
Since the DMM does not hook directly into the stack, it relies on each stack’s application to inform the policy module of any changes in the stack. Such stack changes are usually signaled to the application via a message, callback, or event.
Whenever the application is signaled that its stack has changed state and it results in a chance of application state, the application should immediately notify the DMM of the state using the DMMPolicy_updateStackState API.
Registering Policy Callbacks¶
In order for the application to know when its DMM policy is changed into a paused state, the user has to register callbacks functions with the DMM policy manager. The application callbacks are registered using the DMMPolicy_registerAppCbs() API.
Note
The application callbacks should be registered at an early stage for each affected stack.
Block Mode¶
Block mode is a DMM feature that relates to a stack’s radio scheduling but that is not part of the policy table or GPT. Block mode allows the user to put a constraint on a given stack to not be allowed any radio access, no matter what the actual priority of the command would be.
To block a stack, the DMMPolicy_setBlockModeOn() API is used. The corresponding, DMMPolicy_setBlockModeOff() API is used to turn the block of again. A user can query the blocking status of a stack using the DMMPolicy_getBlockModeStatus() API.
Note
The stack being blocked using this API will experience command rejections at the expected time of execution. This means that an immediate RF command will be rejected at the time it is issued while a command scheduled into the future will not be rejected until it is time to execute.
Warning
As Block Mode while active will prevent all radio access for the given stack, no network level operations such as association of devices and/or joining of networks, will be able to complete successfully.
The block mode is binary in state which means that you can only set one blocking constraint at a time. This means a user will need to consider the current Block Mode status if there could be multiple asynchronous attempts to block/unblock a given stack.
A blocked stack will still be able to schedule commands and the current policy and GPT will be used to make scheduling decisions. This means that while a stack might have a blocking constraint set, it could still impact radio access for the other stack. This would happen in case the blocked stack’s radio commands is scheduled into the future (more then a few ms) while also being considered to be of higher priority.
Note
The Block Mode feature has been tested for end-device type applications only (TI 15.4 sensor, Zigbee End Device, BLE Peripheral). While possible to use it for other stacks and stack roles, the user would need to verify correct/expected behavior.