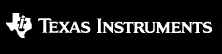 |
 |
Go to the documentation of this file.
69 #if !defined(__TMS320C28XX_CLA__)
76 #if defined(DATALOGI4_EN) || defined(DATALOGF2_EN)
80 #if defined(DATALOGF2_EN)
81 #define DATA_LOG_BUFF_SIZE 200 // Must be intergral times of (20)
82 #define DATA_SIZE_EXT 2 //
84 #define DATA_LOG_BUFF_NUM 2 // = 2 for F28002x or = 4 for other devices
86 #define DLOG_BURST_SIZE 20 // write 200 to the register for
89 #define DLOG_TRANSFER_SIZE (1 + 2* DATA_LOG_BUFF_SIZE / DLOG_BURST_SIZE)
92 #elif defined(DATALOGI4_EN)
93 #define DATA_LOG_BUFF_SIZE 200 //**20
94 #define DATA_SIZE_EXT 20 //
96 #define DATA_LOG_BUFF_NUM 4 // = 2 for F28002x or = 4 for other devices
98 #define DLOG_BURST_SIZE 20 // write 200 to the register for
101 #define DLOG_TRANSFER_SIZE 10 // [(MEM_BUFFER_SIZE/(BURST)]
102 #endif // DATALOGI4_EN
104 #define DLOG_WRAP_SIZE DLOG_TRANSFER_SIZE + 1
106 #define DATA_LOG_SCALE_FACTOR 10 // update every 10 times
115 #if defined(DATALOGF2_EN)
117 #elif defined(DATALOGI4_EN)
119 #endif // DATALOGI4_EN
124 uint16_t scaleFactor;
137 #if defined(DATALOGF2_EN)
140 #elif defined(DATALOGI4_EN)
145 #endif // DATALOGI4_EN
160 extern DATALOG_Handle DATALOGIF_init(
void *pMemory,
const size_t numBytes);
167 bool enableFlag =
false;
171 if(obj->scaleCnt >= obj->scaleFactor)
183 static inline void DATALOGIF_setPrescalar(
DATALOG_Handle handle, uint16_t prescalar)
187 obj->scaleFactor = prescalar;
200 if(obj->scaleCnt >= obj->scaleFactor)
207 #if defined(DATALOGF2_EN)
210 #elif defined(DATALOGI4_EN)
215 #endif // DATALOGI4_EN
230 uint16_t number = obj->
size - 1;
232 #if defined(DATALOGF2_EN)
237 #elif defined(DATALOGI4_EN)
243 obj->faultCount[0]++;
251 obj->faultCount[1]++;
259 obj->faultCount[2]++;
267 obj->faultCount[3]++;
269 #endif // DATALOGI4_EN
273 #endif // DATALOGI4_EN || DATALOGF2_EN
291 #endif // end of DATALOG_H definition
DATALOG_Obj datalog
Defines the DATALOG object.
struct _DATALOG_OBJ_ DATALOG_Obj
Defines the data logging (DATALOG) object.
float float32_t
Definition: sfra_f32.h:42
float32_t * iptr[4]
Input: First input pointer.
Definition: datalog.h:91
#define DATA_LOG_BUFF_NUM
Definition: datalog.h:62
struct _DATALOG_Obj_ * DATALOG_Handle
Defines the DATALOG handle.
Definition: datalog.h:101
uint16_t size
Parameter: Maximum data buffer.
Definition: datalog.h:96
float32_t datalogBuff2[400+2]
#define DATA_LOG_BUFF_SIZE
Definition: datalog.h:64
Defines the data logging (DATALOG) object.
Definition: datalog.h:89
float32_t datalogBuff1[400+2]
uint16_t cntr
Variable: Data log counter.
Definition: datalog.h:95
DATALOG_Handle datalogHandle
float32_t datalogBuff3[400+2]
float32_t datalogBuff4[400+2]
Copyright 2023, Texas Instruments Incorporated