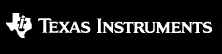 |
 |
Go to the documentation of this file.
57 #define GRAPH_BUFFER_NR 2 // Number of data arrays
59 #define GRAPH_BUFFER_SIZE 256 // Size of data arrays
61 #if (16 < GRAPH_BUFFER_SIZE && GRAPH_BUFFER_SIZE <= 32)
62 #define GRAPH_BUFFER_MASK (32-1)
63 #elif(32 < GRAPH_BUFFER_SIZE && GRAPH_BUFFER_SIZE <= 64)
64 #define GRAPH_BUFFER_MASK (64-1)
65 #elif(64 < GRAPH_BUFFER_SIZE && GRAPH_BUFFER_SIZE <= 128)
66 #define GRAPH_BUFFER_MASK (128-1)
67 #elif(128 < GRAPH_BUFFER_SIZE && GRAPH_BUFFER_SIZE <= 256)
68 #define GRAPH_BUFFER_MASK (256-1)
69 #elif(256 < GRAPH_BUFFER_SIZE && GRAPH_BUFFER_SIZE <= 512)
70 #define GRAPH_BUFFER_MASK (512-1)
71 #elif(512 < GRAPH_BUFFER_SIZE && GRAPH_BUFFER_SIZE <= 1024)
72 #define GRAPH_BUFFER_MASK (1024-1)
73 #elif(1024 < GRAPH_BUFFER_SIZE && GRAPH_BUFFER_SIZE <= 2048)
74 #define GRAPH_BUFFER_MASK (2048-1)
75 #elif(2048 < GRAPH_BUFFER_SIZE && GRAPH_BUFFER_SIZE <= 4096)
76 #define GRAPH_BUFFER_MASK (4096-1)
77 #elif(4096 < GRAPH_BUFFER_SIZE && GRAPH_BUFFER_SIZE <= 8192)
78 #define GRAPH_BUFFER_MASK (8192-1)
80 #error GRAPH_BUFFER_SIZE is outside GRAPH_BUFFER_MASK definition in graph.h
85 #define GRAPH_VARS_INIT { \
89 GRAPH_STEP_RP_CURRENT, \
93 #define GRAPH_STEP_VARS_INIT { \
224 #endif // end of GRAPH_H definition
GRAPH_BufferNR_e
Enumeration for the number of buffers.
Definition: graph.h:116
speed step response
Definition: graph.h:127
current step response
Definition: graph.h:126
float32_t spdRef_StepSize
Definition: graph.h:166
#define GRAPH_BUFFER_SIZE
Definition: graph.h:59
float float32_t
Definition: sfra_f32.h:42
volatile float32_t * pSpeed_in
Definition: graph.h:157
float32_t * pIq_ref
Definition: graph.h:163
GRAPH_Buffer_t bufferData[2]
Definition: graph.h:150
uint16_t bufferCounter
Definition: graph.h:143
void GRAPH_Data_Gather(GRAPH_Vars_t *pGraphVars, GRAPH_BufferNR_e bufferNum, float32_t gData)
Data gathering function for any iq value.
void GRAPH_generateStepResponse(GRAPH_Vars_t *pGraphVars, GRAPH_StepVars_t *pStepVars)
Sets the values to collect in a data array.
GRAPH_StepResponseMode_e
Definition: graph.h:124
uint16_t bufferTick
Definition: graph.h:145
volatile float32_t * pSpeed_ref
Definition: graph.h:161
float32_t data[256]
Definition: graph.h:136
struct _GRAPH_StepVars_t_ GRAPH_StepVars_t
void GRAPH_DATA(GRAPH_Vars_t *pGraphVars, GRAPH_StepVars_t *pStepVars)
Sets the values to collect in a data array.
float32_t * pIq_in
Definition: graph.h:159
void GRAPH_DataPointerInit(GRAPH_StepVars_t *pStepVars, volatile float32_t *pSpeed_in, float32_t *pId_in, float32_t *pIq_in, volatile float32_t *pSpeed_ref, float32_t *pId_ref, float32_t *pIq_ref)
Data gathering function for any iq value.
struct _GRAPH_Vars_t_ GRAPH_Vars_t
float32_t IdRef_Default
Definition: graph.h:168
void GRAPH_BufferInit(GRAPH_Vars_t *pGraphVars)
Init function and reset function.
float32_t IdRef_StepSize
Definition: graph.h:169
GRAPH_StepResponseMode_e bufferMode
Definition: graph.h:147
No Used.
Definition: graph.h:128
bool GRAPH_BufferIn(GRAPH_Buffer_t *pBuffer, float32_t data)
Write into the buffer.
uint16_t stepResponse
Definition: graph.h:155
Buffer define 1.
Definition: graph.h:119
uint16_t read
Definition: graph.h:137
float32_t * pId_ref
Definition: graph.h:162
float32_t spdRef_Default
Definition: graph.h:165
Buffer define 0.
Definition: graph.h:118
uint16_t write
Definition: graph.h:138
uint16_t bufferTickCounter
Definition: graph.h:144
Buffer define 3.
Definition: graph.h:121
#define GRAPH_BUFFER_NR
Definition: graph.h:57
bool GRAPH_BufferOut(GRAPH_Buffer_t *pBuffer, float32_t *pWord)
Read from the buffer.
float32_t * pId_in
Definition: graph.h:158
Buffer define 2.
Definition: graph.h:120
struct _GRAPH_Buffer_t_ GRAPH_Buffer_t
Copyright 2023, Texas Instruments Incorporated