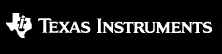 |
 |
Go to the documentation of this file.
15 #ifndef CPU_CLA_SHARED_DM_H
16 #define CPU_CLA_SHARED_DM_H
21 #if defined(F2837x_DEVICE) || defined(F28004x_DEVICE)
22 #include "F28x_Project.h"
24 #include "f28x_project.h"
31 #include "RAMP_GEN_CLA.h"
33 #include "dual_axis_servo_drive_user.h"
44 #define SETGPIO18_HIGH asm(" PUSH DP"); \
45 asm(" MOVW DP,#0x1fc"); \
46 asm(" OR @0x1,#0x0004 "); \
49 #define SETGPIO18_LOW asm(" PUSH DP"); \
50 asm(" MOVW DP,#0x1fc") ; \
51 asm(" AND @0x1,#0xfffb "); \
55 #define SETGPIO18_HIGH
80 #define CMPLXPARS_DEFAULTS { \
97 #define FCL_DEFAULTS { \
100 CMPLXPARS_DEFAULTS, \
103 RAMPGEN_CLA_DEFAULTS, \
104 FCL_PI_CONTROLLER_DEFAULTS, \
154 #define FCL_PI_MACRO(v) \
156 v.out += (v.err * v.Kerr) + v.carryOver; \
157 v.out = (v.out > v.Umax) ? v.Umax : v.out; \
158 v.out = (v.out < v.Umin) ? v.Umin : v.out; \
161 #define CLAMP_MACRO(v) \
163 v.out = (v.out > v.Umax) ? v.Umax : v.out; \
164 v.out = (v.out < v.Umin) ? v.Umin : v.out; \
171 #endif // end of CPU_CLA_SHARED_DM_H definition
FCL_PIController_t pi_iq
Definition: cpu_cla_shared_dm.h:127
float float32_t
Definition: sfra_f32.h:42
float32_t pangle
Definition: cpu_cla_shared_dm.h:125
struct _SVGEN2_t_ SVGEN2_t
struct motPars cmplxPars_t
struct _FCL_Vars_t_ FCL_Vars_t
typedefs for motorVars Variables for CLA tasks
ENC_Status_e lsw
Definition: cpu_cla_shared_dm.h:121
cmplxPars_t Q_cla
Definition: cpu_cla_shared_dm.h:123
Definition: qep_defs.h:27
QEP qep
Definition: cpu_cla_shared_dm.h:128
float32_t cosWTs
Definition: cpu_cla_shared.h:63
Definition: cpu_cla_shared.h:62
typedefs for motorVars Variables for CLA tasks
Definition: cpu_cla_shared_dm.h:119
float32_t Tc
Definition: cpu_cla_shared_dm.h:142
float32_t idErr
Definition: cpu_cla_shared.h:67
float32_t Tb
Definition: cpu_cla_shared_dm.h:141
Definition: cpu_cla_shared_dm.h:136
float32_t expVal
Definition: cpu_cla_shared.h:65
ENC_Status_e
Definition: encoder.h:86
float32_t iqErr
Definition: cpu_cla_shared.h:68
float32_t kDirect
Definition: cpu_cla_shared.h:66
RAMP_GEN_CLA rg
Definition: cpu_cla_shared_dm.h:126
float32_t tmp2
Definition: cpu_cla_shared_dm.h:144
float32_t speedWePrev
Definition: cpu_cla_shared_dm.h:124
float32_t Ta
Definition: cpu_cla_shared_dm.h:140
float32_t tmp1
Definition: cpu_cla_shared_dm.h:143
volatile struct EQEP_REGS * ptrQEP
Definition: cpu_cla_shared_dm.h:129
float32_t sinWTs
Definition: cpu_cla_shared.h:64
float32_t Ubeta
Definition: cpu_cla_shared_dm.h:139
uint32_t taskCount[4]
Definition: cpu_cla_shared_dm.h:130
float32_t Ualpha
Definition: cpu_cla_shared_dm.h:138
float32_t carryOver
Definition: cpu_cla_shared.h:69
Copyright 2023, Texas Instruments Incorporated