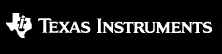 |
 |
Go to the documentation of this file.
64 #ifndef __TMS320C28XX_CLA__
75 #define MATH_Nm_TO_lbin_SF ((float32_t)(8.8507457913f))
82 #define MATH_TWO_OVER_THREE ((float32_t)(0.6666666666666666666666666667f))
89 #define MATH_ONE_OVER_THREE ((float32_t)(0.3333333333333333333333333333f))
96 #define MATH_ONE_OVER_PI ((float32_t)(0.318309886183791f))
104 #define MATH_SQRT_TWO ((float32_t)(1.414213562373095f))
112 #define MATH_SQRT_THREE ((float32_t)(1.73205080756887f))
119 #define MATH_ONE_OVER_SQRT_THREE ((float32_t)(0.5773502691896257645091487805f))
126 #define MATH_ONE_OVER_FOUR_PI ((float32_t)(0.07957747154594767f))
133 #define MATH_ONE_OVER_TWO_PI ((float32_t) (0.1591549430918954f))
140 #define MATH_PI ((float32_t)(3.1415926535897932384626433832f))
147 #define MATH_PI_PU ((float32_t)(0.5f))
154 #define MATH_TWO_PI ((float32_t)(6.283185307179586f))
161 #define MATH_TWO_PI_PU ((float32_t)(1.0f))
168 #define MATH_FOUR_PI ((float32_t)(12.56637061435917f))
175 #define MATH_FOUR_PI_PU ((float32_t)(2.0f))
182 #define MATH_PI_OVER_TWO ((float32_t)(1.570796326794897f))
189 #define MATH_PI_OVER_TWO_PU ((float32_t)(0.25f))
197 #define MATH_PI_OVER_THREE ((float32_t)(1.047197551196598f))
204 #define MATH_PI_OVER_FOUR ((float32_t)(0.785398163397448f))
211 #define MATH_PI_OVER_FOUR_PU ((float32_t)(0.125f))
318 #ifdef __TMS320C28XX_CLA__
319 #pragma FUNC_ALWAYS_INLINE(MATH_incrAngle)
330 angleNew_rad = angle_rad + angleDelta_rad;
347 return(angleNew_rad);
377 out = (out > max) ? max : out;
378 out = (out < min) ? min : out;
415 #define MATH_SIX_PI ((float32_t)(18.8495559215f))
422 #define MATH_SQRTTHREE_OVER_TWO ((float32_t)(0.8660254038f))
434 #ifdef __TMS320C28XX_CLA__
450 #ifdef __TMS320C28XX_TMU__
451 y = pow(x1->
real,2) + pow(x1->
imag,2);
455 #endif // __TMS320C28XX_TMU__
459 #endif // __TMS320C28XX_CLA__
cplx_float_t value[2]
Definition: math.h:394
Defines a two element vector.
Definition: math.h:218
float float32_t
Definition: sfra_f32.h:42
#define MATH_TWO_PI
Defines 2*pi.
Definition: math.h:154
Defines a three element vector.
Definition: math.h:403
static void MATH_add_cc(cplx_float_t *x1, cplx_float_t *x2, cplx_float_t *y)
Definition: math.h:485
Defines a two element vector.
Definition: math.h:391
static float32_t MATH_incrAngle(const float32_t angle_rad, const float32_t angleDelta_rad)
Increments an angle value and handles wrap-around.
Definition: math.h:323
#define MATH_PI
Defines pi.
Definition: math.h:140
cplx_float_t value[3]
Definition: math.h:406
struct _MATH_Vec3_ MATH_Vec3
Defines a three element vector.
MATH_Vec2 MATH_vec2
Definition: math.h:223
static void MATH_mult_rc(float32_t x1, cplx_float_t *x2, cplx_float_t *y)
Definition: math.h:501
static void MATH_sub_cr(cplx_float_t *x1, float32_t x2, cplx_float_t *y)
Definition: math.h:525
double double_t
Defines the portable data type for 64 bit, signed floating-point data.
Definition: types.h:162
static void MATH_sub_cc(cplx_float_t *x1, cplx_float_t *x2, cplx_float_t *y)
Definition: math.h:493
static float32_t MATH_abs(const float32_t in)
Finds the absolute value.
Definition: math.h:247
float32_t value[3]
Definition: math.h:232
static float32_t MATH_max(const float32_t in1, const float32_t in2)
Finds the maximum value between the twp input values.
Definition: math.h:270
static float32_t MATH_sign(const float32_t in)
Definition: math.h:463
struct _MATH_Vec2_ MATH_Vec2
Defines a two element vector.
static void MATH_add_rc(float32_t x1, cplx_float_t *x2, cplx_float_t *y)
Definition: math.h:509
float_t imag
Definition: types.h:308
static float32_t MATH_sat(const float32_t in, const float32_t max, const float32_t min)
Saturates the input value between the minimum and maximum values.
Definition: math.h:364
static void MATH_mult_cc(cplx_float_t *x1, cplx_float_t *x2, cplx_float_t *y)
Definition: math.h:477
struct _MATH_cplx_vec2_ MATH_cplx_vec2
Defines a two element vector.
Define the complex data type for float real and imaginary components.
Definition: types.h:305
MATH_Vec3 MATH_vec3
Definition: math.h:235
static void MATH_sub_rc(float32_t x1, cplx_float_t *x2, cplx_float_t *y)
Definition: math.h:517
static float32_t cAbsSq(const cplx_float_t *x1)
Finds the absolute value.
Definition: math.h:446
float_t real
Definition: types.h:307
struct _MATH_cplx_vec3_ MATH_cplx_vec3
Defines a three element vector.
static float32_t MATH_min(const float32_t in1, const float32_t in2)
Finds the minimum value between the twp input values.
Definition: math.h:294
float32_t value[2]
Definition: math.h:220
Defines a three element vector.
Definition: math.h:230
Copyright 2023, Texas Instruments Incorporated