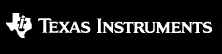 |
 |
Go to the documentation of this file.
64 #ifdef __TMS320C28XX_CLA__
65 #include "libraries/math/src/float/CLAmath.h"
73 #include "userParams.h"
93 #define CAL_BUF_NUM 13
129 uint16_t hallIndexNow;
130 uint16_t hallIndexPre;
131 uint16_t hallIndexFlag;
214 uint32_t hallState = 0;
216 hallState = GPIO_readPin(obj->
gpioHallU);
217 hallState += GPIO_readPin(obj->
gpioHallV)<<1;
218 hallState += GPIO_readPin(obj->
gpioHallW)<<2;
220 return(hallState & 0x0007);
233 if(obj->hallIndexNow != obj->hallIndexPre)
235 obj->hallIndexPre = obj->hallIndexNow;
237 if(obj->hallIndexFlag == 0)
239 obj->thetaIndexBuff[obj->hallIndexNow] = angle;
241 if(obj->hallIndexNow == 0x01)
243 obj->hallIndexFlag = 1;
248 obj->thetaIndexBuff[6 + obj->hallIndexNow] = angle;
250 if(obj->hallIndexNow == 0x01)
252 obj->hallIndexFlag = 0;
256 obj->thetaCalBuff[obj->hallIndexNow] = 0.5f *
257 (obj->thetaIndexBuff[obj->hallIndexNow] +
258 obj->thetaIndexBuff[6 + obj->hallIndexNow]);
303 #define HALL_calcAngle HALL_run
392 #endif //end of _HALL_H_ definition
float32_t speedHall_Hz
Definition: hall.h:105
HALL_Status_e hallStatus
Definition: hall.h:124
uint16_t hallIndexPrev
Definition: hall.h:121
void HALL_resetParams(HALL_Handle handle)
Resets the hall estimator parameters.
uint32_t timeCountPWM
Definition: hall.h:108
float32_t thetaDelta_pu
Definition: hall.h:115
float32_t thetaBuff[7]
Definition: hall.h:112
float float32_t
Definition: sfra_f32.h:42
#define MATH_TWO_PI
Defines 2*pi.
Definition: math.h:154
struct _HALL_Obj_ HALL_Obj
Defines the HALL controller object.
float32_t thetaDelta_rad
Definition: hall.h:114
static void HALL_setForceAngleAndIndex(HALL_Handle handle, float32_t speedRef)
Sets the force angle and index for next step of the hall estimator.
Definition: hall.h:268
float32_t speedCAP_Hz
Definition: hall.h:103
#define MATH_PI
Defines pi.
Definition: math.h:140
void HALL_setGPIOs(HALL_Handle handle, const uint16_t gpioHallU, const uint16_t gpioHallV, const uint16_t gpioHallW)
Sets GPIO for hall sensors input.
float32_t speedPWM_Hz
Definition: hall.h:104
uint16_t hallPrev[7]
Definition: hall.h:122
uint32_t timeCount[2]
Definition: hall.h:109
Defines the HALL controller object.
Definition: hall.h:99
float32_t pwmScaler
Definition: hall.h:102
void HALL_setAngleBuf(HALL_Handle handle, const float32_t *ptrAngleBuf)
Sets the angle buffer value for hall estimator.
float32_t thetaHall_pu
Definition: hall.h:116
float32_t capScaler
Definition: hall.h:101
HALL_Handle HALL_init(void *pMemory, const size_t numBytes)
Initializes the HALL controller.
static void HALL_setTimeStamp(HALL_Handle handle, const uint32_t timeStamp)
Sets a value to the time stamp for the hall estimator.
Definition: hall.h:190
uint16_t hallIndex
Definition: hall.h:120
uint16_t hallDirection
Definition: hall.h:123
uint32_t timeStampCAP
Definition: hall.h:107
static float32_t HALL_getAngle_rad(HALL_Handle handle)
Gets the angle from the hall estimator, rad.
Definition: hall.h:201
HALL_Status_e
Definition: hall.h:84
static void HALL_setSpeed_Hz(HALL_Handle handle, const float32_t speedHall_Hz)
Sets a value to the speed for the hall estimator.
Definition: hall.h:178
uint16_t gpioHallU
Definition: hall.h:117
uint16_t gpioHallW
Definition: hall.h:119
static uint16_t HALL_getInputState(HALL_Handle handle)
Gets the hall sensors input GPIO state.
Definition: hall.h:210
struct _HALL_Obj_ * HALL_Handle
Defines the HALL handle.
Definition: hall.h:138
static void HALL_run(HALL_Handle handle, float32_t speedRef)
Definition: hall.h:305
float32_t speedSwitch_Hz
Definition: hall.h:106
Defines a structure for the user parameters.
Definition: include/userParams.h:98
float32_t thetaHall_rad
Definition: hall.h:113
uint32_t timeCountMax
Definition: hall.h:110
uint16_t gpioHallV
Definition: hall.h:118
static float32_t HALL_getSpeed_Hz(HALL_Handle handle)
Gets the feedback speed from hall estimator.
Definition: hall.h:168
void HALL_setParams(HALL_Handle handle, const USER_Params *pUserParams)
Sets the hall estimator parameters.
Copyright 2023, Texas Instruments Incorporated