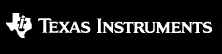 |
 |
Go to the documentation of this file.
25 #if defined(F2837x_DEVICE) || defined(F28004x_DEVICE)
26 #include "F28x_Project.h"
28 #include "f28x_project.h"
52 #include "pid_grando.h"
57 #if(SPD_CNTLR == SPD_NLPID_CNTLR)
58 #include "DCL_NLPID.h"
61 #include "motor_drive_settings.h"
62 #include "motor_drive_user.h"
65 #define PI 3.14159265358979
95 #define FCL_PARS_DEFAULTS { \
113 #if(SPD_CNTLR == SPD_PID_CNTLR)
117 #define MOTOR_DEFAULTS { \
122 (float32_t)(M_BASE_FREQ), \
123 (float32_t)(M_POLES/2.0), \
124 (float32_t)(0.25/M_ENC_SLOTS), \
126 (0.001 / M_ISR_FREQUENCY), \
131 (M_STARTUP_FREQ/M_BASE_FREQ), \
132 (M_MAXIMUM_FREQ/M_BASE_FREQ), \
188 (uint32_t *)(M_U_PWM_BASE + EPWM_O_CMPA), \
189 (uint32_t *)(M_V_PWM_BASE + EPWM_O_CMPA), \
190 (uint32_t *)(M_W_PWM_BASE + EPWM_O_CMPA), \
192 (M_IU_ADCRESULT_BASE + M_IU_ADC_PPB_NUM + ADC_O_PPB1RESULT), \
193 (M_IV_ADCRESULT_BASE + M_IV_ADC_PPB_NUM + ADC_O_PPB1RESULT), \
194 (M_IW_ADCRESULT_BASE + M_IW_ADC_PPB_NUM + ADC_O_PPB1RESULT), \
195 (M_VDC_ADCRESULT_BASE + M_VDC_ADC_PPB_NUM + ADC_O_PPB1RESULT), \
197 (union ADCINTFLG_REG *)(M_IW_ADC_BASE + ADC_INTFLG_ADCINT1), \
198 (struct EQEP_REGS *)(M_QEP_BASE), \
207 SPEED_MEAS_QEP_DEFAULTS, \
209 {PID_TERM_DEFAULTS, PID_PARAM_DEFAULTS, PID_DATA_DEFAULTS}, \
210 PI_CONTROLLER_DEFAULTS, \
212 FCL_CMPLXCTRL_DEFAULTS, \
213 FCL_CMPLXCTRL_DEFAULTS, \
243 2048 + 6.0*4096/M_ADC_SCALE_CURRENT, \
244 2048 - 6.0*4096/M_ADC_SCALE_CURRENT, \
245 2048 + 36.0*4096/M_ADC_SCALE_VOLATGE, \
259 #endif // (SPD_CNTLR == SPD_PID_CNTLR)
261 #if(SPD_CNTLR == SPD_DCL_CNTLR)
265 #define MOTOR_DEFAULTS { \
270 (float32_t)M_BASE_FREQ, \
271 (float32_t)(M_POLES/2.0), \
272 (float32_t)(0.25/M_ENC_SLOTS), \
274 (0.001 / M_ISR_FREQUENCY), \
279 (M_STARTUP_FREQ/M_BASE_FREQ), \
280 (M_MAXIMUM_FREQ/M_BASE_FREQ), \
336 (uint32_t *)(M_U_PWM_BASE + EPWM_O_CMPA), \
337 (uint32_t *)(M_V_PWM_BASE + EPWM_O_CMPA), \
338 (uint32_t *)(M_W_PWM_BASE + EPWM_O_CMPA), \
340 (M_IU_ADCRESULT_BASE + M_IU_ADC_PPB_NUM + ADC_O_PPB1RESULT), \
341 (M_IV_ADCRESULT_BASE + M_IV_ADC_PPB_NUM + ADC_O_PPB1RESULT), \
342 (M_IW_ADCRESULT_BASE + M_IW_ADC_PPB_NUM + ADC_O_PPB1RESULT), \
343 (M_VDC_ADCRESULT_BASE + M_VDC_ADC_PPB_NUM + ADC_O_PPB1RESULT), \
345 (union ADCINTFLG_REG *)(M_IW_ADC_BASE + ADC_INTFLG_ADCINT1), \
346 (struct EQEP_REGS *)(M_QEP_BASE), \
355 SPEED_MEAS_QEP_DEFAULTS, \
360 FCL_CMPLXCTRL_DEFAULTS, \
361 FCL_CMPLXCTRL_DEFAULTS, \
391 2048 + 6.0*4096/M_ADC_SCALE_CURRENT, \
392 2048 - 6.0*4096/M_ADC_SCALE_CURRENT, \
393 2048 + 36.0*4096/M_ADC_SCALE_VOLATGE, \
407 #endif // (SPD_CNTLR == SPD_DCL_CNTLR)
409 #if(SPD_CNTLR == SPD_NLPID_CNTLR)
413 #define MOTOR_DEFAULTS { \
418 (float32_t)M_BASE_FREQ, \
419 (float32_t)(M_POLES/2.0), \
420 (float32_t)(0.25/M_ENC_SLOTS), \
422 (0.001 / M_ISR_FREQUENCY), \
427 (M_STARTUP_FREQ/M_BASE_FREQ), \
428 (M_MAXIMUM_FREQ/M_BASE_FREQ), \
484 (uint32_t *)(M_U_PWM_BASE + EPWM_O_CMPA), \
485 (uint32_t *)(M_V_PWM_BASE + EPWM_O_CMPA), \
486 (uint32_t *)(M_W_PWM_BASE + EPWM_O_CMPA), \
488 (M_IU_ADCRESULT_BASE + M_IU_ADC_PPB_NUM + ADC_O_PPB1RESULT), \
489 (M_IV_ADCRESULT_BASE + M_IV_ADC_PPB_NUM + ADC_O_PPB1RESULT), \
490 (M_IW_ADCRESULT_BASE + M_IW_ADC_PPB_NUM + ADC_O_PPB1RESULT), \
491 (M_VDC_ADCRESULT_BASE + M_VDC_ADC_PPB_NUM + ADC_O_PPB1RESULT), \
493 (union ADCINTFLG_REG *)(M_IW_ADC_BASE + ADC_INTFLG_ADCINT1), \
494 (struct EQEP_REGS *)(M_QEP_BASE), \
503 SPEED_MEAS_QEP_DEFAULTS, \
509 FCL_CMPLXCTRL_DEFAULTS, \
510 FCL_CMPLXCTRL_DEFAULTS, \
540 2048 + 6.0*4096/M_ADC_SCALE_CURRENT, \
541 2048 - 6.0*4096/M_ADC_SCALE_CURRENT, \
542 2048 + 36.0*4096/M_ADC_SCALE_VOLATGE, \
556 #endif // (SPD_CNTLR == SPD_NLPI_CNTLR)
654 SPEED_MEAS_QEP
speed;
656 #if(SPD_CNTLR == SPD_PID_CNTLR)
659 #endif // (SPD_CNTLR == SPD_PID_CNTLR)
661 #if(SPD_CNTLR == SPD_DCL_CNTLR)
664 #endif // (SPD_CNTLR == SPD_DCL_CNTLR)
666 #if(SPD_CNTLR == SPD_NLPID_CNTLR)
669 #endif // (SPD_CNTLR == SPD_NLPI_CNTLR)
730 const uint32_t pwmBaseU,
731 const uint32_t pwmBaseV,
732 const uint32_t pwmBaseW);
735 const uint32_t qepBaseA);
738 const uint32_t adcResultBaseV, ADC_PPBNumber adcV_PPBNum,
739 const uint32_t adcResultBaseW, ADC_PPBNumber adcW_PPBNum);
742 const uint32_t adcResultBaseU, ADC_PPBNumber adcU_PPBNum,
743 const uint32_t adcResultBaseV, ADC_PPBNumber adcV_PPBNum,
744 const uint32_t adcResultBaseW, ADC_PPBNumber adcW_PPBNum);
818 register float32_t clarke1Alpha, clarke1Beta;
819 register float32_t park1Sine, park1Cosine;
822 park1Sine = __sinpuf32(pMotor->
pangle);
823 park1Cosine = __cospuf32(pMotor->
pangle);
828 #if(DRIVER_MODULE == BITFIELD_MODE)
831 while(ADC_getInterruptStatus(pMotor->
adcBaseW, ADC_INT_NUMBER1) == 0);
836 clarke1Beta = ((clarke1Alpha +
838 * pMotor->
FCL_params.adcPPBScale))) * ONEbySQRT3);
844 ((clarke1Beta * park1Cosine) - (clarke1Alpha * park1Sine));
847 ((clarke1Alpha * park1Cosine) + (clarke1Beta * park1Sine));
858 svgen2.Ualpha = ((pMotor->
cmplx_Id.
out * park1Cosine) -
861 svgen2.Ubeta = ((pMotor->
cmplx_Iq.
out * park1Cosine) +
867 svgen2.Tb = (svgen2.Ubeta - svgen2.Ualpha) / 2;
868 svgen2.Tc = svgen2.Tb - svgen2.Ubeta;
870 svgen2.tmp2 = __fmax(__fmax(svgen2.Ualpha, svgen2.Tc), svgen2.Tb);
871 svgen2.tmp2 += __fmin(__fmin(svgen2.Ualpha, svgen2.Tc), svgen2.Tb);
877 *(pMotor->
pwmCompA) = (uint32_t)(svgen2.Tc + svgen2.tmp1);
878 *(pMotor->
pwmCompB) = (uint32_t)(svgen2.Ualpha + svgen2.tmp1);
879 *(pMotor->
pwmCompC) = (uint32_t)(svgen2.Tb + svgen2.tmp1);
953 register float32_t clarke1Alpha, clarke1Beta;
954 register float32_t park1Sine, park1Cosine;
957 park1Sine = __sinpuf32(pMotor->
pangle);
958 park1Cosine = __cospuf32(pMotor->
pangle);
963 #if(DRIVER_MODULE == BITFIELD_MODE)
966 while(ADC_getInterruptStatus(pMotor->
adcBaseW, ADC_INT_NUMBER1) == 0);
972 clarke1Beta = ((clarke1Alpha +
974 * pMotor->
FCL_params.adcPPBScale))) *ONEbySQRT3);
980 ((clarke1Beta * park1Cosine) - (clarke1Alpha * park1Sine));
983 ((clarke1Alpha * park1Cosine) + (clarke1Beta * park1Sine));
993 svgen2.Ualpha = ((pMotor->
cmplx_Id.
out * park1Cosine) -
996 svgen2.Ubeta = ((pMotor->
cmplx_Iq.
out * park1Cosine) +
1002 svgen2.Tb = (svgen2.Ubeta - svgen2.Ualpha) / 2.0;
1003 svgen2.Tc = svgen2.Tb - svgen2.Ubeta;
1005 svgen2.tmp2 = __fmax(__fmax(svgen2.Ualpha, svgen2.Tc), svgen2.Tb);
1006 svgen2.tmp2 += __fmin(__fmin(svgen2.Ualpha, svgen2.Tc), svgen2.Tb);
1012 *(pMotor->
pwmCompA) = (uint32_t)(svgen2.Tc + svgen2.tmp1);
1013 *(pMotor->
pwmCompB) = (uint32_t)(svgen2.Ualpha + svgen2.tmp1);
1014 *(pMotor->
pwmCompC) = (uint32_t)(svgen2.Tb + svgen2.tmp1);
1079 #if(DRIVER_MODULE == BITFIELD_MODE)
1136 pMotor->
pQEPRegs->QEPCTL.bit.IEI = 0;
1174 if(pMotor->
pQEPRegs->QEPSTS.all & 0x000C)
1179 pMotor->
pQEPRegs->QEPSTS.all = 0x000C;
1188 #define EQEP_POSCNT_INIT_NOTHING 0U
1189 #define EQEP_POSCNT_INIT_RISING_EDGE 0x0200U
1190 #define EQEP_POSCNT_INIT_FALLING_EDGE 0x0300U
1197 ASSERT(EQEP_isBaseValid(base));
1202 HWREGH(base + EQEP_O_QEPCTL) = (HWREGH(base + EQEP_O_QEPCTL) &
1203 ~(EQEP_QEPCTL_IEI_M)) | initMode;
1211 uint32_t qepBase = (uint32_t)pMotor->
pQEPRegs;
1221 EQEP_getPositionLatch(qepBase);
1235 if (EQEP_getInterruptStatus(qepBase) & EQEP_INT_INDEX_EVNT_LATCH)
1238 EQEP_setInitialPosition(qepBase,
1239 EQEP_getIndexPositionLatch(qepBase));
1255 EQEP_setPosition(qepBase, 0);
1256 EQEP_clearInterruptStatus(qepBase, EQEP_INT_INDEX_EVNT_LATCH);
1270 uint32_t qepBase = (uint32_t)pMotor->
pQEPRegs;
1275 if (EQEP_getInterruptStatus(qepBase) & EQEP_INT_INDEX_EVNT_LATCH)
1278 EQEP_clearInterruptStatus(qepBase, EQEP_INT_INDEX_EVNT_LATCH);
1283 if (EQEP_getInterruptStatus(qepBase) & EQEP_INT_UNIT_TIME_OUT)
1286 EQEP_clearInterruptStatus(qepBase, EQEP_INT_UNIT_TIME_OUT);
1291 if(EQEP_getStatus(qepBase) &
1292 (EQEP_STS_CAP_OVRFLW_ERROR | EQEP_STS_CAP_DIR_ERROR))
1297 EQEP_clearStatus(qepBase,
1298 (EQEP_STS_CAP_OVRFLW_ERROR | EQEP_STS_CAP_DIR_ERROR));
volatile uint32_t * pwmCompA
Definition: fcl_cpu_cla_dm.h:348
float32_t ref
Definition: fcl_cmplx_ctrl.h:24
DCL_PI dcl_pos
Definition: fcl_foc_cpu.h:663
#define QEP_FLAG_IEL_EVENT
Definition: qep_defs.h:21
float32_t Kp
Definition: fcl_cmplx_ctrl.h:29
uint32_t FCL_getSwVersion(void)
static void FCL_runCCSyn(FCL_cmplxCtrl_t *pId, FCL_cmplxCtrl_t *pIq)
Definition: fcl_foc_cpu.h:795
volatile uint32_t * pwmCompC
Definition: fcl_cpu_cla_dm.h:350
RAMPGEN rg
Definition: fcl_foc_cpu.h:647
volatile uint32_t curB_PPBRESULT
Definition: fcl_cpu_cla_dm.h:353
float32_t IdRefDelta
Definition: fcl_foc_cpu.h:585
void FCL_initADC_2In(MOTOR_Vars_t *pMotor, uint32_t adcBaseW, const uint32_t adcResultBaseV, ADC_PPBNumber adcV_PPBNum, const uint32_t adcResultBaseW, ADC_PPBNumber adcW_PPBNum)
uint16_t speedLoopPrescaler
Definition: fcl_cpu_cla_dm.h:385
uint16_t focCycleCountMax
Definition: fcl_foc_cpu.h:685
DCL_NLPID nlpid_spd
Definition: fcl_foc_cpu.h:667
float float32_t
Definition: sfra_f32.h:42
float32_t ctrlPosOut
Definition: fcl_foc_cpu.h:624
float32_t Rq
Definition: fcl_foc_cpu.h:82
float32_t IdRefPrev
Definition: fcl_foc_cpu.h:581
float32_t invZbase
Definition: fcl_foc_cpu.h:87
float32_t fbk
Definition: fcl_cmplx_ctrl.h:25
static void FCL_runPICtrl(MOTOR_Vars_t *pMotor)
Definition: fcl_foc_cpu.h:816
float32_t posMechScaler
Definition: fcl_foc_cpu.h:569
PWMUpdateMode_e pwmUpdateMode
Definition: fcl_foc_cpu.h:713
float32_t Lq
Definition: fcl_foc_cpu.h:84
CtrlState_e ctrlStateFdb
Definition: fcl_foc_cpu.h:716
float32_t BemfK
Definition: fcl_cpu_cla.h:93
float32_t IdRefSet
Definition: fcl_foc_cpu.h:578
float32_t Rd
Definition: fcl_cpu_cla.h:84
uint16_t alignCntr
Definition: fcl_cpu_cla_dm.h:387
static void FCL_readCount(MOTOR_Vars_t *pMotor)
Definition: fcl_foc_cpu.h:749
PID_CONTROLLER pid_spd
Definition: fcl_cpu_cla_dm.h:368
float32_t Ld
Definition: fcl_cpu_cla.h:86
uint16_t drvClearFaultGPIO
Definition: fcl_cpu_cla_dm.h:397
volatile uint32_t volDC_PPBRESULT
Definition: fcl_cpu_cla_dm.h:355
static void FCL_resetController(MOTOR_Vars_t *pMotor)
Definition: fcl_foc_cpu.h:760
uint16_t alignCnt
Definition: fcl_cpu_cla_dm.h:388
float32_t xErr
Definition: fcl_cmplx_ctrl.h:39
uint16_t faultTimesCount
Definition: fcl_foc_cpu.h:708
uint16_t fclCycleCountMax
Definition: fcl_cpu_cla_dm.h:383
volatile struct EQEP_REGS * pQEPRegs
Definition: fcl_foc_cpu.h:645
float32_t BemfK
Definition: fcl_foc_cpu.h:91
float32_t baseFreq
Definition: fcl_cpu_cla_dm.h:311
float32_t pangle
Definition: fcl_foc_cpu.h:621
PI_CONTROLLER pi_pos
Definition: fcl_cpu_cla_dm.h:367
float32_t IdRef_run
Definition: fcl_cpu_cla_dm.h:322
float32_t ctrlSpeedRef
Definition: fcl_foc_cpu.h:590
#define IEI_RISING
Definition: f28x_bmsk.h:508
void FCL_initQEP(MOTOR_Vars_t *pMotor, const uint32_t qepBaseA)
float32_t currentLimit
Definition: fcl_cpu_cla_dm.h:318
float32_t cosWTs
Definition: fcl_cmplx_ctrl.h:35
float32_t expVal
Definition: fcl_cmplx_ctrl.h:37
Definition: fcl_cmplx_ctrl.h:22
uint32_t pwmBaseU
Definition: fcl_foc_cpu.h:630
uint16_t lsw2EntryFlag
Definition: fcl_foc_cpu.h:688
float32_t IdRef_start
Definition: fcl_cpu_cla_dm.h:321
float32_t focExecutionTime_us
Definition: fcl_foc_cpu.h:678
float32_t kDirect
Definition: fcl_cmplx_ctrl.h:38
FCL_cmplxCtrl_t cmplx_Id
Definition: fcl_foc_cpu.h:671
float32_t IqRefDelta
Definition: fcl_foc_cpu.h:586
float32_t positionRef
Definition: fcl_cpu_cla_dm.h:327
float32_t speedRefMax
Definition: fcl_foc_cpu.h:577
float32_t ctrlIqRef
Definition: fcl_foc_cpu.h:589
float32_t IdRefError
Definition: fcl_foc_cpu.h:583
uint16_t posPtr
Definition: fcl_cpu_cla_dm.h:390
CtrlState_e ctrlStateUse
Definition: fcl_foc_cpu.h:715
float32_t carrierMid
Definition: fcl_cpu_cla.h:78
static void FCL_runQEPPosEst(MOTOR_Vars_t *pMotor)
Definition: fcl_foc_cpu.h:1083
float32_t currentBs
Definition: fcl_cpu_cla_dm.h:336
typedefs for motorVars
Definition: fcl_cpu_cla_dm.h:305
float32_t lsw1Speed
Definition: fcl_cpu_cla_dm.h:328
float32_t offset_currentAs
Definition: fcl_cpu_cla_dm.h:331
float32_t Lq
Definition: fcl_cpu_cla.h:87
float32_t Umax
Definition: fcl_cmplx_ctrl.h:33
float32_t IdRef
Definition: fcl_cpu_cla_dm.h:323
uint16_t offsetDoneFlag
Definition: fcl_foc_cpu.h:689
PARK park
Definition: fcl_cpu_cla_dm.h:362
float32_t Rq
Definition: fcl_cpu_cla.h:85
float32_t currentCs
Definition: fcl_cpu_cla_dm.h:337
uint16_t faultStatusPrev
Definition: fcl_foc_cpu.h:707
float32_t wccQ
Definition: fcl_foc_cpu.h:89
RMPCNTL rc
Definition: fcl_cpu_cla_dm.h:359
float32_t IqRefPrev
Definition: fcl_foc_cpu.h:582
float32_t voltageLimit
Definition: fcl_cpu_cla_dm.h:317
float32_t Vdcbus
Definition: fcl_foc_cpu.h:90
float32_t IqRefError
Definition: fcl_foc_cpu.h:584
float32_t Ld
Definition: fcl_foc_cpu.h:83
float32_t IqRef_start
Definition: fcl_foc_cpu.h:597
float32_t Umin
Definition: fcl_cmplx_ctrl.h:34
float32_t Wbase
Definition: fcl_foc_cpu.h:92
float32_t wccD
Definition: fcl_foc_cpu.h:88
uint16_t posPtrMax
Definition: fcl_cpu_cla_dm.h:389
float32_t speedWePrev
Definition: fcl_foc_cpu.h:620
float32_t fclUpdateLatency_us
Definition: fcl_foc_cpu.h:677
ENC_Status_e lsw
Definition: fcl_foc_cpu.h:717
float32_t polePairs
Definition: fcl_foc_cpu.h:568
float32_t speedRef
Definition: fcl_cpu_cla_dm.h:326
uint16_t currentThreshLo
Definition: fcl_cpu_cla_dm.h:393
float32_t Ki
Definition: fcl_cmplx_ctrl.h:30
float32_t out
Definition: fcl_cmplx_ctrl.h:27
float32_t wccQ
Definition: fcl_cpu_cla.h:91
float32_t wccD
Definition: fcl_cpu_cla.h:90
IPARK ipark
Definition: fcl_cpu_cla_dm.h:363
float32_t Rd
Definition: fcl_foc_cpu.h:81
SVGEN svgen
Definition: fcl_cpu_cla_dm.h:373
float32_t currentAs
Definition: fcl_cpu_cla_dm.h:335
uint16_t fclClrCntr
Definition: fcl_cpu_cla_dm.h:382
#define QEP_FLAG_UTO_EVENT
Definition: qep_defs.h:22
static void FCL_runComplexCtrlWrap(MOTOR_Vars_t *pMotor)
Definition: fcl_foc_cpu.h:1025
float32_t sinWTs
Definition: fcl_cmplx_ctrl.h:36
uint16_t focClrCntr
Definition: fcl_foc_cpu.h:684
ENC_Status_e
Definition: encoder.h:86
float32_t adcPPBScale
Definition: fcl_foc_cpu.h:77
float32_t IqRefSet
Definition: fcl_foc_cpu.h:579
float32_t KerrOld
Definition: fcl_cmplx_ctrl.h:32
float32_t Vdcbus
Definition: fcl_cpu_cla.h:92
float32_t adcScale
Definition: fcl_cpu_cla_dm.h:341
uint32_t posCntr
Definition: fcl_cpu_cla_dm.h:307
uint32_t posCntrMax
Definition: fcl_cpu_cla_dm.h:308
static void FCL_runQEPPosEstWrap(MOTOR_Vars_t *pMotor)
Definition: fcl_foc_cpu.h:1147
CLARKE clarke
Definition: fcl_cpu_cla_dm.h:361
float32_t carrierMid
Definition: fcl_foc_cpu.h:76
uint16_t drvEnableGateGPIO
Definition: fcl_cpu_cla_dm.h:395
uint16_t drvFaultTripGPIO
Definition: fcl_cpu_cla_dm.h:396
uint16_t currentThreshHi
Definition: fcl_cpu_cla_dm.h:392
struct _FCL_Parameters_t_ FCL_Parameters_t
typedefs for motorVars
float32_t Vdcbus
Definition: fcl_cpu_cla_dm.h:375
float32_t speedWe
Definition: fcl_foc_cpu.h:619
DCL_NLPID nlpid_pos
Definition: fcl_foc_cpu.h:668
uint16_t clearFaultFlag
Definition: fcl_foc_cpu.h:709
float32_t cmidsqrt3
Definition: fcl_foc_cpu.h:78
void FCL_initADC_3In(MOTOR_Vars_t *pMotor, uint32_t adcBaseW, const uint32_t adcResultBaseU, ADC_PPBNumber adcU_PPBNum, const uint32_t adcResultBaseV, ADC_PPBNumber adcV_PPBNum, const uint32_t adcResultBaseW, ADC_PPBNumber adcW_PPBNum)
float32_t VdcbusMax
Definition: fcl_cpu_cla_dm.h:376
uint32_t pwmBaseV
Definition: fcl_foc_cpu.h:631
uint32_t isrTicker
Definition: fcl_cpu_cla_dm.h:379
uint16_t speedLoopCount
Definition: fcl_cpu_cla_dm.h:386
float32_t posSlewRate
Definition: fcl_cpu_cla_dm.h:309
static void FCL_runPI(FCL_cmplxCtrl_t *pPI)
Definition: fcl_foc_cpu.h:784
volatile uint32_t curA_PPBRESULT
Definition: fcl_cpu_cla_dm.h:352
float32_t offset_currentBs
Definition: fcl_cpu_cla_dm.h:332
Definition: fcl_cpu_cla.h:77
volatile union ADCINTFLG_REG * pADCIntFlag
Definition: fcl_foc_cpu.h:644
SPEED_MEAS_QEP speed
Definition: fcl_cpu_cla_dm.h:364
uint16_t fclCycleCount
Definition: fcl_foc_cpu.h:682
uint16_t faultStatusFlag
Definition: fcl_foc_cpu.h:706
float32_t VdcbusMin
Definition: fcl_cpu_cla_dm.h:377
void FCL_initPWM(MOTOR_Vars_t *pMotor, const uint32_t pwmBaseU, const uint32_t pwmBaseV, const uint32_t pwmBaseW)
#define EQEP_POSCNT_INIT_RISING_EDGE
poscnt=posinit @ QEPI rise
Definition: fcl_foc_cpu_dm.h:752
float32_t posMechTheta
Definition: fcl_cpu_cla_dm.h:346
float32_t IqRef
Definition: fcl_cpu_cla_dm.h:324
float32_t Ts
Definition: fcl_cpu_cla_dm.h:314
float32_t maxModIndex
Definition: fcl_cpu_cla_dm.h:315
float32_t voltageScale
Definition: fcl_cpu_cla_dm.h:340
uint16_t focCycleCount
Definition: fcl_foc_cpu.h:686
float32_t offset_currentCs
Definition: fcl_cpu_cla_dm.h:333
float32_t ctrlPosRef
Definition: fcl_foc_cpu.h:591
static void FCL_runComplexCtrl(MOTOR_Vars_t *pMotor)
Definition: fcl_foc_cpu.h:951
DCL_PI dcl_spd
Definition: fcl_foc_cpu.h:662
FCL_cmplxCtrl_t cmplx_Iq
Definition: fcl_foc_cpu.h:672
float32_t tSamp
Definition: fcl_foc_cpu.h:80
CtrlState_e ctrlStateCom
Definition: fcl_foc_cpu.h:714
float32_t speedRefStart
Definition: fcl_foc_cpu.h:576
float32_t ctrlSpdOut
Definition: fcl_foc_cpu.h:623
uint32_t adcBaseW
Definition: fcl_foc_cpu.h:633
float32_t Ibase
Definition: fcl_foc_cpu.h:86
float32_t currentScale
Definition: fcl_cpu_cla_dm.h:339
#define EQEP_POSCNT_INIT_NOTHING
No action.
Definition: fcl_foc_cpu_dm.h:751
static void EQEP_resetPoscnt(uint32_t base, uint16_t initMode)
Definition: fcl_foc_cpu_dm.h:755
volatile uint32_t curC_PPBRESULT
Definition: fcl_cpu_cla_dm.h:354
float32_t ctrlIdRef
Definition: fcl_foc_cpu.h:588
float32_t Vbase
Definition: fcl_foc_cpu.h:85
float32_t tSamp
Definition: fcl_cpu_cla.h:83
float32_t Ibase
Definition: fcl_cpu_cla.h:89
float32_t cmidsqrt3
Definition: fcl_cpu_cla.h:81
float32_t carryOver
Definition: fcl_cmplx_ctrl.h:28
volatile uint32_t * pwmCompB
Definition: fcl_cpu_cla_dm.h:349
uint32_t pwmBaseW
Definition: fcl_foc_cpu.h:632
uint16_t voltageThreshHi
Definition: fcl_foc_cpu.h:704
float32_t posElecTheta
Definition: fcl_cpu_cla_dm.h:345
uint16_t enableSpeedLoop
Definition: fcl_foc_cpu.h:711
static void FCL_runPICtrlWrap(MOTOR_Vars_t *pMotor)
Definition: fcl_foc_cpu.h:889
float32_t err
Definition: fcl_cmplx_ctrl.h:26
struct _MOTOR_Vars_t_ MOTOR_Vars_t
typedefs for motorVars
float32_t Kerr
Definition: fcl_cmplx_ctrl.h:31
float32_t Wbase
Definition: fcl_cpu_cla.h:94
typedefs for motorVars
Definition: fcl_foc_cpu.h:74
FCL_Parameters_t FCL_params
Definition: fcl_cpu_cla_dm.h:370
Copyright 2023, Texas Instruments Incorporated