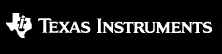 |
 |
Go to the documentation of this file.
84 #define DRV8353_ADDR_MASK (0x7800)
88 #define DRV8353_DATA_MASK (0x07FF)
92 #define DRV8353_RW_MASK (0x8000)
96 #define statReg00_addr 0x00
97 #define statReg01_addr 0x01
98 #define ctrlReg02_addr 0x02
99 #define ctrlReg03_addr 0x03
100 #define ctrlReg04_addr 0x04
101 #define ctrlReg05_addr 0x05
102 #define ctrlReg06_addr 0x06
103 #define ctrlReg07_addr 0x07
110 #define DRV8353_FAULT_TYPE_MASK (0x07FF)
112 #define DRV8353_STATUS00_VDS_LC_BITS (1 << 0)
113 #define DRV8353_STATUS00_VDS_HC_BITS (1 << 1)
114 #define DRV8353_STATUS00_VDS_LB_BITS (1 << 2)
115 #define DRV8353_STATUS00_VDS_HB_BITS (1 << 3)
116 #define DRV8353_STATUS00_VDS_LA_BITS (1 << 4)
117 #define DRV8353_STATUS00_VDS_HA_BITS (1 << 5)
121 #define DRV8353_STATUS00_OTSD_BITS (1 << 6)
122 #define DRV8353_STATUS00_UVLO_BITS (1 << 7)
123 #define DRV8353_STATUS00_GDF_BITS (1 << 8)
124 #define DRV8353_STATUS00_VDS_OCP_BITS (1 << 9)
125 #define DRV8353_STATUS00_FAULT_BITS (1 << 10)
132 #define DRV8353_STATUS01_VGS_LC_BITS (1 << 0)
136 #define DRV8353_STATUS01_VGS_HC_BITS (1 << 1)
140 #define DRV8353_STATUS01_VGS_LB_BITS (1 << 2)
144 #define DRV8353_STATUS01_VGS_HB_BITS (1 << 3)
148 #define DRV8353_STATUS01_VGS_LA_BITS (1 << 4)
152 #define DRV8353_STATUS01_VGS_HA_BITS (1 << 5)
156 #define DRV8353_STATUS01_CPUV_BITS (1 << 6)
160 #define DRV8353_STATUS01_OTW_BITS (1 << 7)
164 #define DRV8353_STATUS01_SC_OC_BITS (1 << 8)
168 #define DRV8353_STATUS01_SB_OC_BITS (1 << 9)
172 #define DRV8353_STATUS01_SA_OC_BITS (1 << 10)
179 #define DRV8353_CTRL02_CLR_FLT_BITS (1 << 0)
183 #define DRV8353_CTRL02_BRAKE_BITS (1 << 1)
187 #define DRV8353_CTRL02_COAST_BITS (1 << 2)
191 #define DRV8353_CTRL02_PWM1_DIR_BITS (1 << 3)
195 #define DRV8353_CTRL02_PWM1_COM_BITS (1 << 4)
199 #define DRV8353_CTRL02_PWM_MODE_BITS (3 << 5)
203 #define DRV8353_CTRL02_OTW_REP_BITS (1 << 7)
207 #define DRV8353_CTRL02_DIS_GDF_BITS (1 << 8)
211 #define DRV8353_CTRL02_DIS_CPUV_BITS (1 << 9)
215 #define DRV8353_CTRL02_RESERVED1_BITS (1 << 10)
222 #define DRV8353_CTRL03_IDRIVEN_HS_BITS (15 << 0)
226 #define DRV8353_CTRL03_IDRIVEP_HS_BITS (15 << 4)
230 #define DRV8353_CTRL03_LOCK_BITS (7 << 8)
237 #define DRV8353_CTRL04_IDRIVEN_LS_BITS (15 << 0)
241 #define DRV8353_CTRL04_IDRIVEP_LS_BITS (15 << 4)
245 #define DRV8353_CTRL04_TDRIVE_BITS (3 << 8)
249 #define DRV8353_CTRL04_CBC_BITS (1 << 10)
256 #define DRV8353_CTRL05_VDS_LVL_BITS (15 << 0)
260 #define DRV8353_CTRL05_OCP_DEG_BITS (3 << 4)
264 #define DRV8353_CTRL05_OCP_MODE_BITS (3 << 6)
268 #define DRV8353_CTRL05_DEAD_TIME_BITS (3 << 8)
272 #define DRV8353_CTRL05_TRETRY_BITS (1 << 10)
279 #define DRV8353_CTRL06_SEN_LVL_BITS (3 << 0)
283 #define DRV8353_CTRL06_CSA_CAL_C_BITS (1 << 2)
287 #define DRV8353_CTRL06_CSA_CAL_B_BITS (1 << 3)
291 #define DRV8353_CTRL06_CSA_CAL_A_BITS (1 << 4)
295 #define DRV8353_CTRL06_DIS_SEN_BITS (1 << 5)
299 #define DRV8353_CTRL06_CSA_GAIN_BITS (3 << 6)
303 #define DRV8353_CTRL06_LS_REF_BITS (1 << 8)
307 #define DRV8353_CTRL06_VREF_DIV_BITS (1 << 9)
311 #define DRV8353_CTRL06_CSA_FET_BITS (1 << 10)
319 #define DRV8353_CTRL07_CAL_MODE_BITS (1 << 0)
861 const uint16_t data);
893 #endif // end of DRV8353_H definition
DRV8353_CTRL06_SENLevel_e SEN_LVL
Definition: drv8353s.h:695
VDS_LEVEL = 0.940V.
Definition: drv8353s.h:515
IDRIVEN_LS = 0.240A.
Definition: drv8353s.h:487
DRV8353_CTRL03_Lock_e LOCK
Definition: drv8353s.h:649
bool VDS_OCP
Definition: drv8353s.h:588
bool CLR_FLT
Definition: drv8353s.h:625
uint16_t manReadAddr
Definition: drv8353s.h:744
GAIN_CSA = 40V/V.
Definition: drv8353s.h:572
Control Register 7.
Definition: drv8353s.h:343
Control Register 6.
Definition: drv8353s.h:342
VGS gate drive fault on B low-side MOSFET.
Definition: drv8353s.h:369
void DRV8353_enable(DRV8353_Handle handle)
Enables the DRV8353.
OCP_MODE = Automatic Retry.
Definition: drv8353s.h:539
charge pump undervoltage fault
Definition: drv8353s.h:373
overtemperature warning
Definition: drv8353s.h:374
OCP_DEG = 8us.
Definition: drv8353s.h:531
PWM_MODE = 6 inputs.
Definition: drv8353s.h:384
IDRIVEN_HS = 0.280A.
Definition: drv8353s.h:422
uint16_t all
Definition: drv8353s.h:655
IDRIVEN_HS = 0.240A.
Definition: drv8353s.h:421
IDRIVEP_LS = 0.030A.
Definition: drv8353s.h:461
bool TRETRY
Definition: drv8353s.h:682
Overtemperature shutdown.
Definition: drv8353s.h:356
VDS_LEVEL = 0.530V.
Definition: drv8353s.h:511
uint16_t rsvd
Definition: drv8353s.h:683
uint16_t rsvd2
Definition: drv8353s.h:635
DRV8353_Address_e
Enumeration for the register addresses.
Definition: drv8353s.h:334
DRV8353_CTRL03_PeakSinkCurHS_e IDRIVEN_HS
Definition: drv8353s.h:647
static void DRV8353_resetRxTimeout(DRV8353_Handle handle)
Resets the RX fifo timeout flag.
Definition: drv8353s.h:834
bool VDS_LA
Definition: drv8353s.h:583
bool CAL_MODE
Definition: drv8353s.h:716
struct DRV8353_STAT00_BITS bit
Definition: drv8353s.h:596
IDRIVEP_HS = 1.000A.
Definition: drv8353s.h:409
TDRIVE = 500ns.
Definition: drv8353s.h:449
IDRIVEP_LS = 0.680A.
Definition: drv8353s.h:473
Status Register 1.
Definition: drv8353s.h:337
IDRIVEN_HS = 0.060A.
Definition: drv8353s.h:418
DRV8353_CTRL05_DeadTime_e DEAD_TIME
Definition: drv8353s.h:681
uint16_t rsvd
Definition: drv8353s.h:650
DRV8353_CTRL06_CSAGain_e
Enumeration for the gain of shunt amplifier.
Definition: drv8353s.h:567
Object for the DRV8353 CTRL06 register.
Definition: drv8353s.h:694
VDS_LEVEL = 1.130V.
Definition: drv8353s.h:516
bool COAST
Definition: drv8353s.h:627
VDS_LEVEL = 0.310V.
Definition: drv8353s.h:509
bool manWriteCmd
Definition: drv8353s.h:747
IDRIVEP_LS = 1.000A.
Definition: drv8353s.h:475
IDRIVEP_LS = 0.170A.
Definition: drv8353s.h:466
IDRIVEP_LS = 0.260A.
Definition: drv8353s.h:468
bool CSA_CAL_A
Definition: drv8353s.h:698
IDRIVEN_LS = 0.880A.
Definition: drv8353s.h:494
DRV8353_CTRL04_PeakSinkCurLS_e
Enumeration for the low side gate drive peak sink current; adapt current ratings.
Definition: drv8353s.h:481
DRV8353_CTRL04_PeakSourCurLS_e
Enumeration for the low side gate drive peak source current; adapt current ratings.
Definition: drv8353s.h:458
IDRIVEN_LS = 1.140A.
Definition: drv8353s.h:495
IDRIVEP_LS = 0.080A.
Definition: drv8353s.h:463
union DRV8353_CTRL05_REG ctrlReg05
Definition: drv8353s.h:737
Object for the DRV8353 registers and commands.
Definition: drv8353s.h:729
Object for the DRV8353 CTRL03 register.
Definition: drv8353s.h:646
SEN_LVL = 0.75V.
Definition: drv8353s.h:560
DRV8353_CtrlMode_e
Enumeration for the R/W modes.
Definition: drv8353s.h:326
VDS overcurrent fault on C high-side MOSFET.
Definition: drv8353s.h:351
void DRV8353_readData(DRV8353_Handle handle, DRV8353_VARS_t *drv8353Vars)
Read from the DRV8353 SPI registers.
bool SC_OC
Definition: drv8353s.h:610
IDRIVEN_LS = 0.340A.
Definition: drv8353s.h:489
IDRIVEP_HS = 0.570A.
Definition: drv8353s.h:406
IDRIVEP_HS = 0.080A.
Definition: drv8353s.h:397
bool OCP_ACT
Definition: drv8353s.h:634
IDRIVEN_LS = 0.660A.
Definition: drv8353s.h:492
VDS overcurrent fault on B high-side MOSFET.
Definition: drv8353s.h:353
IDRIVEP_HS = 0.190A.
Definition: drv8353s.h:401
struct DRV8353_CTRL03_BITS bit
Definition: drv8353s.h:656
VDS overcurrent fault on A high-side MOSFET.
Definition: drv8353s.h:355
DRV8353_CTRL05_OcpMode_e OCP_MODE
Definition: drv8353s.h:680
DRV8353_CTRL05_VDSLVL_e
Enumeration for the VDS comparator threshold.
Definition: drv8353s.h:503
bool PWM1_COM
Definition: drv8353s.h:629
IDRIVEN_LS = 0.280A.
Definition: drv8353s.h:488
uint16_t all
Definition: drv8353s.h:709
DRV8353_CTRL03_PeakSourCurHS_e
Enumeration for the high side gate drive peak source current; gate currents not consistent with DS.
Definition: drv8353s.h:392
IDRIVEN_LS = 0.740A.
Definition: drv8353s.h:493
VDS_LEVEL = 0.130V.
Definition: drv8353s.h:506
struct DRV8353_STAT01_BITS bit
Definition: drv8353s.h:619
bool VREF_DIV
Definition: drv8353s.h:702
union DRV8353_CTRL03_REG ctrlReg03
Definition: drv8353s.h:735
uint32_t gpioNumber_CS
GPIO connected to the DRV8353 CS pin.
Definition: drv8353s.h:760
uint16_t rsvd1
Definition: drv8353s.h:717
bool UVLO
Definition: drv8353s.h:586
VGS gate drive fault on A high-side MOSFET.
Definition: drv8353s.h:372
Object for the DRV8353 STATUS00 register.
Definition: drv8353s.h:578
IDRIVEN_HS = 0.520A.
Definition: drv8353s.h:425
IDRIVEP_HS = 0.170A.
Definition: drv8353s.h:400
overcurrent on phase A
Definition: drv8353s.h:377
static void DRV8353_resetEnableTimeout(DRV8353_Handle handle)
Resets the enable timeout flag.
Definition: drv8353s.h:823
union DRV8353_STAT00_REG statReg00
Definition: drv8353s.h:731
bool PWM1_DIR
Definition: drv8353s.h:628
IDRIVEN_HS = 0.160A.
Definition: drv8353s.h:420
bool CBC
Definition: drv8353s.h:665
IDRIVEN_HS = 0.660A.
Definition: drv8353s.h:426
struct DRV8353_CTRL02_BITS bit
Definition: drv8353s.h:641
Object for the DRV8353 CTRL05 register.
Definition: drv8353s.h:677
IDRIVEP_HS = 0.030A.
Definition: drv8353s.h:395
VDS overcurrent fault on B low-side MOSFET.
Definition: drv8353s.h:352
DRV8353_CTRL02_PWMMode_e
Enumeration for the driver PWM mode.
Definition: drv8353s.h:382
bool VDS_LC
Definition: drv8353s.h:579
bool SB_OC
Definition: drv8353s.h:611
VGS gate drive fault on C high-side MOSFET.
Definition: drv8353s.h:368
struct DRV8353_CTRL07_BITS bit
Definition: drv8353s.h:724
IDRIVEN_HS = 1.360A.
Definition: drv8353s.h:430
IDRIVEP_HS = 0.140A.
Definition: drv8353s.h:399
DRV8353_CTRL03_PeakSourCurHS_e IDRIVEP_HS
Definition: drv8353s.h:648
uint16_t rsvd
Definition: drv8353s.h:666
IDRIVEP_HS = 0.060A.
Definition: drv8353s.h:396
IDRIVEP_LS = 0.190A.
Definition: drv8353s.h:467
overcurrent on phase B
Definition: drv8353s.h:376
IDRIVEN_HS = 0.120A.
Definition: drv8353s.h:419
union DRV8353_STAT01_REG statReg01
Definition: drv8353s.h:732
DRV8353_CTRL04_PeakSourCurLS_e IDRIVEP_LS
Definition: drv8353s.h:663
VDS_LEVEL = 1.300V.
Definition: drv8353s.h:517
GAIN_CSA = 20V/V.
Definition: drv8353s.h:571
uint16_t all
Definition: drv8353s.h:723
DRV8353_STATUS01_OvVdsFaults_e
Enumeration for the Status 1 register, OV/VDS faults.
Definition: drv8353s.h:365
union DRV8353_CTRL06_REG ctrlReg06
Definition: drv8353s.h:738
IDRIVEN_LS = 1.640A.
Definition: drv8353s.h:497
struct _DRV8353_VARS_t_ * DRV8353VARS_Handle
Defines the DRV8353_VARS_t handle.
Definition: drv8353s.h:753
void DRV8353_setGPIOCSNumber(DRV8353_Handle handle, uint32_t gpioNumber)
Sets the GPIO number in the DRV8353.
SEN_LVL = 0.25V.
Definition: drv8353s.h:558
bool VGS_HA
Definition: drv8353s.h:607
TDRIVE = 2000ns.
Definition: drv8353s.h:451
IDRIVEP_HS = 0.680A.
Definition: drv8353s.h:407
Read Mode.
Definition: drv8353s.h:329
uint16_t manReadData
Definition: drv8353s.h:746
OCP_MODE = Disabled.
Definition: drv8353s.h:541
DRV8353_CTRL06_CSAGain_e CSA_GAIN
Definition: drv8353s.h:700
VDS overcurrent fault on A low-side MOSFET.
Definition: drv8353s.h:354
IDRIVEP_HS = 0.820A.
Definition: drv8353s.h:408
IDRIVEN_LS = 1.360A.
Definition: drv8353s.h:496
uint16_t all
Definition: drv8353s.h:595
Defines the DRV8353 object.
Definition: drv8353s.h:757
DRV8353_CTRL05_VDSLVL_e VDS_LVL
Definition: drv8353s.h:678
uint16_t all
Definition: drv8353s.h:640
bool readCmd
Definition: drv8353s.h:742
VDS overcurrent fault on C low-side MOSFET.
Definition: drv8353s.h:350
IDRIVEP_LS = 0.570A.
Definition: drv8353s.h:472
Control Register 4.
Definition: drv8353s.h:340
VDS_LEVEL = 0.750V.
Definition: drv8353s.h:514
IDRIVEN_LS = 0.020A.
Definition: drv8353s.h:483
bool VGS_HB
Definition: drv8353s.h:605
IDRIVEP_HS = 0.120A.
Definition: drv8353s.h:398
bool CSA_FET
Definition: drv8353s.h:703
bool VDS_HB
Definition: drv8353s.h:582
bool VGS_LA
Definition: drv8353s.h:606
IDRIVEP_LS = 0.440A.
Definition: drv8353s.h:471
bool rxTimeOut
timeout flag for the RX FIFO
Definition: drv8353s.h:762
bool GDF
Definition: drv8353s.h:587
OCP_MODE = Latched fault.
Definition: drv8353s.h:538
DEAD_TIME = 200ns.
Definition: drv8353s.h:550
VDS_LEVEL = 1.500V.
Definition: drv8353s.h:518
Status Register 0.
Definition: drv8353s.h:336
bool SA_OC
Definition: drv8353s.h:612
IDRIVEP_HS = 0.010A.
Definition: drv8353s.h:394
Object for the DRV8353 CTRL06 register.
Definition: drv8353s.h:715
bool writeCmd
Definition: drv8353s.h:741
bool CSA_CAL_B
Definition: drv8353s.h:697
uint16_t all
Definition: drv8353s.h:618
uint16_t rsvd2
Definition: drv8353s.h:718
struct DRV8353_CTRL06_BITS bit
Definition: drv8353s.h:710
bool DIS_SEN
Definition: drv8353s.h:699
IDRIVEP_HS = 0.260A.
Definition: drv8353s.h:402
struct _DRV8353_VARS_t_ DRV8353_VARS_t
Object for the DRV8353 registers and commands.
VDS_LEVEL = 0.260V.
Definition: drv8353s.h:508
GAIN_CSA = 5V/V.
Definition: drv8353s.h:569
IDRIVEN_HS = 0.880A.
Definition: drv8353s.h:428
IDRIVEP_LS = 0.010A.
Definition: drv8353s.h:460
uint16_t rsvd2
Definition: drv8353s.h:590
struct DRV8353_CTRL04_BITS bit
Definition: drv8353s.h:672
bool VGS_LB
Definition: drv8353s.h:604
uint16_t DRV8353_readSPI(DRV8353_Handle handle, const DRV8353_Address_e regAddr)
Reads data from the DRV8353 register.
IDRIVEP_HS = 0.370A.
Definition: drv8353s.h:404
Unlock settings.
Definition: drv8353s.h:440
uint16_t all
Definition: drv8353s.h:688
IDRIVEN_LS = 0.120A.
Definition: drv8353s.h:485
void DRV8353_setGPIOENNumber(DRV8353_Handle handle, uint32_t gpioNumber)
Sets the GPIO number in the DRV8353.
IDRIVEN_HS = 2.000A.
Definition: drv8353s.h:432
bool BRAKE
Definition: drv8353s.h:626
bool DIS_GDF
Definition: drv8353s.h:632
#define DRV8353_DATA_MASK
Defines the data mask.
Definition: drv8353s.h:88
bool OTSD
Definition: drv8353s.h:585
bool VGS_LC
Definition: drv8353s.h:602
uint32_t gpioNumber_EN
GPIO connected to the DRV8353 enable pin.
Definition: drv8353s.h:761
DRV8353_CTRL05_OcpDeg_e
Enumeration for the OCP/VDS sense deglitch time; adapt deglitch time comments.
Definition: drv8353s.h:526
IDRIVEP_LS = 0.140A.
Definition: drv8353s.h:465
DRV8353_Handle DRV8353_init(void *pMemory)
Initializes the DRV8353 object.
IDRIVEP_HS = 0.440A.
Definition: drv8353s.h:405
uint16_t all
Definition: drv8353s.h:671
void DRV8353_writeSPI(DRV8353_Handle handle, const DRV8353_Address_e regAddr, const uint16_t data)
Writes data to the DRV8353 register.
bool CSA_CAL_C
Definition: drv8353s.h:696
static DRV_Word_t DRV8353_buildCtrlWord(const DRV8353_CtrlMode_e ctrlMode, const DRV8353_Address_e regAddr, const uint16_t data)
Builds the control word.
Definition: drv8353s.h:792
DRV8353_STATUS00_WarningWatchdog_e
Enumeration for the Status 0 register, faults.
Definition: drv8353s.h:348
DEAD_TIME = 400ns.
Definition: drv8353s.h:551
Definition: drv8353s.h:616
bool enableTimeOut
timeout flag for DRV8353 enable
Definition: drv8353s.h:763
bool VGS_HC
Definition: drv8353s.h:603
IDRIVEP_LS = 0.330A.
Definition: drv8353s.h:469
void DRV8353_writeData(DRV8353_Handle handle, DRV8353_VARS_t *drv8353Vars)
Write to the DRV8353 SPI registers.
IDRIVEP_LS = 0.820A.
Definition: drv8353s.h:474
Definition: drv8353s.h:593
IDRIVEP_LS = 0.120A.
Definition: drv8353s.h:464
IDRIVEN_HS = 0.380A.
Definition: drv8353s.h:424
uint16_t rsvd2
Definition: drv8353s.h:613
union DRV8353_CTRL02_REG ctrlReg02
Definition: drv8353s.h:734
Object for the DRV8353 STATUS01 register.
Definition: drv8353s.h:601
SEN_LVL = 1.00V.
Definition: drv8353s.h:561
bool VDS_HC
Definition: drv8353s.h:580
bool OTW
Definition: drv8353s.h:609
Control Register 3.
Definition: drv8353s.h:339
OCP_MODE = Report only.
Definition: drv8353s.h:540
Gate driver fault condition.
Definition: drv8353s.h:358
IDRIVEN_LS = 0.520A.
Definition: drv8353s.h:491
DRV8353_CTRL02_PWMMode_e PWM_MODE
Definition: drv8353s.h:630
uint16_t manWriteAddr
Definition: drv8353s.h:743
uint16_t DRV_Word_t
Defines the DRV8353 Word type.
Definition: drv8353s.h:772
uint16_t manWriteData
Definition: drv8353s.h:745
Undervoltage lockout fault condition.
Definition: drv8353s.h:357
IDRIVEN_HS = 1.640A.
Definition: drv8353s.h:431
struct DRV8353_CTRL05_BITS bit
Definition: drv8353s.h:689
IDRIVEP_HS = 0.330A.
Definition: drv8353s.h:403
Object for the DRV8353 CTRL02 register.
Definition: drv8353s.h:624
VDS monitor overcurrent fault condition.
Definition: drv8353s.h:359
PWM_MODE = 3 inputs.
Definition: drv8353s.h:385
bool OTW_REP
Definition: drv8353s.h:631
Lock settings.
Definition: drv8353s.h:441
DEAD_TIME = 100ns.
Definition: drv8353s.h:549
DEAD_TIME = 50ns.
Definition: drv8353s.h:548
DRV8353_CTRL05_OcpDeg_e OCP_DEG
Definition: drv8353s.h:679
VGS gate drive fault on A low-side MOSFET.
Definition: drv8353s.h:371
FAULT type, 0-Warning, 1-Latched.
Definition: drv8353s.h:360
TDRIVE = 4000ns.
Definition: drv8353s.h:452
VGS gate drive fault on B high-side MOSFET.
Definition: drv8353s.h:370
struct _DRV8353_Obj_ DRV8353_Obj
Defines the DRV8353 object.
TDRIVE = 1000ns.
Definition: drv8353s.h:450
IDRIVEN_LS = 2.000A.
Definition: drv8353s.h:498
bool VDS_LB
Definition: drv8353s.h:581
uint16_t rsvd
Definition: drv8353s.h:704
void DRV8353_setSPIHandle(DRV8353_Handle handle, uint32_t spiHandle)
Sets the SPI handle in the DRV8353.
IDRIVEN_LS = 0.060A.
Definition: drv8353s.h:484
VDS_LEVEL = 1.880V.
Definition: drv8353s.h:520
overcurrent on phase C
Definition: drv8353s.h:375
VDS_LEVEL = 0.680V.
Definition: drv8353s.h:513
bool manReadCmd
Definition: drv8353s.h:748
PWM_MODE = 1 input.
Definition: drv8353s.h:386
OCP_DEG = 2us.
Definition: drv8353s.h:528
DRV8353_CTRL04_PeakTime_e
Enumeration for the high side and low side gate drive peak source time; adapt timings to DRV8353.
Definition: drv8353s.h:447
DRV8353_CTRL03_Lock_e
Enumeration for the high side and low side gate drive peak source time; adapt timings to DRV8353.
Definition: drv8353s.h:438
DRV8353_CTRL04_PeakSinkCurLS_e IDRIVEN_LS
Definition: drv8353s.h:662
VDS_LEVEL = 0.200V.
Definition: drv8353s.h:507
OCP_DEG = 4us.
Definition: drv8353s.h:529
DRV8353_CTRL05_OcpMode_e
Enumeration for the OCP report mode.
Definition: drv8353s.h:536
IDRIVEN_HS = 0.340A.
Definition: drv8353s.h:423
DRV8353_CTRL06_SENLevel_e
Enumeration for the Sense OCP level.
Definition: drv8353s.h:556
Write Mode.
Definition: drv8353s.h:328
IDRIVEP_LS = 0.370A.
Definition: drv8353s.h:470
IDRIVEN_LS = 0.380A.
Definition: drv8353s.h:490
void DRV8353_setupSPI(DRV8353_Handle handle, DRV8353_VARS_t *drv8353Vars)
Initialize the interface to all 8320 SPI variables.
Control Register 5.
Definition: drv8353s.h:341
bool LS_REF
Definition: drv8353s.h:701
union DRV8353_CTRL07_REG ctrlReg07
Definition: drv8353s.h:739
IDRIVEN_HS = 0.740A.
Definition: drv8353s.h:427
DRV8353_CTRL05_DeadTime_e
Enumeration for the driver dead time.
Definition: drv8353s.h:546
struct _DRV8353_Obj_ * DRV8353_Handle
Defines the DRV8353 handle.
Definition: drv8353s.h:768
IDRIVEP_LS = 0.060A.
Definition: drv8353s.h:462
bool FAULT
Definition: drv8353s.h:589
bool CPUV
Definition: drv8353s.h:608
Control Register 2.
Definition: drv8353s.h:338
DRV8353_CTRL03_PeakSinkCurHS_e
Enumeration for the high side gate drive peak sink current; gate currents not consistent with DS.
Definition: drv8353s.h:415
GAIN_CSA = 10V/V.
Definition: drv8353s.h:570
Object for the DRV8353 CTRL04 register.
Definition: drv8353s.h:661
IDRIVEN_LS = 0.160A.
Definition: drv8353s.h:486
VDS_LEVEL = 0.450V.
Definition: drv8353s.h:510
Definition: drv8353s.h:638
SEN_LVL = 0.50V.
Definition: drv8353s.h:559
Definition: drv8353s.h:707
Definition: drv8353s.h:653
OCP_DEG = 6us.
Definition: drv8353s.h:530
VDS_LEVEL = 1.700V.
Definition: drv8353s.h:519
IDRIVEN_HS = 1.140A.
Definition: drv8353s.h:429
Definition: drv8353s.h:686
VDS_LEVEL = 0.060V.
Definition: drv8353s.h:505
Definition: drv8353s.h:721
Definition: drv8353s.h:669
bool DIS_GPUV
Definition: drv8353s.h:633
DRV8353_CTRL04_PeakTime_e TDRIVE
Definition: drv8353s.h:664
uint32_t spiHandle
handle for the serial peripheral interface
Definition: drv8353s.h:759
VDS_LEVEL = 0.600V.
Definition: drv8353s.h:512
bool VDS_HA
Definition: drv8353s.h:584
VGS gate drive fault on C low-side MOSFET.
Definition: drv8353s.h:367
union DRV8353_CTRL04_REG ctrlReg04
Definition: drv8353s.h:736
IDRIVEN_HS = 0.020A.
Definition: drv8353s.h:417
Copyright 2023, Texas Instruments Incorporated